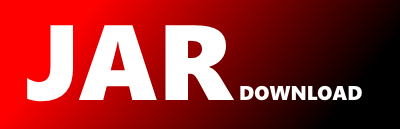
com.pentahohub.nexus.util.ImageUtils Maven / Gradle / Ivy
package com.pentahohub.nexus.util;
import java.awt.AlphaComposite;
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Image;
import java.awt.color.ColorSpace;
import java.awt.image.BufferedImage;
import java.awt.image.ColorConvertOp;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.Random;
import javax.imageio.ImageIO;
import org.springframework.util.StreamUtils;
/**
* 图片处理工具类
*
* @author LUOXC
*/
public class ImageUtils {
/**
* 图形交换格式
*/
public static String IMAGE_TYPE_GIF = "gif";
/**
* 联合照片专家组
*/
public static String IMAGE_TYPE_JPG = "jpg";
/**
* 联合照片专家组
*/
public static String IMAGE_TYPE_JPEG = "jpeg";
/**
* Windows 位图
*/
public static String IMAGE_TYPE_BMP = "bmp";
/**
* 可移植网络图形
*/
public static String IMAGE_TYPE_PNG = "png";
/**
* Photoshop的专用格式
*/
public static String IMAGE_TYPE_PSD = "psd";
/**
* 彩色转为黑白
*
* @param in
* 源图像
* @param out
* 目标图像
* @throws IOException
*/
public final static void gray(InputStream in, OutputStream out) throws IOException {
BufferedImage src = ImageIO.read(in);
ColorSpace cs = ColorSpace.getInstance(ColorSpace.CS_GRAY);
ColorConvertOp op = new ColorConvertOp(cs, null);
src = op.filter(src, null);
ImageIO.write(src, IMAGE_TYPE_PNG, out);
}
/**
* 给图片添加水印
*
* @param text
* 水印文字
* @param in
* 源图像
* @param out
* 目标图像
* @param font
* 水印的字体
* @param color
* 水印的字体颜色
* @param x
* 修正值
* @param y
* 修正值
* @param alpha
* 透明度:alpha 必须是范围 [0.0, 1.0] 之内(包含边界值)的一个浮点数字
* @throws IOException
*/
public final static void watermark(String text, InputStream in, OutputStream out, Font font, Color color, int x, int y, float alpha) throws IOException {
Image src = ImageIO.read(in);
int width = src.getWidth(null);
int height = src.getHeight(null);
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics2D g = image.createGraphics();
g.drawImage(src, 0, 0, width, height, null);
g.setColor(color);
g.setFont(font);
g.setComposite(AlphaComposite.getInstance(AlphaComposite.SRC_ATOP, alpha));
// 在指定坐标绘制水印文字
g.drawString(text, (width - (getLength(text) * font.getSize())) / 2 + x, (height - font.getSize()) / 2 + y);
g.dispose();
ImageIO.write((BufferedImage) image, IMAGE_TYPE_PNG, out);
}
/**
* 计算text的长度(一个中文算两个字符)
*
* @param text
* @return
*/
private final static int getLength(String text) {
int length = 0;
for (int i = 0; i < text.length(); i++) {
if (new String(text.charAt(i) + "").getBytes().length > 1) {
length += 2;
} else {
length += 1;
}
}
return length / 2;
}
/**
* 给图片添加水印
*
* @param image
* 水印图像
* @param in
* 源图像
* @param out
* 目标图像
* @param x
* 修正值
* @param y
* 修正值
* @param alpha
* 透明度:alpha 必须是范围 [0.0, 1.0] 之内(包含边界值)的一个浮点数字
* @throws IOException
*/
public final static void watermark(InputStream image, InputStream in, OutputStream out, int x, int y, float alpha) throws IOException {
Image src = ImageIO.read(in);
int wideth = src.getWidth(null);
int height = src.getHeight(null);
BufferedImage bi = new BufferedImage(wideth, height, BufferedImage.TYPE_INT_RGB);
Graphics2D g = bi.createGraphics();
g.drawImage(src, 0, 0, wideth, height, null);
// 水印文件
Image src_biao = ImageIO.read(image);
int wideth_biao = src_biao.getWidth(null);
int height_biao = src_biao.getHeight(null);
g.setComposite(AlphaComposite.getInstance(AlphaComposite.SRC_ATOP, alpha));
g.drawImage(src_biao, (wideth - wideth_biao) / 2, (height - height_biao) / 2, wideth_biao, height_biao, null);
// 水印文件结束
g.dispose();
ImageIO.write(bi, IMAGE_TYPE_PNG, out);
}
/**
* 生成验证码图片
*
* @param captcha
* 验证码字符
* @return byte[] 图片
* @throws IOException
*/
public static byte[] writeCaptchaToByteArray(String captcha) throws IOException {
ByteArrayOutputStream out = new ByteArrayOutputStream(StreamUtils.BUFFER_SIZE);
// 验证码长度
int codeLength = captcha.length();
// 字体大小
int fSize = 15;
int fWidth = fSize + 1;
// 图片宽度
int width = codeLength * fWidth + 6;
// 图片高度
int height = fSize * 2 + 1;
// 图片
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics g = image.createGraphics();
// 设置背景色
g.setColor(Color.WHITE);
// 填充背景
g.fillRect(0, 0, width, height);
// 设置边框颜色
g.setColor(Color.LIGHT_GRAY);
// 边框字体样式
g.setFont(new Font("Arial", Font.BOLD, height - 2));
// 绘制边框
g.drawRect(0, 0, width - 1, height - 1);
// 绘制噪点
Random rand = new Random();
// 设置噪点颜色
g.setColor(Color.LIGHT_GRAY);
for (int i = 0; i < codeLength * 6; i++) {
int x = rand.nextInt(width);
int y = rand.nextInt(height);
// 绘制1*1大小的矩形
g.drawRect(x, y, 1, 1);
}
// 绘制验证码
int codeY = height - 10;
// 设置字体颜色和样式
g.setColor(new Color(19, 148, 246));
g.setFont(new Font("Georgia", Font.BOLD, fSize));
for (int i = 0; i < codeLength; i++) {
g.drawString(String.valueOf(captcha.charAt(i)), i * 16 + 5, codeY);
}
// 关闭资源
g.dispose();
ImageIO.write(image, ImageUtils.IMAGE_TYPE_PNG, out);
return out.toByteArray();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy