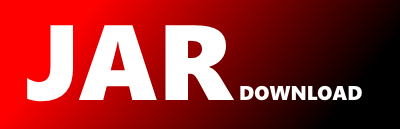
com.pekinsoft.api.WindowManager Maven / Gradle / Ivy
Show all versions of application-framework-api Show documentation
/*
* Copyright (C) 2024 PekinSOFT Systems
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
* *****************************************************************************
* Project : application-framework-api
* Class : WindowManager.java
* Author : Sean Carrick
* Created : Jul 14, 2024
* Modified : Jul 14, 2024
*
* Purpose: See class JavaDoc for explanation
*
* Revision History:
*
* WHEN BY REASON
* ------------ ------------------- -----------------------------------------
* Jul 14, 2024 Sean Carrick Initial creation.
* *****************************************************************************
*/
package com.pekinsoft.api;
import com.pekinsoft.framework.Application;
import com.pekinsoft.framework.BackgroundTask;
import com.pekinsoft.lookup.Lookup;
import java.awt.Container;
import javax.swing.*;
/**
* {@code WindowManager} is an interface that may be used for managing the
* windows of an application. It offers various {@code showMainFrame},
* {@code hide}, and {@code closeMainFrame} methods that each accept a different
* Swing type of window, including the {@link JInternalFrame} type.
*
* Furthermore, the {@code WindowManager} provides methods for negotiating
* {@link JToolBar toolbars} and {@link JMenuBar menu bars} for
* multiple-document interface (MDI) applications, between the inner
* windows and the main window.
*
* Another feature of the {@code WindowManger} is the ability to find a window
* based upon its name. The {@code name} parameter can be defined by the
* implementation to mean what makes the most sense, such as the window's
* {@code name} property, or even the window's {@code title} property. Some
* implementations may even search for both.
*
* @author Sean Carrick ([email protected])
*
* @version 1.0
* @since 1.0
*/
public interface WindowManager {
public static WindowManager getDefault() {
return Lookup.getDefault().lookup(WindowManager.class);
}
/**
* Shows the main window of the application.
*/
void showMainFrame();
/**
* Shows the specified {@link JComponent} in a manner that makes sense for
* the implementation.
*
* @param component the {@code JComponent} to be shown
*/
void show(JComponent component);
/**
* Shows the specified frame.
*
* @param frame the frame to be shown
*/
void show(JFrame frame);
/**
* Shows the specified dialog.
*
* @param dialog the dialog to be shown
*/
void show(JDialog dialog);
/**
* Closes the main window for the application, and allows it to perform any
* last minute cleanup tasks that it may have.
*/
void closeMainFrame();
/**
* Closes the {@link JComponent} in a manner that makes sense for the
* implementation.
*
* @param component the {@code JComponent} to be closed
*/
void close(JComponent component);
/**
* Closes the specified frame, which disposes of it.
*
* @param frame the frame to be closed
*/
void close(JFrame frame);
/**
* Closes the specified dialog, which disposes of it.
*
* @param dialog the dialog to be closed
*/
void close(JDialog dialog);
/**
* Locates a window based upon the value of its name property.
*
* @param name the name of the window to find
*
* @return the window (as a {@link Container}, or {@code null} if none is
* found
*/
Container find(String name);
/**
* Retrieves the main window for the application.
*
* @return the application's main window
*/
JFrame getMainFrame();
/**
* Adds an JToolBar to the main window's toolbars.
*
* @param toolBar the toolbar to be added
*/
void addToolBar(JToolBar toolBar);
/**
* Removes an JToolBar from the main window's toolbars.
*
* @param toolBar the toolbar to be removed
*/
void removeToolBar(JToolBar toolBar);
/**
* Shows the {@link javax.swing.JToolBar toolbar} based on its name.
*
* @param name the name of the toolbar to showMainFrame
*/
void showToolBar(String name);
/**
* Hides the {@link javax.swing.JToolBar toolbar} based on its name.
*
* @param name the name of the toolbar to hide
*/
void hideToolBar(String name);
/**
* Shows/Hides the text on the buttons of the
* {@link javax.swing.JToolBar toolbar}.
*
* @param showText {@code true} will showMainFrame the button text,
* {@code false} will hide it
*/
void showButtonText(boolean showText);
/**
* Provides a means of doing any work that needs to be done prior to the
* {@link #getMainFrame() main frame} being displayed to the user. This
* could include anything from connecting to network resources to loading
* windows that were opened the last time the main frame was closed.
*
* The work performed in this method should be kicked off onto a background
* thread by being performed in a {@link BackgroundTask}.
*/
void preload();
}