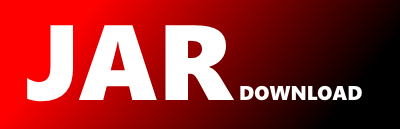
personthecat.catlib.client.gui.LibMenu Maven / Gradle / Ivy
package personthecat.catlib.client.gui;
import com.mojang.blaze3d.platform.GlStateManager;
import com.mojang.blaze3d.platform.Window;
import com.mojang.blaze3d.systems.RenderSystem;
import com.mojang.blaze3d.vertex.*;
import net.minecraft.client.Minecraft;
import net.minecraft.client.gui.Font;
import net.minecraft.client.gui.GuiComponent;
import net.minecraft.client.gui.components.Button;
import net.minecraft.client.gui.screens.Screen;
import net.minecraft.client.renderer.GameRenderer;
import net.minecraft.network.chat.CommonComponents;
import net.minecraft.network.chat.Component;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public class LibMenu extends Screen {
protected static final float G = 32.0F;
protected static final int Y0 = 35;
protected static final int Y1 = 50;
@Nullable protected Screen parent;
protected Font f_96547_;
protected Button previous;
protected Button cancel;
protected Button next;
protected LibMenu(@Nullable Screen parent, Component title) {
super(title);
this.f_96547_ = Minecraft.m_91087_().f_91062_;
this.parent = parent;
}
@Override
protected void m_7856_() {
this.m_169413_();
this.m_6702_().clear();
this.previous = new Button(this.f_96543_ / 2 - 60 - 120 - 10, this.f_96544_ - 35, 120, 20,
CommonComponents.f_130660_, b -> this.onPrevious());
this.cancel = new Button(this.f_96543_ / 2 - 60, this.f_96544_ - 35, 120, 20,
CommonComponents.f_130656_, b -> this.m_7379_());
this.next = new Button(this.f_96543_ / 2 - 60 + 120 + 10, this.f_96544_ - 35, 120, 20,
CommonComponents.f_130659_, b -> this.onNext());
this.m_142416_(this.previous);
this.m_142416_(this.cancel);
this.m_142416_(this.next);
}
public LibMenu loadImmediately() {
final Minecraft minecraft = Minecraft.m_91087_();
final Window window = minecraft.m_91268_();
this.m_6575_(minecraft, window.m_85445_(), window.m_85446_());
return this;
}
boolean menu = true;
@Override
public void m_6305_(@NotNull PoseStack stack, int x, int y, float partial) {
this.m_7333_(stack);
final Tesselator tx = Tesselator.m_85913_();
final BufferBuilder builder = tx.m_85915_();
RenderSystem.m_157427_(GameRenderer::m_172820_);
this.renderBackground(tx, builder);
if (menu) {
this.renderMenu(stack, x, y, partial);
}
this.renderTopAndBottom(tx, builder);
this.renderDetails(stack, x, y, partial);
RenderSystem.m_69493_();
RenderSystem.m_69461_();
}
boolean bgConfig = true, bg = true;
protected void renderBackground(Tesselator tx, BufferBuilder builder) {
if (bgConfig) {
RenderSystem.m_157456_(0, GuiComponent.f_93096_);
RenderSystem.m_157429_(1.0F, 1.0F, 1.0F, 1.0F);
}
if (bg) {
builder.m_166779_(VertexFormat.Mode.QUADS, DefaultVertexFormat.f_85819_);
builder.m_5483_(0.0, this.f_96544_ - Y1, 0.0D).m_7421_(0.0F, (this.f_96544_ - Y1) / G).m_6122_(32, 32, 32, 255).m_5752_();
builder.m_5483_(this.f_96543_, this.f_96544_ - Y1, 0.0D).m_7421_((float) this.f_96543_ / G, (float) (this.f_96544_ - Y1) / G).m_6122_(32, 32, 32, 255).m_5752_();
builder.m_5483_(this.f_96543_, Y0, 0.0D).m_7421_(this.f_96543_ / G, (float) Y0 / G).m_6122_(32, 32, 32, 255).m_5752_();
builder.m_5483_(0.0, Y0, 0.0D).m_7421_(0.0F, Y0 / G).m_6122_(32, 32, 32, 255).m_5752_();
tx.m_85914_();
}
}
protected void renderMenu(PoseStack stack, int x, int y, float partial) {}
boolean topConfig = true,
top = true,
bottomConfig = true,
bottom = true,
forceBlack = true;
protected void renderTopAndBottom(Tesselator tx, BufferBuilder builder) {
if (topConfig) {
RenderSystem.m_157427_(GameRenderer::m_172820_);
RenderSystem.m_157456_(0, GuiComponent.f_93096_);
RenderSystem.m_69482_();
RenderSystem.m_69456_(519); // GL_ALWAYS
}
if (top) { // top and bottom
builder.m_166779_(VertexFormat.Mode.QUADS, DefaultVertexFormat.f_85819_);
builder.m_5483_(0.0, Y0, -100.0D).m_7421_(0.0F, Y0 / G).m_6122_(64, 64, 64, 255).m_5752_();
builder.m_5483_(this.f_96543_, Y0, -100.0D).m_7421_((float)this.f_96543_ / G, Y0 / G).m_6122_(64, 64, 64, 255).m_5752_();
builder.m_5483_(this.f_96543_, 0.0D, -100.0D).m_7421_((float)this.f_96543_ / G, 0.0F).m_6122_(64, 64, 64, 255).m_5752_();
builder.m_5483_(0.0, 0.0D, -100.0D).m_7421_(0.0F, 0.0F).m_6122_(64, 64, 64, 255).m_5752_();
builder.m_5483_(0.0, this.f_96544_, -100.0D).m_7421_(0.0F, (float)this.f_96544_ / G).m_6122_(64, 64, 64, 255).m_5752_();
builder.m_5483_(this.f_96543_, this.f_96544_, -100.0D).m_7421_((float)this.f_96543_ / G, (float)this.f_96544_ / G).m_6122_(64, 64, 64, 255).m_5752_();
builder.m_5483_(this.f_96543_, this.f_96544_ - Y1, -100.0D).m_7421_((float)this.f_96543_ / G, (this.f_96544_ - Y1) / G).m_6122_(64, 64, 64, 255).m_5752_();
builder.m_5483_(0.0, this.f_96544_ - Y1, -100.0D).m_7421_(0.0F, (this.f_96544_ - Y1) / G).m_6122_(64, 64, 64, 255).m_5752_();
tx.m_85914_();
}
if (bottomConfig) {
RenderSystem.m_69456_(515); // GL_EQUAL
RenderSystem.m_69465_();
RenderSystem.m_69478_();
RenderSystem.m_69416_(GlStateManager.SourceFactor.SRC_ALPHA, GlStateManager.DestFactor.ONE_MINUS_SRC_ALPHA, GlStateManager.SourceFactor.ZERO, GlStateManager.DestFactor.ONE);
RenderSystem.m_69472_();
RenderSystem.m_157427_(GameRenderer::m_172811_);
}
if (forceBlack) {
RenderSystem.m_157429_(0, 0, 0, 1);
}
if (bottom) { // shadows
builder.m_166779_(VertexFormat.Mode.QUADS, DefaultVertexFormat.f_85815_);
builder.m_5483_(0.0, Y0 + 4, 0.0D).m_7421_(0.0F, 1.0F).m_6122_(0, 0, 0, 0).m_5752_();
builder.m_5483_(this.f_96543_, Y0 + 4, 0.0D).m_7421_(1.0F, 1.0F).m_6122_(0, 0, 0, 0).m_5752_();
builder.m_5483_(this.f_96543_, Y0, 0.0D).m_7421_(1.0F, 0.0F).m_6122_(0, 0, 0, 255).m_5752_();
builder.m_5483_(0.0, Y0, 0.0D).m_7421_(0.0F, 0.0F).m_6122_(0, 0, 0, 255).m_5752_();
builder.m_5483_(0.0, this.f_96544_ - Y1, 0.0D).m_7421_(0.0F, 1.0F).m_6122_(0, 0, 0, 255).m_5752_();
builder.m_5483_(this.f_96543_, this.f_96544_ - Y1, 0.0D).m_7421_(1.0F, 1.0F).m_6122_(0, 0, 0, 255).m_5752_();
builder.m_5483_(this.f_96543_, this.f_96544_ - Y1 - 4, 0.0D).m_7421_(1.0F, 0.0F).m_6122_(0, 0, 0, 0).m_5752_();
builder.m_5483_(0.0, this.f_96544_ - Y1 - 4, 0.0D).m_7421_(0.0F, 0.0F).m_6122_(0, 0, 0, 0).m_5752_();
tx.m_85914_();
}
}
protected void renderDetails(PoseStack stack, int x, int y, float partial) {
m_93215_(stack, this.f_96547_, this.f_96539_, this.f_96543_ / 2, 20, 16777215);
super.m_6305_(stack, x, y, partial);
}
@Override
public void m_96602_(@NotNull PoseStack stack, @NotNull Component tooltip, int x, int y) {
this.m_96617_(stack, this.f_96547_.m_92923_(tooltip,this.f_96543_ / 2), x, y);
}
@Override
public void m_7379_() {
Minecraft.m_91087_().m_91152_(this.parent);
}
protected void onPrevious() {}
protected void onNext() {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy