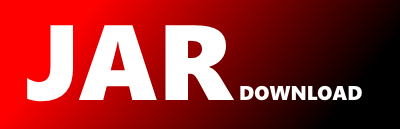
personthecat.catlib.serialization.codec.AutoFlatListCodec Maven / Gradle / Ivy
package personthecat.catlib.serialization.codec;
import com.google.common.collect.ImmutableList;
import com.mojang.datafixers.util.Pair;
import com.mojang.datafixers.util.Unit;
import com.mojang.serialization.*;
import org.apache.commons.lang3.mutable.MutableObject;
import java.util.List;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.stream.Stream;
public class AutoFlatListCodec implements Codec> {
private final Codec elementCodec;
public AutoFlatListCodec(final Codec elementCodec) {
this.elementCodec = elementCodec;
}
@Override
public DataResult encode(final List input, final DynamicOps ops, final T prefix) {
if (input.size() == 1) {
return this.elementCodec.encode(input.get(0), ops, prefix);
}
final ListBuilder builder = ops.listBuilder();
for (final A a : input) {
builder.add(this.elementCodec.encodeStart(ops, a));
}
return builder.build(prefix);
}
@Override
public DataResult, T>> decode(final DynamicOps ops, final T input) {
final ImmutableList.Builder read = ImmutableList.builder();
final Stream.Builder failed = Stream.builder();
final MutableObject> result = new MutableObject<>(DataResult.success(Unit.INSTANCE, Lifecycle.stable()));
this.decodeList(input, ops, read, failed, result);
final ImmutableList elements = read.build();
final T errors = ops.createList(failed.build());
final Pair, T> pair = Pair.of(elements, errors);
return result.getValue().map(unit -> pair).setPartial(pair);
}
private void decodeList(final T input, final DynamicOps ops, final ImmutableList.Builder read,
final Stream.Builder failed, final MutableObject> result) {
// Recurse until not a list.
final Optional>> listResult = ops.getList(input).result();
if (listResult.isPresent()) {
listResult.get().accept(t -> this.decodeList(t, ops, read, failed, result));
} else {
final DataResult> element = this.elementCodec.decode(ops, input);
element.error().ifPresent(e -> failed.add(input));
result.setValue(result.getValue().apply2stable((r, v) -> {
read.add(v.getFirst());
return r;
}, element));
}
}
@Override
public String toString() {
return "AutoFlatListCodec[" + this.elementCodec + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy