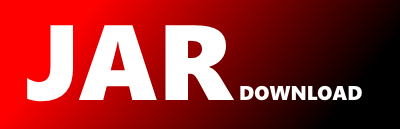
com.penalara.ghc.jsonghcfile.engineghcfile.EngineInputGHCSchema Maven / Gradle / Ivy
Show all versions of engineGHCFile Show documentation
package com.penalara.ghc.jsonghcfile.engineghcfile;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* EngineInputGHCSchema
*
* Schema of the object that contains the timetable planning data.
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"periods",
"frames",
"buildings",
"classRooms",
"classRoomsSets",
"tasks",
"teachers",
"subjects",
"groups",
"sessions",
"meetings",
"complementaryActivities",
"onCallServices",
"optimizationWeights",
"engineSettings"
})
public class EngineInputGHCSchema {
/**
* Periods
*
* Weeks or periods with different assignments which contains the timetable.If there are no defined periods, the engine will create a single period with all the days of the timetable.
*
*/
@JsonProperty("periods")
@JsonPropertyDescription("Weeks or periods with different assignments which contains the timetable.")
private Periods periods;
/**
* Frames
*
* Tables of the timetable that contain sections where the class units are located.
*
*/
@JsonProperty("frames")
@JsonPropertyDescription("Tables of the timetable that contain sections where the class units are located.")
private List frames = new ArrayList();
/**
* Buildings
*
* It contains the school or educational institution buildings.
*
*/
@JsonProperty("buildings")
@JsonPropertyDescription("It contains the school or educational institution buildings.")
private List buildings = new ArrayList();
/**
* ClassRooms
*
* It contains the classrooms to organize the class units.
*
*/
@JsonProperty("classRooms")
@JsonPropertyDescription("It contains\u00a0the\u00a0classrooms\u00a0to organize\u00a0the\u00a0class units.")
private List classRooms = new ArrayList();
/**
* ClassRoomsSets
*
* A division of the classrooms, grouped by organizational characteristics or needs.
*
*/
@JsonProperty("classRoomsSets")
@JsonPropertyDescription("A division of the classrooms, grouped by organizational characteristics or needs.")
private List classRoomsSets = new ArrayList();
/**
* Tasks
*
* Types of tasks or activities for the teachers.
*
*/
@JsonProperty("tasks")
@JsonPropertyDescription("Types of tasks or activities for the teachers.")
private List tasks = new ArrayList();
/**
* Teachers
*
* Teachers list of the timetable.
*
*/
@JsonProperty("teachers")
@JsonPropertyDescription("Teachers list of the timetable.")
private List teachers = new ArrayList();
/**
* Subjects
*
* School subjects taught in the class units.
*
*/
@JsonProperty("subjects")
@JsonPropertyDescription("School subjects taught in the class units.")
private List subjects = new ArrayList();
/**
* Groups
*
* Sets of students.
*
*/
@JsonProperty("groups")
@JsonPropertyDescription("Sets of students.")
private List groups = new ArrayList();
/**
* Sessions
*
* Definition of the teacher class units. These may be with groups of students, meetings with other teachers or complementary activities.
*
*/
@JsonProperty("sessions")
@JsonPropertyDescription("Definition of the teacher class units. These may be with groups of students, meetings with other teachers or complementary activities.")
private List sessions = new ArrayList();
/**
* Meetings
*
* List of meetings between teachers defined in the timetable.
*
*/
@JsonProperty("meetings")
@JsonPropertyDescription("List of meetings between teachers defined in the timetable.")
private List meetings = new ArrayList();
/**
* ComplementaryActivities
*
* List of complementary activities of the teachers.
*
*/
@JsonProperty("complementaryActivities")
@JsonPropertyDescription("List of complementary activities of the teachers.")
private List complementaryActivities = new ArrayList();
/**
* OnCallServices
*
* List of on-call services of teachers defined in the timetable.
*
*/
@JsonProperty("onCallServices")
@JsonPropertyDescription("List of on-call services of teachers defined in the timetable.")
private List onCallServices = new ArrayList();
/**
* OptimizationWeights
*
* This indicates the weights that the weighting options will have for the engine optimisation process. The engine shall apply default values for elements that have not been specified.
*
*/
@JsonProperty("optimizationWeights")
@JsonPropertyDescription("This indicates the weights that the weighting options will have for the engine optimisation process. The engine shall apply default values for elements that have not been specified.")
private OptimizationWeights optimizationWeights;
/**
* EngineSettings
*
* Customised engine configuration parameters.
*
*/
@JsonProperty("engineSettings")
@JsonPropertyDescription("Customised engine configuration parameters.")
private EngineSettings engineSettings;
@JsonIgnore
private Map additionalProperties = new LinkedHashMap();
/**
* Periods
*
* Weeks or periods with different assignments which contains the timetable.If there are no defined periods, the engine will create a single period with all the days of the timetable.
*
*/
@JsonProperty("periods")
public Periods getPeriods() {
return periods;
}
/**
* Periods
*
* Weeks or periods with different assignments which contains the timetable.If there are no defined periods, the engine will create a single period with all the days of the timetable.
*
*/
@JsonProperty("periods")
public void setPeriods(Periods periods) {
this.periods = periods;
}
/**
* Frames
*
* Tables of the timetable that contain sections where the class units are located.
*
*/
@JsonProperty("frames")
public List getFrames() {
return frames;
}
/**
* Frames
*
* Tables of the timetable that contain sections where the class units are located.
*
*/
@JsonProperty("frames")
public void setFrames(List frames) {
this.frames = frames;
}
/**
* Buildings
*
* It contains the school or educational institution buildings.
*
*/
@JsonProperty("buildings")
public List getBuildings() {
return buildings;
}
/**
* Buildings
*
* It contains the school or educational institution buildings.
*
*/
@JsonProperty("buildings")
public void setBuildings(List buildings) {
this.buildings = buildings;
}
/**
* ClassRooms
*
* It contains the classrooms to organize the class units.
*
*/
@JsonProperty("classRooms")
public List getClassRooms() {
return classRooms;
}
/**
* ClassRooms
*
* It contains the classrooms to organize the class units.
*
*/
@JsonProperty("classRooms")
public void setClassRooms(List classRooms) {
this.classRooms = classRooms;
}
/**
* ClassRoomsSets
*
* A division of the classrooms, grouped by organizational characteristics or needs.
*
*/
@JsonProperty("classRoomsSets")
public List getClassRoomsSets() {
return classRoomsSets;
}
/**
* ClassRoomsSets
*
* A division of the classrooms, grouped by organizational characteristics or needs.
*
*/
@JsonProperty("classRoomsSets")
public void setClassRoomsSets(List classRoomsSets) {
this.classRoomsSets = classRoomsSets;
}
/**
* Tasks
*
* Types of tasks or activities for the teachers.
*
*/
@JsonProperty("tasks")
public List getTasks() {
return tasks;
}
/**
* Tasks
*
* Types of tasks or activities for the teachers.
*
*/
@JsonProperty("tasks")
public void setTasks(List tasks) {
this.tasks = tasks;
}
/**
* Teachers
*
* Teachers list of the timetable.
*
*/
@JsonProperty("teachers")
public List getTeachers() {
return teachers;
}
/**
* Teachers
*
* Teachers list of the timetable.
*
*/
@JsonProperty("teachers")
public void setTeachers(List teachers) {
this.teachers = teachers;
}
/**
* Subjects
*
* School subjects taught in the class units.
*
*/
@JsonProperty("subjects")
public List getSubjects() {
return subjects;
}
/**
* Subjects
*
* School subjects taught in the class units.
*
*/
@JsonProperty("subjects")
public void setSubjects(List subjects) {
this.subjects = subjects;
}
/**
* Groups
*
* Sets of students.
*
*/
@JsonProperty("groups")
public List getGroups() {
return groups;
}
/**
* Groups
*
* Sets of students.
*
*/
@JsonProperty("groups")
public void setGroups(List groups) {
this.groups = groups;
}
/**
* Sessions
*
* Definition of the teacher class units. These may be with groups of students, meetings with other teachers or complementary activities.
*
*/
@JsonProperty("sessions")
public List getSessions() {
return sessions;
}
/**
* Sessions
*
* Definition of the teacher class units. These may be with groups of students, meetings with other teachers or complementary activities.
*
*/
@JsonProperty("sessions")
public void setSessions(List sessions) {
this.sessions = sessions;
}
/**
* Meetings
*
* List of meetings between teachers defined in the timetable.
*
*/
@JsonProperty("meetings")
public List getMeetings() {
return meetings;
}
/**
* Meetings
*
* List of meetings between teachers defined in the timetable.
*
*/
@JsonProperty("meetings")
public void setMeetings(List meetings) {
this.meetings = meetings;
}
/**
* ComplementaryActivities
*
* List of complementary activities of the teachers.
*
*/
@JsonProperty("complementaryActivities")
public List getComplementaryActivities() {
return complementaryActivities;
}
/**
* ComplementaryActivities
*
* List of complementary activities of the teachers.
*
*/
@JsonProperty("complementaryActivities")
public void setComplementaryActivities(List complementaryActivities) {
this.complementaryActivities = complementaryActivities;
}
/**
* OnCallServices
*
* List of on-call services of teachers defined in the timetable.
*
*/
@JsonProperty("onCallServices")
public List getOnCallServices() {
return onCallServices;
}
/**
* OnCallServices
*
* List of on-call services of teachers defined in the timetable.
*
*/
@JsonProperty("onCallServices")
public void setOnCallServices(List onCallServices) {
this.onCallServices = onCallServices;
}
/**
* OptimizationWeights
*
* This indicates the weights that the weighting options will have for the engine optimisation process. The engine shall apply default values for elements that have not been specified.
*
*/
@JsonProperty("optimizationWeights")
public OptimizationWeights getOptimizationWeights() {
return optimizationWeights;
}
/**
* OptimizationWeights
*
* This indicates the weights that the weighting options will have for the engine optimisation process. The engine shall apply default values for elements that have not been specified.
*
*/
@JsonProperty("optimizationWeights")
public void setOptimizationWeights(OptimizationWeights optimizationWeights) {
this.optimizationWeights = optimizationWeights;
}
/**
* EngineSettings
*
* Customised engine configuration parameters.
*
*/
@JsonProperty("engineSettings")
public EngineSettings getEngineSettings() {
return engineSettings;
}
/**
* EngineSettings
*
* Customised engine configuration parameters.
*
*/
@JsonProperty("engineSettings")
public void setEngineSettings(EngineSettings engineSettings) {
this.engineSettings = engineSettings;
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
}