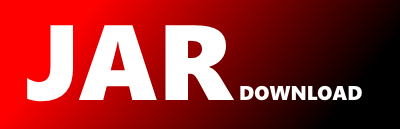
com.pepperize.cdk.organizations.AccountProps Maven / Gradle / Ivy
Show all versions of cdk-organizations Show documentation
package com.pepperize.cdk.organizations;
/**
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.98.0 (build 00b106d)", date = "2024-05-17T01:18:02.593Z")
@software.amazon.jsii.Jsii(module = com.pepperize.cdk.organizations.$Module.class, fqn = "@pepperize/cdk-organizations.AccountProps")
@software.amazon.jsii.Jsii.Proxy(AccountProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface AccountProps extends software.amazon.jsii.JsiiSerializable {
/**
* The friendly name of the member account.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getAccountName();
/**
* The email address of the owner to assign to the new member account.
*
* This email address must not already be associated with another AWS account. You must use a valid email address to complete account creation. You can't access the root user of the account or remove an account that was created with an invalid email address.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getEmail();
/**
* If set to ALLOW , the new account enables IAM users to access account billing information if they have the required permissions.
*
* If set to DENY , only the root user of the new account can access account billing information.
*
* Default: ALLOW
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable com.pepperize.cdk.organizations.IamUserAccessToBilling getIamUserAccessToBilling() {
return null;
}
/**
* Whether to import, if a duplicate account with same name and email already exists.
*
* Default: true
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getImportOnDuplicate() {
return null;
}
/**
* The parent root or OU that you want to create the new Account in.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable com.pepperize.cdk.organizations.IParent getParent() {
return null;
}
/**
* If set to RemovalPolicy.DESTROY, the account will be moved to the root.
*
* Default: RemovalPolicy.Retain
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.RemovalPolicy getRemovalPolicy() {
return null;
}
/**
* The name of an IAM role that AWS Organizations automatically preconfigures in the new member account.
*
* This role trusts the management account, allowing users in the management account to assume the role, as permitted by the management account administrator. The role has administrator permissions in the new member account.
*
* If you don't specify this parameter, the role name defaults to OrganizationAccountAccessRole.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getRoleName() {
return null;
}
/**
* @return a {@link Builder} of {@link AccountProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link AccountProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String accountName;
java.lang.String email;
com.pepperize.cdk.organizations.IamUserAccessToBilling iamUserAccessToBilling;
java.lang.Boolean importOnDuplicate;
com.pepperize.cdk.organizations.IParent parent;
software.amazon.awscdk.RemovalPolicy removalPolicy;
java.lang.String roleName;
/**
* Sets the value of {@link AccountProps#getAccountName}
* @param accountName The friendly name of the member account. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder accountName(java.lang.String accountName) {
this.accountName = accountName;
return this;
}
/**
* Sets the value of {@link AccountProps#getEmail}
* @param email The email address of the owner to assign to the new member account. This parameter is required.
* This email address must not already be associated with another AWS account. You must use a valid email address to complete account creation. You can't access the root user of the account or remove an account that was created with an invalid email address.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder email(java.lang.String email) {
this.email = email;
return this;
}
/**
* Sets the value of {@link AccountProps#getIamUserAccessToBilling}
* @param iamUserAccessToBilling If set to ALLOW , the new account enables IAM users to access account billing information if they have the required permissions.
* If set to DENY , only the root user of the new account can access account billing information.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder iamUserAccessToBilling(com.pepperize.cdk.organizations.IamUserAccessToBilling iamUserAccessToBilling) {
this.iamUserAccessToBilling = iamUserAccessToBilling;
return this;
}
/**
* Sets the value of {@link AccountProps#getImportOnDuplicate}
* @param importOnDuplicate Whether to import, if a duplicate account with same name and email already exists.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder importOnDuplicate(java.lang.Boolean importOnDuplicate) {
this.importOnDuplicate = importOnDuplicate;
return this;
}
/**
* Sets the value of {@link AccountProps#getParent}
* @param parent The parent root or OU that you want to create the new Account in.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parent(com.pepperize.cdk.organizations.IParent parent) {
this.parent = parent;
return this;
}
/**
* Sets the value of {@link AccountProps#getRemovalPolicy}
* @param removalPolicy If set to RemovalPolicy.DESTROY, the account will be moved to the root.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder removalPolicy(software.amazon.awscdk.RemovalPolicy removalPolicy) {
this.removalPolicy = removalPolicy;
return this;
}
/**
* Sets the value of {@link AccountProps#getRoleName}
* @param roleName The name of an IAM role that AWS Organizations automatically preconfigures in the new member account.
* This role trusts the management account, allowing users in the management account to assume the role, as permitted by the management account administrator. The role has administrator permissions in the new member account.
*
* If you don't specify this parameter, the role name defaults to OrganizationAccountAccessRole.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder roleName(java.lang.String roleName) {
this.roleName = roleName;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link AccountProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public AccountProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link AccountProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements AccountProps {
private final java.lang.String accountName;
private final java.lang.String email;
private final com.pepperize.cdk.organizations.IamUserAccessToBilling iamUserAccessToBilling;
private final java.lang.Boolean importOnDuplicate;
private final com.pepperize.cdk.organizations.IParent parent;
private final software.amazon.awscdk.RemovalPolicy removalPolicy;
private final java.lang.String roleName;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.accountName = software.amazon.jsii.Kernel.get(this, "accountName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.email = software.amazon.jsii.Kernel.get(this, "email", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.iamUserAccessToBilling = software.amazon.jsii.Kernel.get(this, "iamUserAccessToBilling", software.amazon.jsii.NativeType.forClass(com.pepperize.cdk.organizations.IamUserAccessToBilling.class));
this.importOnDuplicate = software.amazon.jsii.Kernel.get(this, "importOnDuplicate", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.parent = software.amazon.jsii.Kernel.get(this, "parent", software.amazon.jsii.NativeType.forClass(com.pepperize.cdk.organizations.IParent.class));
this.removalPolicy = software.amazon.jsii.Kernel.get(this, "removalPolicy", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.RemovalPolicy.class));
this.roleName = software.amazon.jsii.Kernel.get(this, "roleName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.accountName = java.util.Objects.requireNonNull(builder.accountName, "accountName is required");
this.email = java.util.Objects.requireNonNull(builder.email, "email is required");
this.iamUserAccessToBilling = builder.iamUserAccessToBilling;
this.importOnDuplicate = builder.importOnDuplicate;
this.parent = builder.parent;
this.removalPolicy = builder.removalPolicy;
this.roleName = builder.roleName;
}
@Override
public final java.lang.String getAccountName() {
return this.accountName;
}
@Override
public final java.lang.String getEmail() {
return this.email;
}
@Override
public final com.pepperize.cdk.organizations.IamUserAccessToBilling getIamUserAccessToBilling() {
return this.iamUserAccessToBilling;
}
@Override
public final java.lang.Boolean getImportOnDuplicate() {
return this.importOnDuplicate;
}
@Override
public final com.pepperize.cdk.organizations.IParent getParent() {
return this.parent;
}
@Override
public final software.amazon.awscdk.RemovalPolicy getRemovalPolicy() {
return this.removalPolicy;
}
@Override
public final java.lang.String getRoleName() {
return this.roleName;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("accountName", om.valueToTree(this.getAccountName()));
data.set("email", om.valueToTree(this.getEmail()));
if (this.getIamUserAccessToBilling() != null) {
data.set("iamUserAccessToBilling", om.valueToTree(this.getIamUserAccessToBilling()));
}
if (this.getImportOnDuplicate() != null) {
data.set("importOnDuplicate", om.valueToTree(this.getImportOnDuplicate()));
}
if (this.getParent() != null) {
data.set("parent", om.valueToTree(this.getParent()));
}
if (this.getRemovalPolicy() != null) {
data.set("removalPolicy", om.valueToTree(this.getRemovalPolicy()));
}
if (this.getRoleName() != null) {
data.set("roleName", om.valueToTree(this.getRoleName()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@pepperize/cdk-organizations.AccountProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AccountProps.Jsii$Proxy that = (AccountProps.Jsii$Proxy) o;
if (!accountName.equals(that.accountName)) return false;
if (!email.equals(that.email)) return false;
if (this.iamUserAccessToBilling != null ? !this.iamUserAccessToBilling.equals(that.iamUserAccessToBilling) : that.iamUserAccessToBilling != null) return false;
if (this.importOnDuplicate != null ? !this.importOnDuplicate.equals(that.importOnDuplicate) : that.importOnDuplicate != null) return false;
if (this.parent != null ? !this.parent.equals(that.parent) : that.parent != null) return false;
if (this.removalPolicy != null ? !this.removalPolicy.equals(that.removalPolicy) : that.removalPolicy != null) return false;
return this.roleName != null ? this.roleName.equals(that.roleName) : that.roleName == null;
}
@Override
public final int hashCode() {
int result = this.accountName.hashCode();
result = 31 * result + (this.email.hashCode());
result = 31 * result + (this.iamUserAccessToBilling != null ? this.iamUserAccessToBilling.hashCode() : 0);
result = 31 * result + (this.importOnDuplicate != null ? this.importOnDuplicate.hashCode() : 0);
result = 31 * result + (this.parent != null ? this.parent.hashCode() : 0);
result = 31 * result + (this.removalPolicy != null ? this.removalPolicy.hashCode() : 0);
result = 31 * result + (this.roleName != null ? this.roleName.hashCode() : 0);
return result;
}
}
}