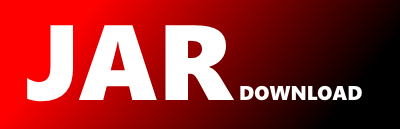
com.pdftools.signaturevalidation.TimeStampContent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
The newest version!
/****************************************************************************
*
* File: TimeStampContent.java
*
* Description: PDFTOOLS TimeStampContent Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.signaturevalidation;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The data and validation result of the cryptographic time-stamp
*/
public class TimeStampContent extends com.pdftools.signaturevalidation.SignatureContent
{
protected TimeStampContent(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static TimeStampContent createDynamicObject(long handle)
{
return new TimeStampContent(handle);
}
/**
* The time at which the signature has been validated (Getter)
*
*/
public OffsetDateTime getValidationTime()
{
OffsetDateTime retVal = getValidationTimeNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The source for the validation time (Getter)
*
*/
public EnumSet getValidationTimeSource()
{
int retVal = getValidationTimeSourceNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return getEnumSet(retVal, com.pdftools.signaturevalidation.TimeSource.class);
}
/**
* The hash algorithm used to calculate the signature's message digest (Getter)
*
*/
public com.pdftools.crypto.HashAlgorithm getHashAlgorithm()
{
int retVal = getHashAlgorithmNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return com.pdftools.crypto.HashAlgorithm.fromValue(retVal);
}
/**
* The time-stamp time (Getter)
*
*/
public OffsetDateTime getDate()
{
OffsetDateTime retVal = getDateNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The signing certificate (Getter)
*
*/
public com.pdftools.signaturevalidation.Certificate getSigningCertificate()
{
long retHandle = getSigningCertificateNative(getHandle());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
return null;
}
return com.pdftools.signaturevalidation.Certificate.createDynamicObject(retHandle);
}
/**
* The certificate chain of the signing certificate (Getter)
*
*/
public com.pdftools.signaturevalidation.CertificateChain getCertificateChain()
{
long retHandle = getCertificateChainNative(getHandle());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
return null;
}
return com.pdftools.signaturevalidation.CertificateChain.createDynamicObject(retHandle);
}
private native OffsetDateTime getValidationTimeNative(long handle);
private native int getValidationTimeSourceNative(long handle);
private native int getHashAlgorithmNative(long handle);
private native OffsetDateTime getDateNative(long handle);
private native long getSigningCertificateNative(long handle);
private native long getCertificateChainNative(long handle);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy