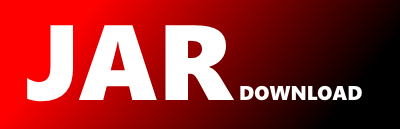
com.pekinsoft.lookup.ModuleHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of application-framework-api Show documentation
Show all versions of application-framework-api Show documentation
A simple platform on which Java/Swing desktop applications may be built.
This updated version has packaged the entire library into a single JAR
file. We have also made the following changes:
ToolBarGenerator should now create ButtonGroups properly for state actions.
ApplicationContext has accessors for the WindowManager, DockingManager,
StatusDisplayer, and ProgressHandler implementations. It defaults to
testing the Application's MainView and the MainView's StatusBar, then
uses the Lookup, if the MainView and its StatusBar do not implement the
desired interfaces.
StatusMessage now uses the com.pekinsoft.desktop.error.ErrorLevel instead
of the java.util.logging.Level, so that the levels will no longer need to
be cast in order to be used.
The newest version!
/*
* Copyright (C) 2023 PekinSOFT Systems
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
* *****************************************************************************
* Project : LookupAPI
* Class : ModuleHelper.java
* Author : Sean Carrick
* Created : Jan 17, 2023
* Modified : Jan 17, 2023
*
* Purpose: See class JavaDoc for explanation
*
* Revision History:
*
* WHEN BY REASON
* ------------ ------------------- -----------------------------------------
* Jan 17, 2023 Sean Carrick Initial creation.
* *****************************************************************************
*/
package com.pekinsoft.lookup;
import java.lang.module.ModuleDescriptor;
import java.lang.module.ModuleFinder;
import java.lang.module.ModuleReference;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Set;
/**
* `ModuleHelper` simply allows for us to remove *most* of the error handling out
* of the `Lookup`s.
*
* @author Sean Carrick <sean at pekinsoft dot com>
*
* @version 1.0
* @since 1.0
*/
class ModuleHelper {
private final ModuleFinder finder;
private final ClassLoader loader;
private final Class type;
ModuleHelper (ModuleFinder finder, ClassLoader loader, Class type) {
this.finder = finder;
this.loader = loader;
this.type = type;
}
Class> find() {
Class> cls = null;
final Set refs = finder.findAll();
for (ModuleReference r : refs) {
ModuleDescriptor d = r.descriptor();
for (ModuleDescriptor.Provides p : d.provides()) {
if (p.service().equals(type.getName())) {
String provider = p.providers().get(0); // The first one.
try {
cls = loader.loadClass(provider);
break;
} catch (ClassNotFoundException cnfe) {
// TODO: Handle the exception.
}
}
}
if (cls != null) {
break;
}
}
return cls;
}
boolean isSingleton(Class> cls) {
try {
Method getInstance = cls.getMethod("getInstance");
if (getInstance != null) {
return true;
}
} catch (NoSuchMethodException
| SecurityException e) {
return false;
}
return false;
}
boolean isProvider(Class> cls) {
try {
Method provider = cls.getMethod("provider");
if (provider != null) {
return true;
}
} catch (NoSuchMethodException
| SecurityException e) {
return false;
}
return false;
}
Object getInstance(Class> cls) {
try {
Method getInstance = cls.getMethod("getInstance");
if (getInstance != null) {
if (!getInstance.canAccess(null)) {
getInstance.setAccessible(true);
}
return getInstance.invoke(cls);
}
} catch (IllegalAccessException
| IllegalArgumentException
| NoSuchMethodException
| SecurityException
| InvocationTargetException e) {
return null;
}
return null;
}
Object getProvider(Class> cls) {
try {
Method provider = cls.getMethod("provider");
if (provider != null) {
if (!provider.canAccess(null)) {
provider.setAccessible(true);
}
return provider.invoke(cls);
}
} catch (IllegalAccessException
| IllegalArgumentException
| NoSuchMethodException
| SecurityException
| InvocationTargetException e) {
return null;
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy