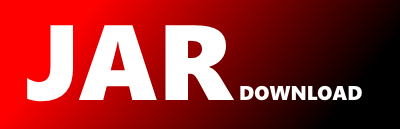
com.pentahohub.nexus.sms.EsoftSmsService Maven / Gradle / Ivy
The newest version!
package com.pentahohub.nexus.sms;
import java.io.IOException;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import org.apache.commons.lang3.time.DateUtils;
import org.dom4j.Document;
import org.dom4j.DocumentException;
import org.dom4j.DocumentHelper;
import org.dom4j.Element;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpRequest;
import org.springframework.http.client.ClientHttpRequestExecution;
import org.springframework.http.client.ClientHttpRequestInterceptor;
import org.springframework.http.client.ClientHttpResponse;
import org.springframework.http.client.SimpleClientHttpRequestFactory;
import org.springframework.http.client.support.HttpRequestWrapper;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.util.StringUtils;
import org.springframework.web.client.RestTemplate;
/**
* 短信发送服务 ——北京神州软科信息技术有限责任公司
*
* @author LUOXC
*/
public class EsoftSmsService implements SmsService {
private static final Logger logger = LoggerFactory.getLogger(EsoftSmsService.class);
private String url;
private String username;
private String password;
private SimpleClientHttpRequestFactory clientHttpRequestFactory;
public EsoftSmsService(String url, String username, String password) {
this.url = url;
this.username = username;
this.password = password;
clientHttpRequestFactory = new SimpleClientHttpRequestFactory();
clientHttpRequestFactory.setReadTimeout(10000);
clientHttpRequestFactory.setConnectTimeout(10000);
}
public void send(String toMobilePhone, String messageContent) throws SmsException {
try {
RestTemplate restTemplate = new RestTemplate(clientHttpRequestFactory);
List interceptors = new ArrayList();
interceptors.add(new ClientHttpRequestInterceptor() {
public ClientHttpResponse intercept(HttpRequest request, byte[] body, ClientHttpRequestExecution execution) throws IOException {
HttpRequestWrapper requestWrapper = new HttpRequestWrapper(request);
requestWrapper.getHeaders().add("Content-Type", "application/x-www-form-urlencoded");
return execution.execute(requestWrapper, body);
}
});
MultiValueMap request = new LinkedMultiValueMap();
request.add("CorpID", username);
request.add("Pwd", password);
request.add("Mobile", toMobilePhone);
request.add("Content", messageContent);
request.add("Cell", "");
request.add("SendTime", "");
String response = restTemplate.postForObject("{host}/WS.asmx/BatchSend", request, String.class, url);
Document doc = DocumentHelper.parseText(response);
Element root = doc.getRootElement();
String result = root.getTextTrim();
if ("-1".equals(result)) {
throw new SmsException("账号未注册");
} else if ("-2".equals(result)) {
throw new SmsException("其他错误");
} else if ("-3".equals(result)) {
throw new SmsException("密码错误");
} else if ("-4".equals(result)) {
throw new SmsException("一次提交信息不能超过10000个手机号码,号码逗号隔开");
} else if ("-5".equals(result)) {
throw new SmsException("余额不足");
} else if ("-6".equals(result)) {
throw new SmsException("定时发送时间不是有效的时间格式");
} else if ("-8".equals(result)) {
throw new SmsException("发送内容需在3到250字之间");
} else if ("-9".equals(result)) {
throw new SmsException("发送号码为空");
}
logger.info("SMS:/Send/{} {}", toMobilePhone, messageContent);
} catch (Exception e) {
throw new SmsException(e);
}
}
public List get() throws SmsException {
try {
return get("{host}/WS.asmx/Get?CorpID={username}&Pwd={password}", url, username, password);
} catch (Exception e) {
throw new SmsException(e);
}
}
public List get(String mobilePhone) throws SmsException {
try {
return get("{host}/WS.asmx/GetOne?CorpID={username}&Pwd={password}&Phone={mobilePhone}", url, username, password, mobilePhone);
} catch (Exception e) {
throw new SmsException(e);
}
}
private List get(String url, Object... urlVariables) throws DocumentException, SmsException {
RestTemplate restTemplate = new RestTemplate(clientHttpRequestFactory);
String response = restTemplate.getForObject(url, String.class, urlVariables);
Document doc = DocumentHelper.parseText(response);
Element root = doc.getRootElement();
String result = root.getTextTrim();
if ("-1".equals(result)) {
throw new SmsException("账号未注册");
} else if ("-2".equals(result)) {
throw new SmsException("其他错误");
} else if ("-3".equals(result)) {
throw new SmsException("密码错误");
} else {
List msgs = new ArrayList();
if (!StringUtils.isEmpty(result)) {
logger.info("SMS:/Get {}", result);
String[] msgsArray = result.substring(2).split("\\|\\|");
for (String tmp : msgsArray) {
String[] fields = tmp.split("#");
SmsMessage msg = new SmsMessage();
msg.setPhone(fields[0]);
msg.setContent(fields[1]);
try {
msg.setTime(DateUtils.parseDate(fields[2], new String[] { "yyyy-MM-dd HH:mm:ss" }));
} catch (ParseException e) {
msg.setTime(new Date());
}
msgs.add(msg);
}
}
return msgs;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy