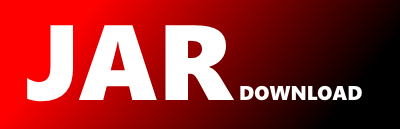
com.pepperize.cdk.github.GithubApiCall Maven / Gradle / Ivy
Show all versions of cdk-github Show documentation
package com.pepperize.cdk.github;
/**
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.97.0 (build 729de35)", date = "2024-06-03T23:55:30.179Z")
@software.amazon.jsii.Jsii(module = com.pepperize.cdk.github.$Module.class, fqn = "@pepperize/cdk-github.GithubApiCall")
@software.amazon.jsii.Jsii.Proxy(GithubApiCall.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface GithubApiCall extends software.amazon.jsii.JsiiSerializable {
/**
* The endpoint to call.
*
* @see https://github.com/octokit/rest.js
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getEndpoint();
/**
* The method to call.
*
* @see https://github.com/octokit/rest.js
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getMethod();
/**
* The regex pattern to use to catch API errors.
*
* The message
property of the RequestError
object will be tested against this pattern. If there is a match an error will not be thrown.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getIgnoreErrorCodesMatching() {
return null;
}
/**
* Filter the data returned by the custom resource to specific paths in the API response.
*
* The total size of the response body can't exceed 4096 bytes.
*
* Default: undefined - it's recommended to define it
*
* @see https://docs.github.com/en/rest
* Example for octokit.rest.repos.createInOrg: ['id', 'full_name', 'owner.id']
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getOutputPaths() {
return null;
}
/**
* The parameters for the service action.
*
* @see https://github.com/octokit/rest.js
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getParameters() {
return null;
}
/**
* The physical resource id of the custom resource for this call.
*
* Default: undefined - for "Create" requests, defaults to the event's RequestId, for "Update" and "Delete", defaults to the current `PhysicalResourceId`.
*
* @see https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/crpg-ref-responses.html
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.customresources.PhysicalResourceId getPhysicalResourceId() {
return null;
}
/**
* @return a {@link Builder} of {@link GithubApiCall}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link GithubApiCall}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String endpoint;
java.lang.String method;
java.lang.String ignoreErrorCodesMatching;
java.util.List outputPaths;
java.lang.Object parameters;
software.amazon.awscdk.customresources.PhysicalResourceId physicalResourceId;
/**
* Sets the value of {@link GithubApiCall#getEndpoint}
* @param endpoint The endpoint to call. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder endpoint(java.lang.String endpoint) {
this.endpoint = endpoint;
return this;
}
/**
* Sets the value of {@link GithubApiCall#getMethod}
* @param method The method to call. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder method(java.lang.String method) {
this.method = method;
return this;
}
/**
* Sets the value of {@link GithubApiCall#getIgnoreErrorCodesMatching}
* @param ignoreErrorCodesMatching The regex pattern to use to catch API errors.
* The message
property of the RequestError
object will be tested against this pattern. If there is a match an error will not be thrown.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ignoreErrorCodesMatching(java.lang.String ignoreErrorCodesMatching) {
this.ignoreErrorCodesMatching = ignoreErrorCodesMatching;
return this;
}
/**
* Sets the value of {@link GithubApiCall#getOutputPaths}
* @param outputPaths Filter the data returned by the custom resource to specific paths in the API response.
* The total size of the response body can't exceed 4096 bytes.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder outputPaths(java.util.List outputPaths) {
this.outputPaths = outputPaths;
return this;
}
/**
* Sets the value of {@link GithubApiCall#getParameters}
* @param parameters The parameters for the service action.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parameters(java.lang.Object parameters) {
this.parameters = parameters;
return this;
}
/**
* Sets the value of {@link GithubApiCall#getPhysicalResourceId}
* @param physicalResourceId The physical resource id of the custom resource for this call.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder physicalResourceId(software.amazon.awscdk.customresources.PhysicalResourceId physicalResourceId) {
this.physicalResourceId = physicalResourceId;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link GithubApiCall}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public GithubApiCall build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link GithubApiCall}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements GithubApiCall {
private final java.lang.String endpoint;
private final java.lang.String method;
private final java.lang.String ignoreErrorCodesMatching;
private final java.util.List outputPaths;
private final java.lang.Object parameters;
private final software.amazon.awscdk.customresources.PhysicalResourceId physicalResourceId;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.endpoint = software.amazon.jsii.Kernel.get(this, "endpoint", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.method = software.amazon.jsii.Kernel.get(this, "method", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.ignoreErrorCodesMatching = software.amazon.jsii.Kernel.get(this, "ignoreErrorCodesMatching", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.outputPaths = software.amazon.jsii.Kernel.get(this, "outputPaths", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.parameters = software.amazon.jsii.Kernel.get(this, "parameters", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.physicalResourceId = software.amazon.jsii.Kernel.get(this, "physicalResourceId", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.customresources.PhysicalResourceId.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.endpoint = java.util.Objects.requireNonNull(builder.endpoint, "endpoint is required");
this.method = java.util.Objects.requireNonNull(builder.method, "method is required");
this.ignoreErrorCodesMatching = builder.ignoreErrorCodesMatching;
this.outputPaths = builder.outputPaths;
this.parameters = builder.parameters;
this.physicalResourceId = builder.physicalResourceId;
}
@Override
public final java.lang.String getEndpoint() {
return this.endpoint;
}
@Override
public final java.lang.String getMethod() {
return this.method;
}
@Override
public final java.lang.String getIgnoreErrorCodesMatching() {
return this.ignoreErrorCodesMatching;
}
@Override
public final java.util.List getOutputPaths() {
return this.outputPaths;
}
@Override
public final java.lang.Object getParameters() {
return this.parameters;
}
@Override
public final software.amazon.awscdk.customresources.PhysicalResourceId getPhysicalResourceId() {
return this.physicalResourceId;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("endpoint", om.valueToTree(this.getEndpoint()));
data.set("method", om.valueToTree(this.getMethod()));
if (this.getIgnoreErrorCodesMatching() != null) {
data.set("ignoreErrorCodesMatching", om.valueToTree(this.getIgnoreErrorCodesMatching()));
}
if (this.getOutputPaths() != null) {
data.set("outputPaths", om.valueToTree(this.getOutputPaths()));
}
if (this.getParameters() != null) {
data.set("parameters", om.valueToTree(this.getParameters()));
}
if (this.getPhysicalResourceId() != null) {
data.set("physicalResourceId", om.valueToTree(this.getPhysicalResourceId()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@pepperize/cdk-github.GithubApiCall"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
GithubApiCall.Jsii$Proxy that = (GithubApiCall.Jsii$Proxy) o;
if (!endpoint.equals(that.endpoint)) return false;
if (!method.equals(that.method)) return false;
if (this.ignoreErrorCodesMatching != null ? !this.ignoreErrorCodesMatching.equals(that.ignoreErrorCodesMatching) : that.ignoreErrorCodesMatching != null) return false;
if (this.outputPaths != null ? !this.outputPaths.equals(that.outputPaths) : that.outputPaths != null) return false;
if (this.parameters != null ? !this.parameters.equals(that.parameters) : that.parameters != null) return false;
return this.physicalResourceId != null ? this.physicalResourceId.equals(that.physicalResourceId) : that.physicalResourceId == null;
}
@Override
public final int hashCode() {
int result = this.endpoint.hashCode();
result = 31 * result + (this.method.hashCode());
result = 31 * result + (this.ignoreErrorCodesMatching != null ? this.ignoreErrorCodesMatching.hashCode() : 0);
result = 31 * result + (this.outputPaths != null ? this.outputPaths.hashCode() : 0);
result = 31 * result + (this.parameters != null ? this.parameters.hashCode() : 0);
result = 31 * result + (this.physicalResourceId != null ? this.physicalResourceId.hashCode() : 0);
return result;
}
}
}