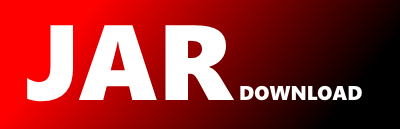
com.pepperize.cdk.organizations.Account Maven / Gradle / Ivy
Show all versions of cdk-organizations Show documentation
package com.pepperize.cdk.organizations;
/**
* Creates or imports an AWS account that is automatically a member of the organization whose credentials made the request.
*
* AWS Organizations automatically copies the information from the management account to the new member account
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.98.0 (build 00b106d)", date = "2024-05-17T01:18:02.586Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = com.pepperize.cdk.organizations.$Module.class, fqn = "@pepperize/cdk-organizations.Account")
public class Account extends software.constructs.Construct implements com.pepperize.cdk.organizations.IAccount, com.pepperize.cdk.organizations.ITaggableResource {
protected Account(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected Account(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Account(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull com.pepperize.cdk.organizations.AccountProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Attach a policy.
*
* Before you can attach the policy, you must enable that policy type for use. You can use policies when you have all features enabled.
*
* @see https://docs.aws.amazon.com/organizations/latest/userguide/orgs_manage_policies.html
* @param policy This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void attachPolicy(final @org.jetbrains.annotations.NotNull com.pepperize.cdk.organizations.IPolicy policy) {
software.amazon.jsii.Kernel.call(this, "attachPolicy", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(policy, "policy is required") });
}
/**
* Enables trusted access for the AWS service (trusted service) as Delegated Administrator, which performs tasks in your organization and its accounts on your behalf.
*
* @param servicePrincipal The supported AWS service that you specify. This parameter is required.
* @param region The region to delegate in.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void delegateAdministrator(final @org.jetbrains.annotations.NotNull java.lang.String servicePrincipal, final @org.jetbrains.annotations.Nullable java.lang.String region) {
software.amazon.jsii.Kernel.call(this, "delegateAdministrator", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(servicePrincipal, "servicePrincipal is required"), region });
}
/**
* Enables trusted access for the AWS service (trusted service) as Delegated Administrator, which performs tasks in your organization and its accounts on your behalf.
*
* @param servicePrincipal The supported AWS service that you specify. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void delegateAdministrator(final @org.jetbrains.annotations.NotNull java.lang.String servicePrincipal) {
software.amazon.jsii.Kernel.call(this, "delegateAdministrator", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(servicePrincipal, "servicePrincipal is required") });
}
/**
* The unique identifier (ID) of the parent root, organizational unit (OU), account, or policy that you want to create the new OU in.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull java.lang.String identifier() {
return software.amazon.jsii.Kernel.call(this, "identifier", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The Amazon Resource Name (ARN) of the account.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAccountArn() {
return software.amazon.jsii.Kernel.get(this, "accountArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* If the account was created successfully, the unique identifier (ID) of the new account.
*
* Exactly 12 digits.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAccountId() {
return software.amazon.jsii.Kernel.get(this, "accountId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The friendly name of the account.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAccountName() {
return software.amazon.jsii.Kernel.get(this, "accountName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The email address of the owner to assign to the new member account.
*
* This email address must not already be associated with another AWS account. You must use a valid email address to complete account creation. You can't access the root user of the account or remove an account that was created with an invalid email address.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getEmail() {
return software.amazon.jsii.Kernel.get(this, "email", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull software.amazon.awscdk.CustomResource getResource() {
return software.amazon.jsii.Kernel.get(this, "resource", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.CustomResource.class));
}
/**
* TagManager to set, remove and format tags.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.TagManager getTags() {
return software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.TagManager.class));
}
/**
* A fluent builder for {@link com.pepperize.cdk.organizations.Account}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final com.pepperize.cdk.organizations.AccountProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new com.pepperize.cdk.organizations.AccountProps.Builder();
}
/**
* The friendly name of the member account.
*
* @return {@code this}
* @param accountName The friendly name of the member account. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder accountName(final java.lang.String accountName) {
this.props.accountName(accountName);
return this;
}
/**
* The email address of the owner to assign to the new member account.
*
* This email address must not already be associated with another AWS account. You must use a valid email address to complete account creation. You can't access the root user of the account or remove an account that was created with an invalid email address.
*
* @return {@code this}
* @param email The email address of the owner to assign to the new member account. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder email(final java.lang.String email) {
this.props.email(email);
return this;
}
/**
* If set to ALLOW , the new account enables IAM users to access account billing information if they have the required permissions.
*
* If set to DENY , only the root user of the new account can access account billing information.
*
* Default: ALLOW
*
* @return {@code this}
* @param iamUserAccessToBilling If set to ALLOW , the new account enables IAM users to access account billing information if they have the required permissions. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder iamUserAccessToBilling(final com.pepperize.cdk.organizations.IamUserAccessToBilling iamUserAccessToBilling) {
this.props.iamUserAccessToBilling(iamUserAccessToBilling);
return this;
}
/**
* Whether to import, if a duplicate account with same name and email already exists.
*
* Default: true
*
* @return {@code this}
* @param importOnDuplicate Whether to import, if a duplicate account with same name and email already exists. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder importOnDuplicate(final java.lang.Boolean importOnDuplicate) {
this.props.importOnDuplicate(importOnDuplicate);
return this;
}
/**
* The parent root or OU that you want to create the new Account in.
*
* @return {@code this}
* @param parent The parent root or OU that you want to create the new Account in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parent(final com.pepperize.cdk.organizations.IParent parent) {
this.props.parent(parent);
return this;
}
/**
* If set to RemovalPolicy.DESTROY, the account will be moved to the root.
*
* Default: RemovalPolicy.Retain
*
* @return {@code this}
* @param removalPolicy If set to RemovalPolicy.DESTROY, the account will be moved to the root. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder removalPolicy(final software.amazon.awscdk.RemovalPolicy removalPolicy) {
this.props.removalPolicy(removalPolicy);
return this;
}
/**
* The name of an IAM role that AWS Organizations automatically preconfigures in the new member account.
*
* This role trusts the management account, allowing users in the management account to assume the role, as permitted by the management account administrator. The role has administrator permissions in the new member account.
*
* If you don't specify this parameter, the role name defaults to OrganizationAccountAccessRole.
*
* @return {@code this}
* @param roleName The name of an IAM role that AWS Organizations automatically preconfigures in the new member account. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder roleName(final java.lang.String roleName) {
this.props.roleName(roleName);
return this;
}
/**
* @return a newly built instance of {@link com.pepperize.cdk.organizations.Account}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public com.pepperize.cdk.organizations.Account build() {
return new com.pepperize.cdk.organizations.Account(
this.scope,
this.id,
this.props.build()
);
}
}
}