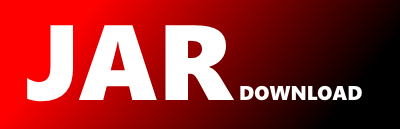
com.pentahohub.nexus.util.FileZipUtils Maven / Gradle / Ivy
package com.pentahohub.nexus.util;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.Enumeration;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
import java.util.zip.ZipOutputStream;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class FileZipUtils {
private static final Logger logger = LoggerFactory.getLogger(FileZipUtils.class);
public static void zip(File out, File... in) throws IOException {
ZipOutputStream zos = new ZipOutputStream(new FileOutputStream(out));
zip(zos, "", in);
zos.close();
logger.debug("*****************压缩完毕*******************");
}
private static void zip(ZipOutputStream out, String path, File... in) throws IOException {
path = path.replaceAll("\\*", "/");
byte[] buf = new byte[1024];
for (File inf : in) {
if (inf.isDirectory()) {
File[] files = inf.listFiles();
String srcPath = inf.getName();
srcPath = srcPath.replaceAll("\\*", "/");
if (!srcPath.endsWith("/")) {
srcPath += "/";
}
out.putNextEntry(new ZipEntry(path + srcPath));
zip(out, path + srcPath, files);
} else {
FileInputStream fis = new FileInputStream(inf);
logger.debug(path + inf.getName());
out.putNextEntry(new ZipEntry(path + inf.getName()));
int len;
while ((len = fis.read(buf)) > 0) {
out.write(buf, 0, len);
}
out.closeEntry();
fis.close();
}
}
}
@SuppressWarnings("resource")
public static void unzip(File zipFile, String outDir) throws IOException {
File pathFile = new File(outDir);
if (!pathFile.exists()) {
pathFile.mkdirs();
}
ZipFile zip = new ZipFile(zipFile);
Enumeration> entries = zip.entries();
while (entries.hasMoreElements()) {
ZipEntry entry = (ZipEntry) entries.nextElement();
String zipEntryName = entry.getName();
InputStream in = zip.getInputStream(entry);
String outPath = (outDir + zipEntryName).replaceAll("\\*", "/");
// 判断路径是否存在,不存在则创建文件路径
File file = new File(outPath.substring(0, outPath.lastIndexOf('/')));
if (!file.exists()) {
file.mkdirs();
}
// 判断文件全路径是否为文件夹,如果是上面已经上传,不需要解压
if (new File(outPath).isDirectory()) {
continue;
}
// 输出文件路径信息
logger.debug(outPath);
OutputStream out = new FileOutputStream(outPath);
byte[] buf1 = new byte[1024];
int len;
while ((len = in.read(buf1)) > 0) {
out.write(buf1, 0, len);
}
in.close();
out.close();
}
logger.debug("******************解压完毕********************");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy