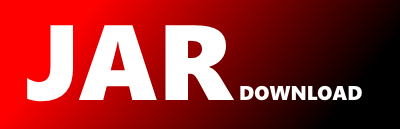
com.penalara.ghc.jsonghcfile.engineghcfile.AssignedTeachingSession Maven / Gradle / Ivy
Show all versions of engineGHCFile Show documentation
package com.penalara.ghc.jsonghcfile.engineghcfile;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* AssignedTeachingSession
*
* Class unit or on call hour assigned in the timetable.
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"refSession",
"refClassRomms",
"refTeacher"
})
public class AssignedTeachingSession {
/**
* Identifier of the teaching session assigned.
*
*/
@JsonProperty("refSession")
@JsonPropertyDescription("Identifier of the teaching session assigned.")
private Integer refSession;
/**
* RefclassRomms
*
* List of classrooms assigned to teach the class unit.
* (Required)
*
*/
@JsonProperty("refClassRomms")
@JsonPropertyDescription("List of classrooms assigned to teach the class unit.")
private List refClassRomms = new ArrayList();
/**
* RefMainTeacher
*
* Identifier of the MAIN teacher of the session in the input file.
*
*/
@JsonProperty("refTeacher")
@JsonPropertyDescription("Identifier of the MAIN teacher of the session in the input file.")
private String refTeacher;
/**
* Identifier of the teaching session assigned.
*
*/
@JsonProperty("refSession")
public Integer getRefSession() {
return refSession;
}
/**
* Identifier of the teaching session assigned.
*
*/
@JsonProperty("refSession")
public void setRefSession(Integer refSession) {
this.refSession = refSession;
}
/**
* RefclassRomms
*
* List of classrooms assigned to teach the class unit.
* (Required)
*
*/
@JsonProperty("refClassRomms")
public List getRefClassRomms() {
return refClassRomms;
}
/**
* RefclassRomms
*
* List of classrooms assigned to teach the class unit.
* (Required)
*
*/
@JsonProperty("refClassRomms")
public void setRefClassRomms(List refClassRomms) {
this.refClassRomms = refClassRomms;
}
/**
* RefMainTeacher
*
* Identifier of the MAIN teacher of the session in the input file.
*
*/
@JsonProperty("refTeacher")
public String getRefTeacher() {
return refTeacher;
}
/**
* RefMainTeacher
*
* Identifier of the MAIN teacher of the session in the input file.
*
*/
@JsonProperty("refTeacher")
public void setRefTeacher(String refTeacher) {
this.refTeacher = refTeacher;
}
}