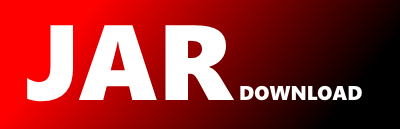
com.penalara.ghc.jsonghcfile.engineghcfile.GhcEngineSchema Maven / Gradle / Ivy
package com.penalara.ghc.jsonghcfile.engineghcfile;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* GhcEngineSchema
*
* Schema with the structure of GHC Engine files
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"id",
"meta",
"specifications",
"results"
})
public class GhcEngineSchema {
/**
* IdFile
*
* Indicates the id number of the file at the Penalara Server.
*
*/
@JsonProperty("id")
@JsonPropertyDescription("Indicates the id number of the file at the Penalara Server.")
private Integer id;
/**
* FileMetadata
*
* Information about the file.
* (Required)
*
*/
@JsonProperty("meta")
@JsonPropertyDescription("Information about the file.")
private FileMetadata meta;
/**
* EngineInputGHCSchema
*
* Schema of the object that contains the timetable planning data.
* (Required)
*
*/
@JsonProperty("specifications")
@JsonPropertyDescription("Schema of the object that contains the timetable planning data.")
private EngineInputGHCSchema specifications;
/**
* EngineResults
*
* Tables of the timetable that contain sections where the class units are located.
*
*/
@JsonProperty("results")
@JsonPropertyDescription("Tables of the timetable that contain sections where the class units are located.")
private List results = new ArrayList();
/**
* IdFile
*
* Indicates the id number of the file at the Penalara Server.
*
*/
@JsonProperty("id")
public Integer getId() {
return id;
}
/**
* IdFile
*
* Indicates the id number of the file at the Penalara Server.
*
*/
@JsonProperty("id")
public void setId(Integer id) {
this.id = id;
}
/**
* FileMetadata
*
* Information about the file.
* (Required)
*
*/
@JsonProperty("meta")
public FileMetadata getMeta() {
return meta;
}
/**
* FileMetadata
*
* Information about the file.
* (Required)
*
*/
@JsonProperty("meta")
public void setMeta(FileMetadata meta) {
this.meta = meta;
}
/**
* EngineInputGHCSchema
*
* Schema of the object that contains the timetable planning data.
* (Required)
*
*/
@JsonProperty("specifications")
public EngineInputGHCSchema getSpecifications() {
return specifications;
}
/**
* EngineInputGHCSchema
*
* Schema of the object that contains the timetable planning data.
* (Required)
*
*/
@JsonProperty("specifications")
public void setSpecifications(EngineInputGHCSchema specifications) {
this.specifications = specifications;
}
/**
* EngineResults
*
* Tables of the timetable that contain sections where the class units are located.
*
*/
@JsonProperty("results")
public List getResults() {
return results;
}
/**
* EngineResults
*
* Tables of the timetable that contain sections where the class units are located.
*
*/
@JsonProperty("results")
public void setResults(List results) {
this.results = results;
}
}