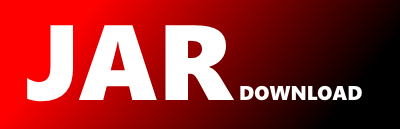
com.pentahohub.nexus.util.ImageCropUtils Maven / Gradle / Ivy
package com.pentahohub.nexus.util;
import java.awt.Graphics;
import java.awt.Image;
import java.awt.Toolkit;
import java.awt.image.BufferedImage;
import java.awt.image.CropImageFilter;
import java.awt.image.FilteredImageSource;
import java.awt.image.ImageFilter;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import javax.imageio.ImageIO;
import org.springframework.util.StreamUtils;
/**
* 图片剪裁工具类
*
* @author LUOXC
*/
public class ImageCropUtils {
/**
* 图像剪裁(按指定起点坐标和宽高切割)
*
* @param in
* 源图像
* @param out
* 剪裁后的图像
* @param x
* 目标图像起点坐标X
* @param y
* 目标图像起点坐标Y
* @param width
* 目标图像宽度
* @param height
* 目标图像高度
* @throws IOException
*/
public final static void crop(InputStream in, OutputStream out, int x, int y, int width, int height) throws IOException {
BufferedImage bi = ImageIO.read(in);
int srcHeight = bi.getHeight();
int srcWidth = bi.getWidth();
if (srcWidth > 0 && srcHeight > 0) {
Image image = bi.getScaledInstance(srcWidth, srcHeight, Image.SCALE_DEFAULT);
ImageFilter cropFilter = new CropImageFilter(x, y, width, height);
Image img = Toolkit.getDefaultToolkit().createImage(new FilteredImageSource(image.getSource(), cropFilter));
BufferedImage tag = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics g = tag.getGraphics();
g.drawImage(img, 0, 0, width, height, null);
g.dispose();
ImageIO.write(tag, ImageUtils.IMAGE_TYPE_PNG, out);
}
}
public final static void crop(File in, File out, int x, int y, int width, int height) throws IOException {
crop(new FileInputStream(in), new FileOutputStream(out), x, y, width, height);
}
public final static byte[] cropToByteArray(InputStream in, int x, int y, int width, int height) throws IOException {
ByteArrayOutputStream out = new ByteArrayOutputStream(StreamUtils.BUFFER_SIZE);
crop(in, out, x, y, width, height);
return out.toByteArray();
}
public final static byte[] cropToByteArray(File in, int x, int y, int width, int height) throws IOException {
return cropToByteArray(new FileInputStream(in), x, y, width, height);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy