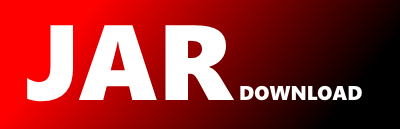
com.pepperize.cdk.organizations.IOrganizationalUnit Maven / Gradle / Ivy
Show all versions of cdk-organizations Show documentation
package com.pepperize.cdk.organizations;
/**
* A container for accounts within a root.
*
* An OU also can contain other OUs, enabling you to create a hierarchy that resembles an upside-down tree, with a root at the top and branches of OUs that reach down, ending in accounts that are the leaves of the tree. When you attach a policy to one of the nodes in the hierarchy, it flows down and affects all the branches (OUs) and leaves (accounts) beneath it. An OU can have exactly one parent, and currently each account can be a member of exactly one OU.
*
* You must first move all accounts out of the OU and any child OUs, and then you can delete the child OUs.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.98.0 (build 00b106d)", date = "2024-05-17T01:18:02.613Z")
@software.amazon.jsii.Jsii(module = com.pepperize.cdk.organizations.$Module.class, fqn = "@pepperize/cdk-organizations.IOrganizationalUnit")
@software.amazon.jsii.Jsii.Proxy(IOrganizationalUnit.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface IOrganizationalUnit extends software.amazon.jsii.JsiiSerializable, com.pepperize.cdk.organizations.IPolicyAttachmentTarget, com.pepperize.cdk.organizations.IParent, com.pepperize.cdk.organizations.IChild, software.constructs.IConstruct {
/**
* The Amazon Resource Name (ARN) of this OU.
*
* For more information about ARNs in Organizations, see ARN Formats Supported by Organizations in the AWS Service Authorization Reference.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getOrganizationalUnitArn();
/**
* The unique identifier (ID) associated with this OU.
*
* The regex pattern for an organizational unit ID string requires "ou-" followed by from 4 to 32 lowercase letters or digits (the ID of the root that contains the OU). This string is followed by a second "-" dash and from 8 to 32 additional lowercase letters or digits.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getOrganizationalUnitId();
/**
* The friendly name of this OU.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getOrganizationalUnitName();
/**
* The tree node.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.constructs.Node getNode();
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements com.pepperize.cdk.organizations.IOrganizationalUnit.Jsii$Default {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
/**
* The tree node.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final @org.jetbrains.annotations.NotNull software.constructs.Node getNode() {
return software.amazon.jsii.Kernel.get(this, "node", software.amazon.jsii.NativeType.forClass(software.constructs.Node.class));
}
/**
* The Amazon Resource Name (ARN) of this OU.
*
* For more information about ARNs in Organizations, see ARN Formats Supported by Organizations in the AWS Service Authorization Reference.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final @org.jetbrains.annotations.NotNull java.lang.String getOrganizationalUnitArn() {
return software.amazon.jsii.Kernel.get(this, "organizationalUnitArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The unique identifier (ID) associated with this OU.
*
* The regex pattern for an organizational unit ID string requires "ou-" followed by from 4 to 32 lowercase letters or digits (the ID of the root that contains the OU). This string is followed by a second "-" dash and from 8 to 32 additional lowercase letters or digits.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final @org.jetbrains.annotations.NotNull java.lang.String getOrganizationalUnitId() {
return software.amazon.jsii.Kernel.get(this, "organizationalUnitId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The friendly name of this OU.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final @org.jetbrains.annotations.NotNull java.lang.String getOrganizationalUnitName() {
return software.amazon.jsii.Kernel.get(this, "organizationalUnitName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The unique identifier (ID) of the parent root, organizational unit (OU), account, or policy that you want to create the new OU in.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public final @org.jetbrains.annotations.NotNull java.lang.String identifier() {
return software.amazon.jsii.Kernel.call(this, "identifier", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
}
/**
* Internal default implementation for {@link IOrganizationalUnit}.
*/
@software.amazon.jsii.Internal
interface Jsii$Default extends IOrganizationalUnit, com.pepperize.cdk.organizations.IChild.Jsii$Default, com.pepperize.cdk.organizations.IParent.Jsii$Default, com.pepperize.cdk.organizations.IPolicyAttachmentTarget.Jsii$Default, software.constructs.IConstruct.Jsii$Default {
/**
* The tree node.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.NotNull software.constructs.Node getNode() {
return software.amazon.jsii.Kernel.get(this, "node", software.amazon.jsii.NativeType.forClass(software.constructs.Node.class));
}
/**
* The Amazon Resource Name (ARN) of this OU.
*
* For more information about ARNs in Organizations, see ARN Formats Supported by Organizations in the AWS Service Authorization Reference.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.NotNull java.lang.String getOrganizationalUnitArn() {
return software.amazon.jsii.Kernel.get(this, "organizationalUnitArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The unique identifier (ID) associated with this OU.
*
* The regex pattern for an organizational unit ID string requires "ou-" followed by from 4 to 32 lowercase letters or digits (the ID of the root that contains the OU). This string is followed by a second "-" dash and from 8 to 32 additional lowercase letters or digits.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.NotNull java.lang.String getOrganizationalUnitId() {
return software.amazon.jsii.Kernel.get(this, "organizationalUnitId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The friendly name of this OU.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.NotNull java.lang.String getOrganizationalUnitName() {
return software.amazon.jsii.Kernel.get(this, "organizationalUnitName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The unique identifier (ID) of the parent root, organizational unit (OU), account, or policy that you want to create the new OU in.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
default @org.jetbrains.annotations.NotNull java.lang.String identifier() {
return software.amazon.jsii.Kernel.call(this, "identifier", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
}
}