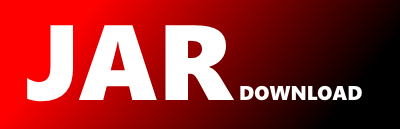
com.pdftools.signaturevalidation.Validator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
/****************************************************************************
*
* File: Validator.java
*
* Description: PDFTOOLS Validator Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.signaturevalidation;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.*;
/**
* The class to check the validity of signatures
*/
public class Validator extends NativeObject
{
protected Validator(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static Validator createDynamicObject(long handle)
{
return new Validator(handle);
}
/***
* Listener interface for the {@link Constraint} event.
*
*/
public interface ConstraintListener extends EventListener
{
void constraint(Constraint event);
}
/**
*
* Report the result of a constraint validation of {@link Validator#validate }.
*/
public class Constraint extends EventObject
{
private static final long serialVersionUID = 1061L;
private String message;
private com.pdftools.signaturevalidation.Indication indication;
private com.pdftools.signaturevalidation.SubIndication subIndication;
private com.pdftools.pdf.SignedSignatureField signature;
private String dataPart;
private Constraint(Object source, String message, com.pdftools.signaturevalidation.Indication indication, com.pdftools.signaturevalidation.SubIndication subIndication, com.pdftools.pdf.SignedSignatureField signature, String dataPart) {
super(source);
this.message = message;
this.indication = indication;
this.subIndication = subIndication;
this.signature = signature;
this.dataPart = dataPart;
}
/**
* The validation message
*/
public String getMessage() {
return message;
}
/**
* The main indication
*/
public com.pdftools.signaturevalidation.Indication getIndication() {
return indication;
}
/**
* The sub indication
*/
public com.pdftools.signaturevalidation.SubIndication getSubIndication() {
return subIndication;
}
/**
* The signature field
*/
public com.pdftools.pdf.SignedSignatureField getSignature() {
return signature;
}
/**
*
* The data part is {@code null} for constraints of the main signature and a path for constraints related to elements of the signature.
*
* Examples:
*
* -
* {@code certificate:"Some Certificate"}: When validating a certificate "Some Certificate" of the main signature.
* -
* {@code time-stamp":Some TSA Responder"/certificate:"Intermediate TSA Responder Certificate"}: When validating a certificate "Intermediate TSA Responder Certificate" of the time-stamp embedded into the main signature.
*
*/
public String getDataPart() {
return dataPart;
}
}
private Hashtable constraintDic = new Hashtable();
private class ConstraintNativeClass
{
private ConstraintListener listener;
private long context;
public ConstraintNativeClass(ConstraintListener listener)
{
this.listener = listener;
}
public void constraintHandler(String message, com.pdftools.signaturevalidation.Indication indication, com.pdftools.signaturevalidation.SubIndication subIndication, com.pdftools.pdf.SignedSignatureField signature, String dataPart)
{
Constraint event = new Constraint(this, message, indication, subIndication, signature, dataPart);
try {
this.listener.constraint(event);
}
catch (Exception ex) { }
}
}
/**
* Add a listener for the {@link Constraint} event.
* @param listener Listener for the {@link Constraint} event.
* If a listener is added that is already registered, it is ignored.
*/
public void addConstraintListener(ConstraintListener listener)
{
if(!constraintDic.containsKey(listener))
{
ConstraintNativeClass eventNativeClass = new ConstraintNativeClass(listener);
long context = addConstraintHandlerNative(getHandle(), eventNativeClass);
if (context == 0)
throwLastRuntimeException();
eventNativeClass.context = context;
constraintDic.put(listener, eventNativeClass);
}
}
/**
* Remove registered listener for the {@link Constraint} event.
* @param listener Listener for the {@link Constraint} event that should be removed.
* If the listener is not registered, it is ignored.
*/
public void removeConstraintListener(ConstraintListener listener)
{
if(constraintDic.containsKey(listener))
{
if (!removeConstraintHandlerNative(getHandle(), constraintDic.get(listener).context))
{
if (getLastErrorCode() != 5)
throwLastRuntimeException();
}
constraintDic.remove(listener);
}
}
/**
*
*/
public Validator()
{
this(newHelper());
}
private static long newHelper()
{
long handle = newNative();
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return handle;
}
/**
* Validate the signatures of a PDF document
* @param document
* The document to check the signatures of
* @param profile
* The validation profile
* @param selector
* The signatures to validate
* @return
* The result of the validation
*
* @throws com.pdftools.LicenseException The license check has failed.
* @throws com.pdftools.ProcessingException The processing has failed.
* @throws IllegalArgumentException if {@code document} is {@code null}
* @throws IllegalArgumentException if {@code profile} is {@code null}
* @throws IllegalArgumentException if {@code selector} is {@code null}
*/
public com.pdftools.signaturevalidation.ValidationResults validate(com.pdftools.pdf.Document document, com.pdftools.signaturevalidation.profiles.Profile profile, com.pdftools.signaturevalidation.SignatureSelector selector)
throws
com.pdftools.LicenseException,
com.pdftools.ProcessingException
{
if (document == null)
throw new IllegalArgumentException("Argument 'document' must not be null.", new NullPointerException("'document'"));
if (profile == null)
throw new IllegalArgumentException("Argument 'profile' must not be null.", new NullPointerException("'profile'"));
if (selector == null)
throw new IllegalArgumentException("Argument 'selector' must not be null.", new NullPointerException("'selector'"));
long retHandle = validateNative(getHandle(), getHandle(document), document, getHandle(profile), profile, selector.getValue());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 12: throw new com.pdftools.LicenseException(getLastErrorMessage());
case 21: throw new com.pdftools.ProcessingException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return com.pdftools.signaturevalidation.ValidationResults.createDynamicObject(retHandle);
}
private native long addConstraintHandlerNative(long handle, ConstraintNativeClass eventClass);
private native boolean removeConstraintHandlerNative(long handle, long context);
private static native long newNative();
private native long validateNative(long handle, long document, com.pdftools.pdf.Document documentObj, long profile, com.pdftools.signaturevalidation.profiles.Profile profileObj, int selector);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy