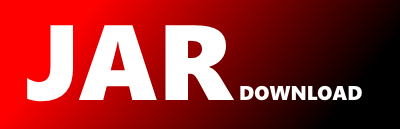
com.pepperize.cdk.github.GithubCustomResource Maven / Gradle / Ivy
Show all versions of cdk-github Show documentation
package com.pepperize.cdk.github;
/**
*
*
* new GithubCustomResource(scope, "GithubRepo", {
* onCreate: {
* // https://octokit.github.io/rest.js/v19/#repos-create-in-org
* endpoint: "repos",
* method: "createInOrg",
* parameters: {
* org: "pepperize",
* name: "cdk-github",
* },
* outputPaths: ["id", "full_name"],
* physicalResourceId: custom_resources.PhysicalResourceId.fromResponse("full_name"),
* ignoreErrorCodesMatching: "name already exists on this account",
* },
* onUpdate: {
* // https://octokit.github.io/rest.js/v19#repos-get
* endpoint: "repos",
* method: "get",
* parameters: {
* owner: "pepperize",
* repo: "cdk-github",
* },
* outputPaths: ["id", "full_name"],
* physicalResourceId: custom_resources.PhysicalResourceId.fromResponse("full_name"),
* },
* onDelete: {
* // https://octokit.github.io/rest.js/v19#repos-delete
* endpoint: "repos",
* method: "delete",
* parameters: {
* owner: "pepperize",
* repo: "cdk-github",
* },
* outputPaths: [],
* },
* authOptions: AuthOptions.appAuth(auth),
* });
*
*
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.97.0 (build 729de35)", date = "2024-06-03T23:55:30.186Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = com.pepperize.cdk.github.$Module.class, fqn = "@pepperize/cdk-github.GithubCustomResource")
public class GithubCustomResource extends com.pepperize.cdk.github.GithubCustomResourceBase {
protected GithubCustomResource(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected GithubCustomResource(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public GithubCustomResource(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull com.pepperize.cdk.github.GithubCustomResourceProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* A fluent builder for {@link com.pepperize.cdk.github.GithubCustomResource}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final com.pepperize.cdk.github.GithubCustomResourceProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new com.pepperize.cdk.github.GithubCustomResourceProps.Builder();
}
/**
* Currently, supports only GitHub App.
*
*
* const auth = { appId, privateKey };
* const installationAuth = { appId, privateKey, installationId };
*
*
* @return {@code this}
* @see https://github.com/octokit/authentication-strategies.js/#github-app-or-installation-authentication
* @param authOptions Currently, supports only GitHub App. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder authOptions(final com.pepperize.cdk.github.IAuthOptions authOptions) {
this.props.authOptions(authOptions);
return this;
}
/**
* The GitHub Api call to make when the resource is created.
*
* @return {@code this}
* @param onCreate The GitHub Api call to make when the resource is created. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder onCreate(final com.pepperize.cdk.github.GithubApiCall onCreate) {
this.props.onCreate(onCreate);
return this;
}
/**
* The GitHub Api call to make when the resource is deleted.
*
* @return {@code this}
* @param onDelete The GitHub Api call to make when the resource is deleted. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder onDelete(final com.pepperize.cdk.github.GithubApiCall onDelete) {
this.props.onDelete(onDelete);
return this;
}
/**
* The GitHub Api call to make when the resource is updated.
*
* @return {@code this}
* @param onUpdate The GitHub Api call to make when the resource is updated. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder onUpdate(final com.pepperize.cdk.github.GithubApiCall onUpdate) {
this.props.onUpdate(onUpdate);
return this;
}
/**
* Cloudformation Resource type.
*
* @return {@code this}
* @param resourceType Cloudformation Resource type. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder resourceType(final java.lang.String resourceType) {
this.props.resourceType(resourceType);
return this;
}
/**
* @return a newly built instance of {@link com.pepperize.cdk.github.GithubCustomResource}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public com.pepperize.cdk.github.GithubCustomResource build() {
return new com.pepperize.cdk.github.GithubCustomResource(
this.scope,
this.id,
this.props.build()
);
}
}
}