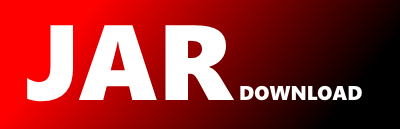
com.pentahohub.nexus.util.DateUtils Maven / Gradle / Ivy
package com.pentahohub.nexus.util;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
public class DateUtils {
public static Calendar toCalendar(Date date) {
if (date == null) {
return null;
}
Calendar c = Calendar.getInstance();
c.setTime(date);
return c;
}
public static int getYear(Date date) {
return toCalendar(date).get(Calendar.YEAR);
}
public static int getMonth(Date date) {
return toCalendar(date).get(Calendar.MONTH) + 1;
}
public static int getDay(Date date) {
return toCalendar(date).get(Calendar.DAY_OF_MONTH);
}
public static int getHour(Date date) {
return toCalendar(date).get(Calendar.HOUR_OF_DAY);
}
public static int getMinute(Date date) {
return toCalendar(date).get(Calendar.MINUTE);
}
public static int getSecond(Date date) {
return toCalendar(date).get(Calendar.SECOND);
}
public static long getMillis(Date date) {
return toCalendar(date).getTimeInMillis();
}
/**
* 获取一个月的天数
*/
public static int getDaysOfTheMonth(Date date) {
int year = getYear(date);
int month = getMonth(date) - 1;
int day = getDay(date);
int hour = getHour(date);
int minute = getMinute(date);
int second = getSecond(date);
Calendar calendar = new GregorianCalendar(year, month, day, hour, minute, second);
return calendar.getActualMaximum(Calendar.DAY_OF_MONTH);
}
public static Date parse(String date, String format) {
if (StringUtils.hasText(date) && StringUtils.hasText(format)) {
DateFormat df = new SimpleDateFormat(format);
try {
return df.parse(date);
} catch (ParseException e) {
throw new RuntimeException("Parse date exception");
}
}
return null;
}
public static Date getNextDate(Date date, int days) {
long time = date.getTime();
time = time + days * 24 * 60 * 60 * 1000;
return new Date(time);
}
public static String format(Date date, String format) {
if (date == null) {
return StringUtils.EMPTY;
}
return new SimpleDateFormat(format).format(date);
}
public static String format(long date, String pattern) {
return format(new Date(date), pattern);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy