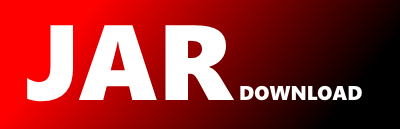
com.pekinsoft.desktop.error.ErrorSupport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of application-framework-api Show documentation
Show all versions of application-framework-api Show documentation
A simple platform on which Java/Swing desktop applications may be built.
This updated version has packaged the entire library into a single JAR
file. We have also made the following changes:
ToolBarGenerator should now create ButtonGroups properly for state actions.
ApplicationContext has accessors for the WindowManager, DockingManager,
StatusDisplayer, and ProgressHandler implementations. It defaults to
testing the Application's MainView and the MainView's StatusBar, then
uses the Lookup, if the MainView and its StatusBar do not implement the
desired interfaces.
StatusMessage now uses the com.pekinsoft.desktop.error.ErrorLevel instead
of the java.util.logging.Level, so that the levels will no longer need to
be cast in order to be used.
The newest version!
/*
* $Id: ErrorSupport.java 3840 2010-10-09 03:25:17Z kschaefe $
*
* Copyright 2006 Sun Microsystems, Inc., 4150 Network Circle,
* Santa Clara, California 95054, U.S.A. All rights reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
*/
package com.pekinsoft.desktop.error;
import java.util.ArrayList;
import java.util.List;
import javax.swing.SwingUtilities;
/**
* ErrorSupport provides support for managing error listeners.
* @author Joshua Marinacci [email protected]
* @see ErrorListener
* @see ErrorEvent
*/
public class ErrorSupport {
private List listeners;
private Object source;
/**
* Creates a new instance of {@code ErrorSupport}
* @param source The object which will fire the {@code ErrorEvent}s
*/
public ErrorSupport(Object source) {
this.source = source;
listeners = new ArrayList();
}
/**
* Add an ErrorListener
* @param listener the listener to add
*/
public void addErrorListener(ErrorListener listener) {
listeners.add(listener);
}
/**
* Remove an error listener
* @param listener the listener to remove
*/
public void removeErrorListener(ErrorListener listener) {
listeners.remove(listener);
}
/**
* Returns an array of all the listeners which were added to the
* {@code ErrorSupport} object with {@code addErrorListener()}.
* @return all of the {@code ErrorListener}s added or an empty array if no listeners have been
* added.
*/
public ErrorListener[] getErrorListeners() {
return listeners.toArray(ErrorListener[]::new);
}
/**
* Report that an error has occurred
* @param throwable The {@code {@link Error}} or {@code {@link Exception}} which occured.
*/
public void fireErrorEvent(final Throwable throwable) {
final ErrorEvent evt = new ErrorEvent(throwable, source);
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
for(ErrorListener el : listeners) {
el.errorOccured(evt);
}
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy