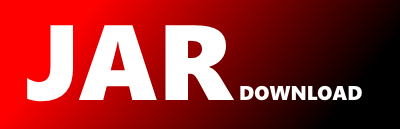
com.perforce.p4java.impl.mapbased.rpc.CommandEnv Maven / Gradle / Ivy
Show all versions of p4java Show documentation
/*
* Copyright 2009 Perforce Software Inc., All Rights Reserved.
*/
package com.perforce.p4java.impl.mapbased.rpc;
import com.perforce.p4java.Log;
import com.perforce.p4java.exception.P4JavaException;
import com.perforce.p4java.impl.mapbased.rpc.connection.RpcConnection;
import com.perforce.p4java.impl.mapbased.rpc.func.RpcFunctionMapKey;
import com.perforce.p4java.impl.mapbased.rpc.func.proto.ProtocolCommand;
import com.perforce.p4java.impl.mapbased.rpc.packet.helper.RpcPacketFieldRule;
import com.perforce.p4java.server.callback.IFilterCallback;
import com.perforce.p4java.server.callback.IParallelCallback;
import com.perforce.p4java.server.callback.IProgressCallback;
import com.perforce.p4java.server.callback.IStreamingCallback;
import java.io.File;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Used to package up the Perforce function environment for a single
* Perforce command across multiple RPC function calls.
*
* In particular, we need to keep things like file handles,
* arbitrary RPC function arguments, etc., around for use during
* complex long-running commands that span many dispatch calls
* in loop or duplex mode, etc., in response to single
* user commands like 'sync'.
*
* Note that this is in distinction to a) the command's external
* environment (in the ExternalEnv class), and b) the command's
* individual function environments,
*/
public class CommandEnv {
/**
* Max number of live handlers per command cycle.
* Value copied straight from the C++ API.
*/
public static final int MAX_HANDLERS = 10;
/**
* Sequence used by operating system to separate lines in text files.
* Default to "\n" if it is not available.
*/
public static final String LINE_SEPARATOR = System.getProperty("line.separator", "\n");
/**
* P4Java's version of the notorious handler class
* in the C++ API. Basically used (and abused) in
* much the same way, mostly (at least) until I can
* work out a better way to do things, at which
* point it's likely to be factored out elsewhere.
*/
public class RpcHandler {
private String name = null;
private String type = null;
private boolean error = false;
private File file = null;
private Map map = null;
public RpcHandler(String name, boolean error, File file) {
this.name = name;
this.error = error;
this.file = file;
this.map = new HashMap();
}
public String getName() {
return this.name;
}
public void setName(String name) {
this.name = name;
}
public String getType() {
return this.type;
}
public void setType(String type) {
this.type = type;
}
public boolean isError() {
return this.error;
}
public void setError(boolean error) {
this.error = error;
}
public File getFile() {
return this.file;
}
public void setFile(File file) {
this.file = file;
}
public Map getMap() {
return this.map;
}
public void setMap(Map map) {
this.map = map;
}
}
/**
* The parent server object
*/
private RpcServer server = null;
/**
* The current user function that started this all...
*/
private RpcCmdSpec cmdSpec = null;
/**
* The result maps that will ultimately be passed back to the user levels.
*/
private List