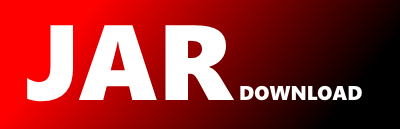
com.pdftools.sys.FileStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
package com.pdftools.sys;
/**
* The stream implementation to read from and write to files.
*
* This implementation is backed by {@code java.io.RandomAccessFile}.
*/
public class FileStream implements Stream
{
/**
* The file access mode.
*/
public static enum Mode {
/**
* Open for reading only.
* Invoking any of the write methods of the resulting object will cause an IOException to be thrown.
*/
READ_ONLY("r"),
/**
* Open for reading and writing. The existing file content will be deleted.
* If the file does not already exist then an attempt will be made to create it.
*/
READ_WRITE_NEW("rw"),
/**
* Open for reading and writing. The newly added content will be appended to the file.
* If the file does not already exist then an attempt will be made to create it.
*/
READ_WRITE_APPEND("rw"),
/**
* Open for reading and writing. Require that every update to the file's content or metadata be written synchronously to the underlying storage device.
*/
READ_WRITE_S("rws"),
/**
* Open for reading and writing. Require that every update to the file's content be written synchronously to the underlying storage device.
*/
READ_WRITE_D("rwd");
private String mode;
private Mode(String mode) {
this.mode = mode;
}
@Override
public String toString()
{
return mode;
}
}
/**
* Create a new file stream from a file.
* This constructor works similarly to the constructor of the underlying {@code java.io.RandomAccessFile}.
*
* @param file The file to be opened
* @param mode The open mode of the file
* @see java.io.RandomAccessFile#RandomAccessFile(java.io.File, String)
* @throws java.io.IOException
*/
public FileStream(java.io.File file, Mode mode) throws java.io.IOException
{
raFile = new java.io.RandomAccessFile(file, mode.toString());
if (mode == Mode.READ_WRITE_NEW)
{
raFile.setLength(0);
}
}
/**
* Create a new file stream from a file name.
* This constructor works similarly to the constructor of the underlying {@code java.io.RandomAccessFile}.
*
* @param filename The name of the file to be opened
* @param mode The open mode of the file
* @see java.io.RandomAccessFile#RandomAccessFile(java.io.File, String)
* @throws java.io.IOException
*/
public FileStream(String filename, Mode mode) throws java.io.IOException
{
this(new java.io.File(filename), mode);
}
/**
* Create a new file stream from a {@code RandomAccessFile}.
*
* ATTENTION: Opening a {@code RandomAccessFile} in "rw" mode does not delete the current content of the file,
* so be sure that you delete it yourself if needed (which is mostly the case).
*
* @param raFile The underlying {@code RandomAccessFile}
* @see java.io.RandomAccessFile
*/
public FileStream(java.io.RandomAccessFile raFile)
{ this.raFile = raFile; }
/**
* Get the underlying {@code RandomAccessFile}.
* @return The underlying {@code RandomAccessFile}
*/
public java.io.RandomAccessFile GetRandomAccessFile()
{ return raFile; }
/**
* Get the length of the stream in bytes.
* @return the length of the stream in bytes
*/
public long getLength() throws java.io.IOException
{ return raFile.length(); }
/**
* Set the length of the stream in bytes.
* @param length the length of the stream in bytes
*/
public void setLength(long length) throws java.io.IOException
{ raFile.setLength(length); }
/**
* Set byte position.
* @param position The new position of the stream (-1 for EOS)
* @return true if successful
*/
public boolean seek(long position) throws java.io.IOException
{ if (position >= 0)raFile.seek(position); else raFile.seek(raFile.length()); return true; }
/**
* Get current byte position.
* @return byte position, -1 if position unknown
*/
public long tell() throws java.io.IOException
{ return raFile.getFilePointer(); }
/**
* Read from the stream.
* @param buffer The buffer where the data is written
* @param offset The starting element in the buffer
* @param length The maximum number of bytes to be read
* @return The actual number of bytes read (-1 if EOS)
*/
public int read(byte[] buffer, int offset, int length) throws java.io.IOException
{ return raFile.read(buffer, offset, length); }
/**
* Write to the stream.
* @param buffer The buffer where the data lies
* @param offset The starting element in the buffer
* @param length The maximum number of bytes to be written
*/
public void write(byte[] buffer, int offset, int length) throws java.io.IOException
{ raFile.write(buffer, offset, length); }
/**
* Copy the content from another stream.
* @param stream The stream from which the content is copied
*/
public void copy(Stream stream) throws java.io.IOException
{
byte[] buffer = new byte[8192];
int read;
while ((read = stream.read(buffer, 0, 8192)) > 0)
write(buffer, 0, read);
}
/**
* Close the file stream.
* This closes also the underlying {@code RandomAccessFile}.
*/
public void close() throws java.io.IOException
{ raFile.close(); }
private java.io.RandomAccessFile raFile = null;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy