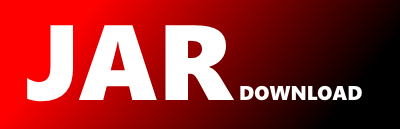
personthecat.catlib.exception.FormattedIOException Maven / Gradle / Ivy
package personthecat.catlib.exception;
import net.minecraft.ChatFormatting;
import net.minecraft.network.chat.*;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import personthecat.catlib.util.PathUtils;
import java.io.File;
public class FormattedIOException extends FormattedException {
final File root;
final File file;
final String relative;
final String msg;
public FormattedIOException(final File file, final Throwable cause) {
this(file.getParentFile(), file, cause);
}
public FormattedIOException(final File file, final Throwable cause, final String msg) {
this(file.getParentFile(), file, cause, msg);
}
public FormattedIOException(final File root, final File file, final Throwable cause) {
this(root, file, cause, createMsg(cause));
}
public FormattedIOException(final File root, final File file, final Throwable cause, final String msg) {
super(msg, cause);
this.root = root;
this.file = file;
this.relative = PathUtils.getRelativePath(root, file);
this.msg = msg;
}
@Override
public @NotNull String getCategory() {
return "catlib.errorMenu.io";
}
@Override
public @NotNull Component getDisplayMessage() {
return new TextComponent(this.relative);
}
@Override
public @Nullable Component getTooltip() {
return new TranslatableComponent(this.msg);
}
@Override
public @NotNull Component getTitleMessage() {
return new TranslatableComponent("catlib.errorText.fileError", this.relative);
}
@Override
public @NotNull Component getDetailMessage() {
final Component newLine = new TextComponent("\n");
final MutableComponent component = new TextComponent("");
final Style title = Style.f_131099_.m_131152_(ChatFormatting.UNDERLINE, ChatFormatting.BOLD);
component.m_7220_(new TranslatableComponent("catlib.errorText.fileDiagnostics").m_130948_(title));
component.m_7220_(newLine);
component.m_7220_(newLine);
final Component bullet = new TextComponent(" * ").m_130948_(Style.f_131099_.m_131136_(true));
final Component space = new TextComponent(" ");
final Style purple = Style.f_131099_.m_131140_(ChatFormatting.LIGHT_PURPLE);
final Style orange = Style.f_131099_.m_131140_(ChatFormatting.GOLD);
final Style green = Style.f_131099_.m_131140_(ChatFormatting.GREEN);
final Style blue = Style.f_131099_.m_131140_(ChatFormatting.DARK_BLUE);
final Style red = Style.f_131099_.m_131140_(ChatFormatting.RED);
try {
final boolean exists = this.file.exists();
component.m_7220_(bullet);
component.m_7220_(new TranslatableComponent("catlib.errorText.fileExists").m_130948_(purple));
component.m_7220_(space);
component.m_7220_(new TextComponent(String.valueOf(exists)).m_130948_(orange));
component.m_7220_(newLine);
final boolean dirExists = this.file.getParentFile().exists();
component.m_7220_(bullet);
component.m_7220_(new TranslatableComponent("catlib.errorText.directoryExists").m_130948_(purple));
component.m_7220_(space);
component.m_7220_(new TextComponent(String.valueOf(dirExists)).m_130948_(orange));
component.m_7220_(newLine);
final String type = PathUtils.extension(this.file);
component.m_7220_(bullet);
component.m_7220_(new TranslatableComponent("catlib.errorText.fileType").m_130948_(purple));
component.m_7220_(space);
component.m_7220_(new TextComponent(type).m_130948_(green));
component.m_7220_(newLine);
final long kb = this.file.length() / 1000;
component.m_7220_(bullet);
component.m_7220_(new TranslatableComponent("catlib.errorText.fileSize").m_130948_(purple));
component.m_7220_(space);
component.m_7220_(new TextComponent(kb + "kb").m_130948_(blue));
component.m_7220_(newLine);
} catch (final SecurityException ignored) {
component.m_7220_(new TranslatableComponent("catlib.errorText.ioNotAllowed").m_130948_(red));
component.m_7220_(newLine);
}
component.m_7220_(newLine);
component.m_7220_(new TranslatableComponent("catlib.errorText.stackTrace").m_130948_(title));
component.m_7220_(newLine);
component.m_7220_(newLine);
component.m_7220_(super.getDetailMessage());
return component;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy