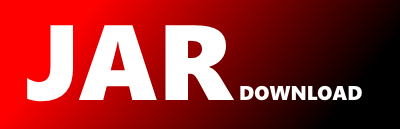
com.pekinsoft.wizard.api.displayer.NavProgress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of application-framework-api Show documentation
Show all versions of application-framework-api Show documentation
A simple platform on which Java/Swing desktop applications may be built.
This updated version has packaged the entire library into a single JAR
file. We have also made the following changes:
ToolBarGenerator should now create ButtonGroups properly for state actions.
ApplicationContext has accessors for the WindowManager, DockingManager,
StatusDisplayer, and ProgressHandler implementations. It defaults to
testing the Application's MainView and the MainView's StatusBar, then
uses the Lookup, if the MainView and its StatusBar do not implement the
desired interfaces.
StatusMessage now uses the com.pekinsoft.desktop.error.ErrorLevel instead
of the java.util.logging.Level, so that the levels will no longer need to
be cast in order to be used.
The newest version!
package com.pekinsoft.wizard.api.displayer;
import com.pekinsoft.api.Exceptions;
import com.pekinsoft.framework.Application;
import com.pekinsoft.lookup.Lookup;
import com.pekinsoft.wizard.spi.ResultProgressHandle;
import com.pekinsoft.wizard.spi.Summary;
import java.awt.Container;
import java.awt.EventQueue;
import java.lang.System.Logger;
import java.lang.System.Logger.Level;
import java.lang.reflect.InvocationTargetException;
import java.net.URL;
import javax.swing.Icon;
import javax.swing.ImageIcon;
import javax.swing.JLabel;
import javax.swing.JProgressBar;
import javax.swing.border.EmptyBorder;
/**
* Show progress bar for deferred results, with a label showing percent done and
* progress bar.
*
*
* ***This class is NOT AN API CLASS. There is no
* commitment that it will remain backward compatible or even exist in the
* future. The API of this library is in the packages
* `com.pekinsoft.wizard.api` and
* `com.pekinsoft.wizard.spi`***.
*
* @author [email protected]
*/
public class NavProgress implements ResultProgressHandle {
private static final Logger logger = System.getLogger(NavProgress.class.getName());
Exceptions exceptions = Lookup.getDefault().lookup(Exceptions.class);
JProgressBar progressBar = new JProgressBar();
JLabel lbl = new JLabel();
JLabel busy = new JLabel();
WizardDisplayerImpl parent;
String failMessage = null;
boolean isUseBusy = false;
Container ipanel = null;
boolean isInitialized = false;
/**
* isRunning is true until finished or failed is called
*/
boolean isRunning = true;
NavProgress(WizardDisplayerImpl impl, boolean useBusy) {
this.parent = impl;
isUseBusy = useBusy;
}
@Override
public void addProgressComponents(Container panel) {
panel.add(lbl);
if (isUseBusy) {
ensureBusyInitialized();
panel.add(busy);
} else {
panel.add(progressBar);
}
isInitialized = true;
ipanel = panel;
}
@Override
public void setProgress(final String description, final int currentStep, final int totalSteps) {
Runnable r = () -> {
lbl.setText(description == null ? " " : description); // NOI18N
setProgress(currentStep, totalSteps);
};
invoke(r);
}
@Override
public void setProgress(final int currentStep, final int totalSteps) {
Runnable r = () -> {
if (totalSteps == -1) {
progressBar.setIndeterminate(true);
} else {
if (currentStep > totalSteps || currentStep < 0) {
if (currentStep == -1 && totalSteps == -1) {
return;
}
throw new IllegalArgumentException("Bad step values: " // NOI18N
+ currentStep + " out of " + totalSteps); // NOI18N
}
progressBar.setIndeterminate(false);
progressBar.setMaximum(totalSteps);
progressBar.setValue(currentStep);
}
setUseBusy(false);
};
invoke(r);
}
@Override
public void setBusy(final String description) {
Runnable r = () -> {
lbl.setText(description == null ? " " : description); // NOI18N
progressBar.setIndeterminate(true);
setUseBusy(true);
};
invoke(r);
}
protected void setUseBusy(boolean useBusy) {
if (isInitialized) {
if (useBusy && (!isUseBusy)) {
ipanel.remove(progressBar);
ensureBusyInitialized();
ipanel.add(busy);
ipanel.invalidate();
} else if (!useBusy && isUseBusy) {
ipanel.remove(busy);
ipanel.add(progressBar);
ipanel.invalidate();
}
}
isUseBusy = useBusy;
}
private void ensureBusyInitialized() {
if (busy.getIcon() == null) {
URL url = getClass().getResource("busy.gif");
Icon icon = new ImageIcon(url);
busy.setIcon(icon);
}
}
private void invoke(Runnable r) {
if (EventQueue.isDispatchThread()) {
r.run();
} else {
try {
EventQueue.invokeAndWait(r);
} catch (InvocationTargetException ex) {
exceptions.print(ex);
logger.log(Level.ERROR, "Error invoking operation {0}: {1}", ex,
ex.getClass().getName(), ex.getMessage());
} catch (InterruptedException ex) {
logger.log(Level.ERROR, "Error invoking operation {0}: {1}", ex,
ex.getClass().getName(), ex.getMessage());
exceptions.print(ex);
}
}
}
@Override
public void finished(final Object o) {
isRunning = false;
Runnable r = () -> {
if (o instanceof Summary) {
Summary summary = (Summary) o;
parent.handleSummary(summary);
parent.setWizardResult(summary.getResult());
} else if (parent.getDeferredResult() != null) {
parent.setWizardResult(o);
// handle result based on which button was pushed
parent.getButtonManager().deferredResultFinished(o);
}
};
invoke(r);
}
@Override
public void failed(final String message, final boolean canGoBack) {
failMessage = message;
isRunning = false;
Runnable r = () -> {
// cheap word wrap
JLabel comp = new JLabel("" + message); // NOI18N
comp.setBorder(new EmptyBorder(5, 5, 5, 5));
parent.setCurrentWizardPanel(comp);
parent.getTtlLabel().setText(
Application
.getInstance()
.getContext()
.getResourceMap(getClass())
.getString("Failed")); // NOI18N
NavButtonManager bm = parent.getButtonManager();
bm.deferredResultFailed(canGoBack);
};
invoke(r);
}
@Override
public boolean isRunning() {
return isRunning;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy