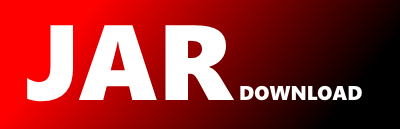
com.pepperize.cdk.github.package-info Maven / Gradle / Ivy
Show all versions of cdk-github Show documentation
/**
*
*
*
*
*
*
*
*
*
*
*
CDK Github
*
* Manage GitHub resources like repositories, teams, members, integrations and workflows with the AWS CDK as Custom Resources in CloudFormation with cdk-github.
*
*
*
* You configure the endpoint, method and parameters documented by @octokit/rest and AWS CloudFormation runs them anytime you create, update (if you changed the custom resource), or delete stacks. When CloudFormation sends a lifecycle event notification, then your custom resource sends the request to the GitHub REST API.
*
*
*
*
Install
*
* TypeScript
*
*
* npm install @pepperize/cdk-github
*
*
* or
*
*
* yarn add @pepperize/cdk-github
*
*
*
Python
*
*
* pip install pepperize.cdk-github
*
*
*
C#
*
*
* dotnet add package Pepperize.CDK.Github
*
*
*
Java
*
*
* <dependency>
* <groupId>com.pepperize</groupId>
* <artifactId>cdk-github</artifactId>
* <version>${cdkGithub.version}</version>
* </dependency>
*
*
*
*
*
Contributing
*
* Contributions of all kinds are welcome :rocket: Check out our contributor's guide.
*
* For a quick start, fork and check out a development environment:
*
*
* git clone git@github.com:pepperize/cdk-github
* cd cdk-github
* # install dependencies
* yarn
* # build with projen
* yarn build
*
*
*
Getting Started
*
*
* - Creating a GitHub App
* - Installing GitHub Apps
* - Create an AWS Secrets Manager secret
*
*
* {
* "appId": "123456",
* "privateKey": "-----BEGIN RSA PRIVATE KEY-----\nExample==\n-----END RSA PRIVATE KEY-----",
* "installationId": "12345678"
* }
*
* - Add @pepperize/cdk-github to your project dependencies
*
*
* yarn add @pepperize/cdk-github
*
* - Add your
main.ts
*
*
* const app = new App();
* const stack = new Stack(app, "GithubCustomResources");
*
*
*
*
* Just for simplicity, it's up to you how to organize your app :wink:
*
*
* - Import your secret
*
*
* const secret = secrets_manager.Secret.fromSecretNameV2(stack, "Auth", "cdk-github/github-token");
*
* - Configure GitHub App authenticate as an installation
*
*
* const authOptions = AuthOptions.appAuth(secret);
*
* - Add your first GitHub Custom Resource with the AWS CDK
*
*
* new GithubCustomResource(stack, "GithubRepo", {
* onCreate: {
* // 👇The endpoint of the GitHub API.
* endpoint: "repos",
* // 👇The method of the GitHub API.
* method: "createInOrg",
* // https://octokit.github.io/rest.js/v19/#repos-create-in-org
* parameters: {
* // 👇The request parameters to send.
* org: "pepperize",
* name: "cdk-github",
* },
* // 👇The object keys from the GitHub API response to return to CFN.
* outputPaths: ["id", "full_name"],
* // 👇This becomes the CFN Physical ID visible in the Console.
* physicalResourceId: custom_resources.PhysicalResourceId.fromResponse("full_name"),
* // 👇Don't throw an error if message matching this regex.
* ignoreErrorCodesMatching: "name already exists on this account",
* },
* // 👇The implemented authentication strategy.
* authOptions: AuthOptions.appAuth(secret),
* });
*
* - Deploy your first GitHub Custom Resource
*
*
* npx cdk deploy
*
*
*
*
Authentication
*
*
GitHub App or installation authentication
*
* Configure the AWS SecretsManager Secret with the AuthOptions that will be passed to octokit.auth
. i.e. as an installation:
*
*
* {
* "appId": "123456",
* "privateKey": "-----BEGIN RSA PRIVATE KEY-----\nExample==\n-----END RSA PRIVATE KEY-----",
* "installationId": "12345678"
* }
*
*
* Lookup the secret in your AWS CDK app:
*
*
* // 👇Lookup your secret containing the AuthOptions
* const secret = secrets_manager.Secret.fromSecretNameV2(stack, "Auth", "cdk-github/github-token");
* // 👇This will send the secret arn to the custom resource handler
* const authOptions = AuthOptions.appAuth(secret);
*
*
* The custom resource handler will configure octokit.js with the createAppAuth
:
*
*
* const getSecretValueResponse = await SSM.getSecretValue({ SecretId: secret }).promise();
* const octokitOptions: OctokitOptions = {
* authStrategy: createAppAuth,
* auth: (auth = JSON.parse(getSecretValueResponse.SecretString)),
* };
*
*
*
*
* Supported through @octokit/auth-app
*
*
*
*
Personal Access Token authentication
*
* Just add your PAT to an SSM StringParameter
*
*
* // 👇Lookup your parameter containing the TOKEN
* const parameter = ssm.StringParameter.fromStringParameterName(stack, "Auth", "cdk-github/github-token");
* // 👇This will send the parameter arn to the custom resource handler
* const authOptions = AuthOptions.tokenAuth(parameter);
*
*
*
*
* Supported through @octokit/auth-token
*
*
*
*
Unauthenticated
*
*
* // 👇This will configure octokit without authentication
* const authOptions = AuthOptions.unauthenticated();
*
*
*
Manage a GitHub Repository - Example
*
* @octokit/plugin-rest-endpoint-methods
*
*
* const auth = secrets_manager.Secret.fromSecretNameV2(stack, "Auth", "cdk-github/github-token");
*
* const repo = new GithubCustomResource(stack, "GithubRepo", {
* onCreate: {
* // https://octokit.github.io/rest.js/v19/#repos-create-in-org
* endpoint: "repos",
* method: "createInOrg",
* parameters: {
* org: "pepperize",
* name: "cdk-github",
* },
* outputPaths: ["id", "full_name"],
* physicalResourceId: custom_resources.PhysicalResourceId.fromResponse("full_name"),
* ignoreErrorCodesMatching: "name already exists on this account",
* },
* onUpdate: {
* // https://octokit.github.io/rest.js/v19#repos-get
* endpoint: "repos",
* method: "get",
* parameters: {
* owner: "pepperize",
* repo: "cdk-github",
* },
* outputPaths: ["id", "full_name"],
* physicalResourceId: custom_resources.PhysicalResourceId.fromResponse("full_name"),
* },
* onDelete: {
* // https://octokit.github.io/rest.js/v19#repos-delete
* endpoint: "repos",
* method: "delete",
* parameters: {
* owner: "pepperize",
* repo: "cdk-github",
* },
* outputPaths: [],
* },
* authOptions: AuthOptions.appAuth(auth),
* });
*
* // 👇 This will return the created repository id as a CDK Token
* repo.getAtt("id");
*
*
*
Manage GitHub Actions Secrets
*
*
Environment Secret
*
* Manages an environment secret. Will fetch the source AWS SecretsManager secret and encrypt it to store in GitHub.
*
*
* // 👇The GitHub API authentication secret
* const auth = secrets_manager.Secret.fromSecretNameV2(scope, "Auth", "cdk-github/github-token");
*
* // 👇The AWS SecretsManager Secret to configure as GitHub Action secret.
* const secret = secrets_manager.Secret.fromSecretNameV2(scope, "Secret", "any-secret/example");
*
* new GithubActionsSecretEnvironment(scope, "GithubRepo", {
* // 👇The repository id, which you may lookup from the page source or via a custom resource
* repositoryId: "558989134",
* environmentName: "production",
* // 👇The name of the created GitHub secret
* secretName: "example",
* // 👇The source AWS SecretsManager secret and JSON field to use
* source: GithubActionsSecret.fromSecretsManager(secret, "some-json-field"),
* authOptions: AuthOptions.appAuth(auth),
* // 👇Whether to delete or retain the GitHub secret on resource removal
* removalPolicy: RemovalPolicy.DESTROY,
* });
*
*
*
*
* You may retrieve the repository_id
from the GitHub Repository page source's meta tag i.e. <meta name="octolytics-dimension-repository_id" content="558989134">
or from another GithubCustomResource
via getAtt()
.
*
*
*
* See GitHub Developer Guide, API Reference
*
*
Organization Secret
*
* Manage an GitHib Actions organization secret. Will fetch the source AWS SecretsManager secret and encrypt it to store in GitHub.
*
*
* // 👇The GitHub API authentication secret
* const auth = secrets_manager.Secret.fromSecretNameV2(scope, "Auth", "cdk-github/github-token");
*
* // 👇The AWS SecretsManager Secret to configure as GitHub Action secret.
* const secret = secrets_manager.Secret.fromSecretNameV2(scope, "Secret", "any-secret/example");
*
* new GithubActionsSecretOrganization(scope, "GithubRepo", {
* organizationName: "pepperize",
* // 👇The name of the created GitHub secret
* secretName: "example",
* // 👇The source AWS SecretsManager secret and JSON field to use
* source: GithubActionsSecret.fromSecretsManager(secret, "some-json-field"),
* visibility: Visibility.ALL,
* authOptions: AuthOptions.appAuth(auth),
* // 👇Whether to delete or retain the GitHub secret on resource removal
* removalPolicy: RemovalPolicy.DESTROY,
* });
*
*
* See GitHub Developer Guide, API Reference
*
*
Repository Secret
*
* Manage an GitHib Actions Repository secret. Will fetch the source AWS SecretsManager secret and encrypt it to store in GitHub.
*
*
* // 👇The GitHub API authentication secret
* const auth = secrets_manager.Secret.fromSecretNameV2(scope, "Auth", "cdk-github/github-token");
*
* // 👇The AWS SecretsManager Secret to configure as GitHub Action secret.
* const secret = secrets_manager.Secret.fromSecretNameV2(scope, "Secret", "any-secret/example");
*
* new GithubActionsSecretRepository(scope, "GithubRepo", {
* owner: "pepperize",
* repositoryName: "cdk-github",
* // 👇The name of the created GitHub secret
* secretName: "example",
* // 👇The source AWS SecretsManager secret and JSON field to use
* source: GithubActionsSecret.fromSecretsManager(secret, "some-json-field"),
* authOptions: AuthOptions.appAuth(auth),
* // 👇Whether to delete or retain the GitHub secret on resource removal
* removalPolicy: RemovalPolicy.DESTROY,
* });
*
*
* See GitHub Developer Guide, API Reference
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
package com.pepperize.cdk.github;