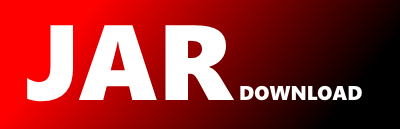
com.penalara.ghc.jsonghcfile.engineghcfile.StablePeriods Maven / Gradle / Ivy
Show all versions of engineGHCFile Show documentation
package com.penalara.ghc.jsonghcfile.engineghcfile;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* StablePeriods
*
* The allocation of the class unit of the session is the same in each period.Only one property must be specified.
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"stableTiming",
"variableTiming",
"stableTimingList"
})
public class StablePeriods {
/**
* StableTiming
*
* It contains the fixed way of allocating time across the days of the period.
*
*/
@JsonProperty("stableTiming")
@JsonPropertyDescription("It contains the fixed way of allocating time across the days of the period.")
private List stableTiming = new ArrayList();
/**
* VariableTiming
*
* It defines the allocating time as a total of time, maximum and minimun range of sections per day.
*
*/
@JsonProperty("variableTiming")
@JsonPropertyDescription("It defines the allocating time as a total of time, maximum and minimun range of sections per day.")
private VariableTiming variableTiming;
/**
* StableTimingList
*
* It contains an array of 'StableTiming' distributions.
*
*/
@JsonProperty("stableTimingList")
@JsonPropertyDescription("It contains an array of 'StableTiming' distributions.")
private List> stableTimingList = new ArrayList>();
/**
* StableTiming
*
* It contains the fixed way of allocating time across the days of the period.
*
*/
@JsonProperty("stableTiming")
public List getStableTiming() {
return stableTiming;
}
/**
* StableTiming
*
* It contains the fixed way of allocating time across the days of the period.
*
*/
@JsonProperty("stableTiming")
public void setStableTiming(List stableTiming) {
this.stableTiming = stableTiming;
}
/**
* VariableTiming
*
* It defines the allocating time as a total of time, maximum and minimun range of sections per day.
*
*/
@JsonProperty("variableTiming")
public VariableTiming getVariableTiming() {
return variableTiming;
}
/**
* VariableTiming
*
* It defines the allocating time as a total of time, maximum and minimun range of sections per day.
*
*/
@JsonProperty("variableTiming")
public void setVariableTiming(VariableTiming variableTiming) {
this.variableTiming = variableTiming;
}
/**
* StableTimingList
*
* It contains an array of 'StableTiming' distributions.
*
*/
@JsonProperty("stableTimingList")
public List> getStableTimingList() {
return stableTimingList;
}
/**
* StableTimingList
*
* It contains an array of 'StableTiming' distributions.
*
*/
@JsonProperty("stableTimingList")
public void setStableTimingList(List> stableTimingList) {
this.stableTimingList = stableTimingList;
}
}