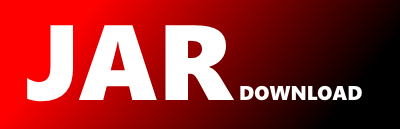
personthecat.catlib.event.registry.forge.RegistryAddedEventImpl Maven / Gradle / Ivy
package personthecat.catlib.event.registry.forge;
import lombok.extern.log4j.Log4j2;
import net.minecraft.core.Registry;
import net.minecraft.core.RegistryAccess;
import net.minecraft.resources.ResourceKey;
import personthecat.catlib.event.LibEvent;
import personthecat.catlib.event.registry.*;
import personthecat.catlib.registry.forge.ForgeRegistryHandle;
import personthecat.catlib.registry.RegistryHandle;
import personthecat.catlib.registry.RegistryUtils;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
@Log4j2
public class RegistryAddedEventImpl {
private static final Map, LibEvent>> DYNAMIC_EVENT_MAP = new ConcurrentHashMap<>();
@SuppressWarnings("unchecked")
public static LibEvent> get(final ResourceKey> key) {
final personthecat.catlib.registry.RegistryHandle handle = RegistryUtils.getHandle(key);
final RegistryEventAccessor accessor = handle instanceof ForgeRegistryHandle
? (RegistryEventAccessor) ((ForgeRegistryHandle>) handle).getRegistry()
: (RegistryEventAccessor) ((personthecat.catlib.registry.MojangRegistryHandle>) handle).getRegistry();
return accessor.getRegistryAddedEvent();
}
public static void withRetroactive(final ResourceKey> key, final RegistryAddedCallback f) {
get(key).register(f);
runRetroactively(key, f);
}
public static void withDynamic(final ResourceKey> key, final RegistryAddedCallback f) {
get(key).register(f);
runDynamically(key, f);
}
public static void exhaustive(final ResourceKey> key, final RegistryAddedCallback f) {
get(key).register(f);
runRetroactively(key, f);
runDynamically(key, f);
}
@SuppressWarnings({"unchecked", "rawtypes"})
public static void onRegistryAccess(final RegistryAccess registries) {
DYNAMIC_EVENT_MAP.forEach((key, event) -> {
final personthecat.catlib.registry.RegistryHandle
© 2015 - 2025 Weber Informatics LLC | Privacy Policy