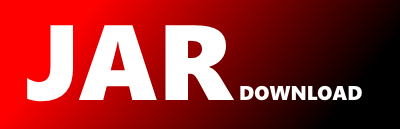
com.pekinsoft.utils.ScreenUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of application-framework-api Show documentation
Show all versions of application-framework-api Show documentation
A simple platform on which Java/Swing desktop applications may be built.
This updated version has packaged the entire library into a single JAR
file. We have also made the following changes:
ToolBarGenerator should now create ButtonGroups properly for state actions.
ApplicationContext has accessors for the WindowManager, DockingManager,
StatusDisplayer, and ProgressHandler implementations. It defaults to
testing the Application's MainView and the MainView's StatusBar, then
uses the Lookup, if the MainView and its StatusBar do not implement the
desired interfaces.
StatusMessage now uses the com.pekinsoft.desktop.error.ErrorLevel instead
of the java.util.logging.Level, so that the levels will no longer need to
be cast in order to be used.
The newest version!
/*
* Copyright (C) 2022 PekinSOFT Systems
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*
* *****************************************************************************
* Project : AppUtils
* Class : ScreenUtils.java
* Author : Jiří Kovalský
* Created : Mar 08, 2020
* Modified : Apr 01, 2022
*
* Purpose: See class JavaDoc comments.
*
* Revision History:
*
* WHEN BY REASON
* ------------ ------------------- ------------------------------------------
* Mar 08, 2020 Jiří Kovalský Initial creation.
* Nov 13, 2020 Jiří Kovalský Contributed the getCenterPoint method for
* centering windows on their parent.
* Feb 13, 2021 Sean Carrick Introduced {@code null} container for centering a
* window on the screen to getCenterPoint.
* Apr 01, 2022 Sean Carrick Added the getCenterPoint(Container,
* Container) method to allow for centering a
* child window or dialog on the screen that
* the parent window is showing. This is used
* for proper window placement in a multi-
* display environment.
*
* I also added the getCurrentScreenNumber()
* method to allow a program to determine on
* which screen number the given window is
* located. The only parameter is the window's
* getLocationOnScreen() Point.
* *****************************************************************************
*/
package com.pekinsoft.utils;
import java.awt.*;
/**
* This class provides static utility methods that concentrate on using the
* screen (or desktop) effectively. Currently, there are three `public static`
* methods available:
*
* * `getCenterPoint(Dimension, Dimension)` which centers the child `Dimension`
* within the constraints of the parent `Dimension`. This method also allows for
* the child `Dimension` to be centered on the screen (without taking into
* account *multi-screen* environments) if the parent supplied is {@code null}.
* * `getCenterPoint(Container, Container, boolean)` which centers the child
* `Container` on screen or on the parent `Container` if the {@code boolean}
* value is {@code true}. This method differs from the
* `getCenterPoint(Dimension, Dimension)` method in that this method takes into
* consideration a multi-screen environment. * `getCurrentScreenNumber(Point)`
* which returns the screen number for the screen upon which the window whose
* `java.awt.Point` is provided. The `Point` needs to be the windows
* `getLocationOnScreen()` return value, from which the current screen number is
* able to be determined. This method works for multi-screen environments that
* are set up horizontally or vertically, as well as if there are more than just
* two screens.
*
* With these methods, applications will be able to use the desktop and the
* various available screens efficiently.
*
* - Class Information
* - This class was originally started by Jiří Kovalský, one of the two
* founding partners of GS United Labs. The increases in functionality have been
* provided by the other founding partner, Sean Carrick. Even so, much credit
* goes to Jiří for getting this class started, as Sean never even thought of
* creating a method to center a window at all. He just kept reinventing the
* wheel whenever he needed to center a window within a program.
*
* @author Jiří Kovalský <jiri dot kovalsky at centrum dot cz>
*
* @since 1.0
* @version 1.7
*/
public class ScreenUtils {
/**
* Calculates central position of the window within its container. If the
* specified `Window` is a top-AppLevel window or dialog, {@code null} can
* be specified for the provided `container` to center the window or dialog
* on the screen.
*
*
* - Contributed By
* - Jiří Kovalský <jiri dot kovalsky at centrum dot cz>
* - Updated Feb 13, 2021
* - Update allows the specified `container` to be set to {@code null} to
* allow centering a top-AppLevel window or dialog on the screen.
* Sean Carrick <PekinSOFT at outlook dot com>
*
*
* @param container Dimensions of parent container where window will be
* located. If {@code null} is supplied, then the `Window`
* will be centered on the screen.
* @param window Dimensions of child window which will be displayed
* within its parent container.
*
* @return Location of top left corner of window to be displayed in the
* center of its parent container.
*/
public static Point getCenterPoint(Dimension container, Dimension window) {
if (container != null) {
int x = container.width / 2;
int y = container.height / 2;
x = x - (window.width / 2);
y = y - (window.height / 2);
x = x < 0 ? 0 : x;
y = y < 0 ? 0 : y;
return new Point(x, y);
} else {
GraphicsDevice device = GraphicsEnvironment.
getLocalGraphicsEnvironment()
.getDefaultScreenDevice();
GraphicsConfiguration conf = device.getDefaultConfiguration();
Rectangle screenBounds = conf.getBounds();
int x = screenBounds.width / 2;
int y = screenBounds.height / 2;
x = x - (window.width / 2);
y = y - (window.height / 2);
x = x < 0 ? 0 : x;
y = y < 0 ? 0 : y;
return new Point(x, y);
}
}
/**
* Calculates central position of the window within its container.
*
* If the specified `child` is a top-AppLevel window or dialog, {@code null}
* can be specified for the provided {@code parent} to center the window or
* dialog on the screen.
*
* This version of `getCenterPoint` requires that `java.awt.Container`s be
* provided so that the `java.awt.GraphicsConfiguration` can be taken into
* account for systems that are using multiple virtual screens (i.e., using
* multiple monitors). By taking this into account, the `child` container
* will be displayed on the same virtual screen as the {@code parent}
* container.
*
* - Contributed By
* - Sean Carrick <PekinSOFT at outlook dot com>
* - Contributed on April 01, 2022
* - I had used `getCenterPoint(Dimension, Dimension)` to center an
* Options dialog due to the `SessionStorage` not working for an unknown
* reason (TBD later). The Options dialog did indeed center on the screen,
* but on the extension monitor on my laptop, when the primary program's
* main window was on the laptop screen. I felt this should not happen, so
* created this version of `getCenterPoint(Container, Container)` in order
* to not have this happen. It just seemed to be unprofessional for an
* application to place its windows and dialogs willy-nilly, without paying
* attention to which screen the main window is on when displaying child
* windows.
*
*
* In essence, this method gets the `java.awt.GraphicsDevice` on which the
* {@code parent} window is displayed, and then centers the `child` window
* on that graphics device.
*
* @param parent the parent (top-AppLevel) window or dialog
* @param child the child (secondary) window or dialog
* @param centerOnParent {@code true} to center the `child` over the
* {@code parent}; {@code false} to center the `child`
* on the screen
*
* @return the `java.awt.Point` at which to place the `child` to have it
* properly centered
*
* @throws IllegalArgumentException if {@code parent} or `child` is
* {@code null}
*/
public static Point getCenterPoint(Container parent, Container child,
boolean centerOnParent) {
/*
* if (parent == null) { throw new IllegalArgumentException("{@code
* null} parent"); }
*/
if (child == null) {
throw new IllegalArgumentException("{@code null} child");
}
if (centerOnParent) {
return centerOnParent(parent, child);
} else {
return centerOnParentsScreen(parent, child);
}
}
/**
* Determines on which screen number in a multi-screen environment the
* current window is located. The only required parameter is the current
* window's `getLocationOnScreen()` `java.awt.Point` value. Using this
* `Point`, this method will determine upon which screen the window is
* located, regardless of whether the multi-screen setup is horizontal (left
* and right), vertical (top and bottom), or if there are more than just two
* screens.
*
* @param locationOnScreen the `java.util.Point` at which the window is
* located
*
* @return the current screen number (zero-based)
*
* @throws IllegalArgumentException if `locationOnScreen` is {@code null}
*/
public static int getCurrentScreenNumber(Point locationOnScreen) {
if (locationOnScreen == null) {
throw new IllegalArgumentException("{@code null} locationOnScreen");
} else if (locationOnScreen.x < Toolkit.getDefaultToolkit().
getScreenSize().width
&& locationOnScreen.y < Toolkit.getDefaultToolkit().
getScreenSize().height) {
return 0;
}
GraphicsEnvironment ge = GraphicsEnvironment.
getLocalGraphicsEnvironment();
GraphicsDevice[] devices = ge.getScreenDevices();
Rectangle virtualBounds = new Rectangle();
for (GraphicsDevice gd : devices) {
GraphicsConfiguration[] gc = gd.getConfigurations();
for (GraphicsConfiguration gc1 : gc) {
virtualBounds = virtualBounds.union(gc1.getBounds());
}
}
int screenWidth = virtualBounds.width / devices.length;
int screenHeight = virtualBounds.height / devices.length;
int screenNumber = 0;
for (int x = 0; x < devices.length; x++) {
if (locationOnScreen.x > (screenWidth)) {
screenWidth *= x + 1;
screenNumber = x;
} else if (locationOnScreen.y > screenHeight) {
screenHeight *= x + 1;
screenNumber = x;
}
}
return screenNumber;
}
private static Point centerOnParent(Container parent, Container child) {
Rectangle virtualBounds = new Rectangle();
GraphicsEnvironment ge = GraphicsEnvironment.
getLocalGraphicsEnvironment();
GraphicsDevice[] devices = ge.getScreenDevices();
DisplayMode mode = GraphicsEnvironment.getLocalGraphicsEnvironment()
.getDefaultScreenDevice().getDisplayMode();
GraphicsDevice defaultScreen = GraphicsEnvironment
.getLocalGraphicsEnvironment().getDefaultScreenDevice();
boolean screen0IsDefault = GraphicsEnvironment
.getLocalGraphicsEnvironment().getScreenDevices()[0]
.equals(defaultScreen);
boolean isVertical = mode.getWidth() < mode.getHeight();
for (GraphicsDevice gd : devices) {
GraphicsConfiguration[] gc = gd.getConfigurations();
for (GraphicsConfiguration gc1 : gc) {
virtualBounds = virtualBounds.union(gc1.getBounds());
}
}
int locX = ((parent == null)
? (mode.getWidth() - child.getWidth()) / 2
: (parent.getWidth() - child.getWidth()) / 2);
locX += (parent != null)
? parent.getLocationOnScreen().x
: screen0IsDefault
? 0
: ((virtualBounds.width - child.getWidth()) / 2) + child.
getWidth();
int locY = ((parent == null)
? (mode.getHeight() - child.getHeight()) / 2
: (parent.getHeight() - child.getHeight()) / 2);
locY += (parent != null)
? parent.getLocationOnScreen().x
: screen0IsDefault
? 0
: ((virtualBounds.height - child.getHeight()) / 2);
Point position = new Point(locX, locY);
return position;
}
private static Point centerOnParentsScreen(Container parent, Container child) {
Rectangle virtualBounds = new Rectangle();
GraphicsEnvironment ge = GraphicsEnvironment.
getLocalGraphicsEnvironment();
GraphicsDevice[] devices = ge.getScreenDevices();
Dimension screenSize = Toolkit.getDefaultToolkit().getScreenSize();
for (GraphicsDevice gd : devices) {
GraphicsConfiguration[] gc = gd.getConfigurations();
for (GraphicsConfiguration gc1 : gc) {
virtualBounds = virtualBounds.union(gc1.getBounds());
}
}
boolean right = parent.getLocationOnScreen().x > screenSize.width;
boolean bottom = parent.getLocationOnScreen().y > screenSize.height;
int shiftX = screenSize.width;
int shiftY = screenSize.height;
int locX = (screenSize.width - child.getWidth()) / 2;
int locY = (screenSize.height - child.getHeight()) / 2;
if (right) {
locX += shiftX;
}
if (bottom) {
locY += shiftY;
}
Point position = new Point(locX, locY);
return position;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy