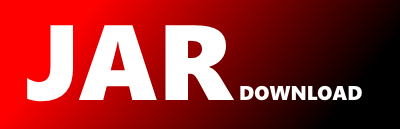
com.pekinsoft.wizard.spi.WizardPanelNavResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of application-framework-api Show documentation
Show all versions of application-framework-api Show documentation
A simple platform on which Java/Swing desktop applications may be built.
This updated version has packaged the entire library into a single JAR
file. We have also made the following changes:
ToolBarGenerator should now create ButtonGroups properly for state actions.
ApplicationContext has accessors for the WindowManager, DockingManager,
StatusDisplayer, and ProgressHandler implementations. It defaults to
testing the Application's MainView and the MainView's StatusBar, then
uses the Lookup, if the MainView and its StatusBar do not implement the
desired interfaces.
StatusMessage now uses the com.pekinsoft.desktop.error.ErrorLevel instead
of the java.util.logging.Level, so that the levels will no longer need to
be cast in order to be used.
The newest version!
/* The contents of this file are subject to the terms of the Common Development
and Distribution License (the License). You may not use this file except in
compliance with the License.
You can obtain a copy of the License at http://www.netbeans.org/cddl.html
or http://www.netbeans.org/cddl.txt.
When distributing Covered Code, include this CDDL Header Notice in each file
and include the License file at http://www.netbeans.org/cddl.txt.
*/
package com.pekinsoft.wizard.spi;
import java.util.Map;
/**
* Result class for the methods in WizardPanel.
*
* For immediate action, one of the two constantants PROCEED or REMAIN_ON_PAGE
* should be returned. Otherwise an instance of a subclass should be returned
* that computes a Boolean result.
*
* @author [email protected]
*/
public abstract class WizardPanelNavResult extends DeferredWizardResult
{
/**
* value for procced to next step in the wizard.
*/
public static final WizardPanelNavResult PROCEED = new WPNRimmediate(true);
/**
* Value to remain on the current page in the wizard
*/
public static final WizardPanelNavResult REMAIN_ON_PAGE = new WPNRimmediate(false);
public WizardPanelNavResult(boolean useBusy) {
super (false, useBusy);
}
public WizardPanelNavResult(boolean useBusy, boolean canAbort) {
super (canAbort, useBusy);
}
public WizardPanelNavResult() {
super (false, false);
}
public boolean isDeferredComputation()
{
return true;
}
/*
* internal class for the constants only
*/
private final static class WPNRimmediate extends WizardPanelNavResult
{
boolean value;
WPNRimmediate (boolean v)
{
value = v;
}
@Override
public boolean isDeferredComputation()
{
return false;
}
@Override
public boolean equals (Object o)
{
return o instanceof WPNRimmediate && ((WPNRimmediate)o).value == value;
}
@Override
public int hashCode()
{
return value ? 1 : 2;
}
@Override
public void start(Map settings, ResultProgressHandle progress)
{
// Should never get here, this is supposed to be immediate!
throw new RuntimeException("Immediate result was called as deferral!");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy