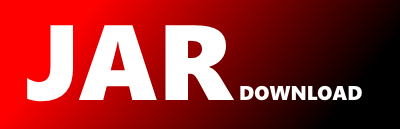
com.pepperize.cdk.organizations.Organization Maven / Gradle / Ivy
Show all versions of cdk-organizations Show documentation
package com.pepperize.cdk.organizations;
/**
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.98.0 (build 00b106d)", date = "2024-05-17T01:18:02.655Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = com.pepperize.cdk.organizations.$Module.class, fqn = "@pepperize/cdk-organizations.Organization")
public class Organization extends software.constructs.Construct implements com.pepperize.cdk.organizations.IOrganization {
protected Organization(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected Organization(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Organization(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.Nullable com.pepperize.cdk.organizations.OrganizationProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), props });
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Organization(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required") });
}
/**
* Describe the organization that the current account belongs to.
*
* @see https://docs.aws.amazon.com/organizations/latest/APIReference/API_DescribeOrganization.html
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull com.pepperize.cdk.organizations.IOrganization of(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(com.pepperize.cdk.organizations.Organization.class, "of", software.amazon.jsii.NativeType.forClass(com.pepperize.cdk.organizations.IOrganization.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required") });
}
/**
* Attach a policy.
*
* Before you can attach the policy, you must enable that policy type for use. You can use policies when you have all features enabled.
*
* @see https://docs.aws.amazon.com/organizations/latest/userguide/orgs_manage_policies.html
* @param policy This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void attachPolicy(final @org.jetbrains.annotations.NotNull com.pepperize.cdk.organizations.IPolicy policy) {
software.amazon.jsii.Kernel.call(this, "attachPolicy", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(policy, "policy is required") });
}
/**
* Enables trusted access for a supported AWS service (trusted service), which performs tasks in your organization and its accounts on your behalf.
*
* @see https://docs.aws.amazon.com/organizations/latest/userguide/orgs_integrate_services_list.html
* @param servicePrincipal The supported AWS service that you specify. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void enableAwsServiceAccess(final @org.jetbrains.annotations.NotNull java.lang.String servicePrincipal) {
software.amazon.jsii.Kernel.call(this, "enableAwsServiceAccess", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(servicePrincipal, "servicePrincipal is required") });
}
/**
* Enables policy types in the following two broad categories: Authorization policies and Management policies.
*
* @see https://docs.aws.amazon.com/organizations/latest/userguide/orgs_manage_policies.html#orgs-policy-types
* @param policyType : the type of the policy that you specify. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void enablePolicyType(final @org.jetbrains.annotations.NotNull com.pepperize.cdk.organizations.PolicyType policyType) {
software.amazon.jsii.Kernel.call(this, "enablePolicyType", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(policyType, "policyType is required") });
}
/**
* Specifies the functionality that currently is available to the organization.
*
* If set to "ALL", then all features are enabled and policies can be applied to accounts in the organization. If set to "CONSOLIDATED_BILLING", then only consolidated billing functionality is available.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull com.pepperize.cdk.organizations.FeatureSet getFeatureSet() {
return software.amazon.jsii.Kernel.get(this, "featureSet", software.amazon.jsii.NativeType.forClass(com.pepperize.cdk.organizations.FeatureSet.class));
}
/**
* The Amazon Resource Name (ARN) of the account that is designated as the management account for the organization.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getManagementAccountArn() {
return software.amazon.jsii.Kernel.get(this, "managementAccountArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The email address that is associated with the AWS account that is designated as the management account for the organization.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getManagementAccountEmail() {
return software.amazon.jsii.Kernel.get(this, "managementAccountEmail", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The unique identifier (ID) of the management account of an organization.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getManagementAccountId() {
return software.amazon.jsii.Kernel.get(this, "managementAccountId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The Amazon Resource Name (ARN) of an organization.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getOrganizationArn() {
return software.amazon.jsii.Kernel.get(this, "organizationArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The unique identifier (ID) of an organization.
*
* The regex pattern for an organization ID string requires "o-" followed by from 10 to 32 lowercase letters or digits.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getOrganizationId() {
return software.amazon.jsii.Kernel.get(this, "organizationId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The principal that represents this AWS Organization.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IPrincipal getPrincipal() {
return software.amazon.jsii.Kernel.get(this, "principal", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IPrincipal.class));
}
/**
* The root of the current organization, which is automatically created.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull com.pepperize.cdk.organizations.Root getRoot() {
return software.amazon.jsii.Kernel.get(this, "root", software.amazon.jsii.NativeType.forClass(com.pepperize.cdk.organizations.Root.class));
}
/**
* A fluent builder for {@link com.pepperize.cdk.organizations.Organization}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private com.pepperize.cdk.organizations.OrganizationProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
}
/**
* Enabling features in your organization.
*
* Default: ALL
*
* @return {@code this}
* @see https://docs.aws.amazon.com/organizations/latest/userguide/orgs_manage_org_support-all-features.html
* @param featureSet Enabling features in your organization. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder featureSet(final com.pepperize.cdk.organizations.FeatureSet featureSet) {
this.props().featureSet(featureSet);
return this;
}
/**
* @return a newly built instance of {@link com.pepperize.cdk.organizations.Organization}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public com.pepperize.cdk.organizations.Organization build() {
return new com.pepperize.cdk.organizations.Organization(
this.scope,
this.id,
this.props != null ? this.props.build() : null
);
}
private com.pepperize.cdk.organizations.OrganizationProps.Builder props() {
if (this.props == null) {
this.props = new com.pepperize.cdk.organizations.OrganizationProps.Builder();
}
return this.props;
}
}
}