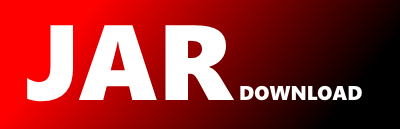
com.penalara.ghc.jsonghcfile.engineghcfile.Distribution Maven / Gradle / Ivy
Show all versions of engineInputGHCFile Show documentation
package com.penalara.ghc.jsonghcfile.engineghcfile;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* Distribution
*
* It contains information on how the class units of the session are distributed throughout the week. It must contain only one element between 'stablePeriods' and 'variablePeriods'.The 'periods' property is required if there is more than one period in the timetable. The 'stablePeriods' and 'variablePeriods' properties are mutually exclusive.
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"periods",
"stablePeriods",
"variablePeriods"
})
public class Distribution {
/**
* RefPeriods
*
* List of periods or weeks in which the class unit must be assigned.
*
*/
@JsonProperty("periods")
@JsonPropertyDescription("List of periods or weeks in which the class unit must be assigned.")
private List periods = new ArrayList();
/**
* StablePeriods
*
* The allocation of the class unit of the session is the same in each period.Only one property must be specified.
*
*/
@JsonProperty("stablePeriods")
@JsonPropertyDescription("The allocation of the class unit of the session is the same in each period.")
private StablePeriods stablePeriods;
/**
* VariablePeriods
*
* The total of the class units of the distribution are allocation between the periods.Properties 'weeklyStable' and 'weeklyVariable' are mutually exclusive.
*
*/
@JsonProperty("variablePeriods")
@JsonPropertyDescription("The total of the class units of the distribution are allocation between the periods.")
private VariablePeriods variablePeriods;
/**
* RefPeriods
*
* List of periods or weeks in which the class unit must be assigned.
*
*/
@JsonProperty("periods")
public List getPeriods() {
return periods;
}
/**
* RefPeriods
*
* List of periods or weeks in which the class unit must be assigned.
*
*/
@JsonProperty("periods")
public void setPeriods(List periods) {
this.periods = periods;
}
/**
* StablePeriods
*
* The allocation of the class unit of the session is the same in each period.Only one property must be specified.
*
*/
@JsonProperty("stablePeriods")
public StablePeriods getStablePeriods() {
return stablePeriods;
}
/**
* StablePeriods
*
* The allocation of the class unit of the session is the same in each period.Only one property must be specified.
*
*/
@JsonProperty("stablePeriods")
public void setStablePeriods(StablePeriods stablePeriods) {
this.stablePeriods = stablePeriods;
}
/**
* VariablePeriods
*
* The total of the class units of the distribution are allocation between the periods.Properties 'weeklyStable' and 'weeklyVariable' are mutually exclusive.
*
*/
@JsonProperty("variablePeriods")
public VariablePeriods getVariablePeriods() {
return variablePeriods;
}
/**
* VariablePeriods
*
* The total of the class units of the distribution are allocation between the periods.Properties 'weeklyStable' and 'weeklyVariable' are mutually exclusive.
*
*/
@JsonProperty("variablePeriods")
public void setVariablePeriods(VariablePeriods variablePeriods) {
this.variablePeriods = variablePeriods;
}
}