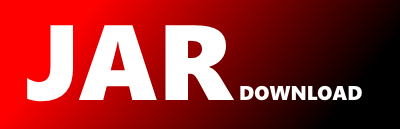
com.pentahohub.nexus.util.ImageZoomUtils Maven / Gradle / Ivy
package com.pentahohub.nexus.util;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Image;
import java.awt.geom.AffineTransform;
import java.awt.image.AffineTransformOp;
import java.awt.image.BufferedImage;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import javax.imageio.ImageIO;
import org.springframework.util.StreamUtils;
/**
* 图像缩放工具类
*
* @author LUOXC
*/
public class ImageZoomUtils {
/**
* 放大图像
*
* @param in
* 源图像文件地址
* @param out
* 放大后的图像地址
* @param scale
* 放大比例
* @throws IOException
*/
public final static void zoomIn(File in, File out, int scale) throws IOException {
zoom(in, out, scale, true);
}
/**
* 放大图像
*
* @param in
* 源图像
* @param out
* 放大后的图像
* @param scale
* 放大比例
* @throws IOException
*/
public final static void zoomIn(InputStream in, OutputStream out, int scale) throws IOException {
zoom(in, out, scale, true);
}
/**
* 缩小图像
*
* @param in
* 源图像文件地址
* @param out
* 缩小后的图像地址
* @param scale
* 缩小比例
* @throws IOException
*/
public final static void zoomOut(File in, File out, int scale) throws IOException {
zoom(in, out, scale, false);
}
/**
* 缩小图像
*
* @param in
* 源图像
* @param out
* 缩小后的图像
* @param scale
* 缩小比例
* @throws IOException
*/
public final static void zoomOut(InputStream in, OutputStream out, int scale) throws IOException {
zoom(in, out, scale, false);
}
/**
* 缩放图像(按比例缩放)
*
* @throws IOException
*/
private final static void zoom(File in, File out, int scale, boolean zoomIn) throws IOException {
zoom(new FileInputStream(in), new FileOutputStream(out), scale, zoomIn);
}
/**
* 缩放图像(按比例缩放)
*
* @throws IOException
*/
private final static void zoom(InputStream in, OutputStream out, int scale, boolean zoomIn) throws IOException {
BufferedImage src = ImageIO.read(in);
int width = src.getWidth();
int height = src.getHeight();
if (zoomIn) {
width = width * scale;
height = height * scale;
} else {
width = width / scale;
height = height / scale;
}
Image image = src.getScaledInstance(width, height, Image.SCALE_DEFAULT);
BufferedImage tag = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics g = tag.getGraphics();
g.drawImage(image, 0, 0, null);
g.dispose();
ImageIO.write(tag, ImageUtils.IMAGE_TYPE_PNG, out);
}
/**
* 缩放图像(按高度和宽度缩放)
*
* @param in
* 源图像文件地址
* @param out
* 缩放后的图像地址
* @param width
* 缩放后的宽度
* @param height
* 缩放后的高度
* @throws IOException
*/
public final static void zoom(File in, File out, int width, int height) throws IOException {
zoom(in, out, width, height, false);
}
/**
* 缩放图像(按高度和宽度缩放)
*
* @param in
* 源图像
* @param out
* 缩放后的图像
* @param width
* 缩放后的宽度
* @param height
* 缩放后的高度
* @throws IOException
*/
public final static void zoom(InputStream in, OutputStream out, int width, int height) throws IOException {
zoom(in, out, width, height, false);
}
/**
* 缩放图像(按高度和宽度缩放)
*
* @param in
* 源图像文件地址
* @param out
* 缩放后的图像地址
* @param width
* 缩放后的宽度
* @param height
* 缩放后的高度
* @param isFillWithWhite
* 比例不对时是否需要补白:true为补白; false为不补白;
* @throws IOException
*/
public final static void zoom(File in, File out, int width, int height, boolean isFillWithWhite) throws IOException {
zoom(new FileInputStream(in), new FileOutputStream(out), width, height, isFillWithWhite);
}
/**
* 缩放图像(按高度和宽度缩放)
*
* @param in
* 源图像
* @param out
* 缩放后的图像
* @param width
* 缩放后的宽度
* @param height
* 缩放后的高度
* @param isFillWithWhite
* 比例不对时是否需要补白:true为补白; false为不补白;
* @throws IOException
*/
public final static void zoom(InputStream in, OutputStream out, int width, int height, boolean isFillWithWhite) throws IOException {
BufferedImage src = ImageIO.read(in);
Image tag = src.getScaledInstance(width, height, BufferedImage.SCALE_SMOOTH);
// 计算比例
double ratio;
if ((src.getHeight() > height) || (src.getWidth() > width)) {
if (src.getHeight() > src.getWidth()) {
ratio = (new Integer(height)).doubleValue() / src.getHeight();
} else {
ratio = (new Integer(width)).doubleValue() / src.getWidth();
}
AffineTransformOp op = new AffineTransformOp(AffineTransform.getScaleInstance(ratio, ratio), null);
tag = op.filter(src, null);
}
if (isFillWithWhite) {
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics2D g = image.createGraphics();
g.setColor(Color.white);
g.fillRect(0, 0, width, height);
if (width == tag.getWidth(null))
g.drawImage(tag, 0, (height - tag.getHeight(null)) / 2, tag.getWidth(null), tag.getHeight(null), Color.white, null);
else
g.drawImage(tag, (width - tag.getWidth(null)) / 2, 0, tag.getWidth(null), tag.getHeight(null), Color.white, null);
g.dispose();
tag = image;
}
ImageIO.write((BufferedImage) tag, ImageUtils.IMAGE_TYPE_PNG, out);
}
public final static byte[] zoomToByteArray(File in, int width, int height) throws IOException {
return zoomToByteArray(in, width, height, false);
}
public final static byte[] zoomToByteArray(InputStream in, int width, int height) throws IOException {
return zoomToByteArray(in, width, height, false);
}
public final static byte[] zoomToByteArray(File in, int width, int height, boolean isFillWithWhite) throws IOException {
return zoomToByteArray(new FileInputStream(in), width, height, isFillWithWhite);
}
public final static byte[] zoomToByteArray(InputStream in, int width, int height, boolean isFillWithWhite) throws IOException {
ByteArrayOutputStream out = new ByteArrayOutputStream(StreamUtils.BUFFER_SIZE);
zoom(in, out, width, height, isFillWithWhite);
return out.toByteArray();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy