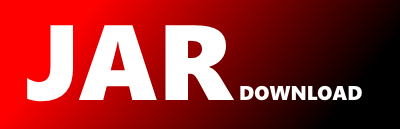
com.penalara.ghc.jsonghcfile.engineghcfile.Assignment Maven / Gradle / Ivy
Show all versions of engineGHCFile Show documentation
package com.penalara.ghc.jsonghcfile.engineghcfile;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* Assignment
*
* Object with list of each type of session can be assigned in the section (teaching session, meetings, complementary activities, on call services).
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"teachingSessions",
"meetings",
"complementaryActivities",
"onCallServices"
})
public class Assignment {
/**
* TeachingSessions
*
* List of teaching sessions assigned in the section.
*
*/
@JsonProperty("teachingSessions")
@JsonPropertyDescription("List of teaching sessions assigned in the section.")
private List teachingSessions = new ArrayList();
/**
* AssignedMeetings
*
* List of meetings assigned in the section.
*
*/
@JsonProperty("meetings")
@JsonPropertyDescription("List of meetings assigned in the section.")
private List meetings = new ArrayList();
/**
* ComplementaryActivitiesAssigned
*
* List of meetings assigned in the section.
*
*/
@JsonProperty("complementaryActivities")
@JsonPropertyDescription("List of meetings assigned in the section.")
private List complementaryActivities = new ArrayList();
/**
* OnCallServices
*
* List of teaching on call hours assigned in the section.
*
*/
@JsonProperty("onCallServices")
@JsonPropertyDescription("List of teaching on call hours assigned in the section.")
private List onCallServices = new ArrayList();
@JsonIgnore
private Map additionalProperties = new LinkedHashMap();
/**
* TeachingSessions
*
* List of teaching sessions assigned in the section.
*
*/
@JsonProperty("teachingSessions")
public List getTeachingSessions() {
return teachingSessions;
}
/**
* TeachingSessions
*
* List of teaching sessions assigned in the section.
*
*/
@JsonProperty("teachingSessions")
public void setTeachingSessions(List teachingSessions) {
this.teachingSessions = teachingSessions;
}
/**
* AssignedMeetings
*
* List of meetings assigned in the section.
*
*/
@JsonProperty("meetings")
public List getMeetings() {
return meetings;
}
/**
* AssignedMeetings
*
* List of meetings assigned in the section.
*
*/
@JsonProperty("meetings")
public void setMeetings(List meetings) {
this.meetings = meetings;
}
/**
* ComplementaryActivitiesAssigned
*
* List of meetings assigned in the section.
*
*/
@JsonProperty("complementaryActivities")
public List getComplementaryActivities() {
return complementaryActivities;
}
/**
* ComplementaryActivitiesAssigned
*
* List of meetings assigned in the section.
*
*/
@JsonProperty("complementaryActivities")
public void setComplementaryActivities(List complementaryActivities) {
this.complementaryActivities = complementaryActivities;
}
/**
* OnCallServices
*
* List of teaching on call hours assigned in the section.
*
*/
@JsonProperty("onCallServices")
public List getOnCallServices() {
return onCallServices;
}
/**
* OnCallServices
*
* List of teaching on call hours assigned in the section.
*
*/
@JsonProperty("onCallServices")
public void setOnCallServices(List onCallServices) {
this.onCallServices = onCallServices;
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
}