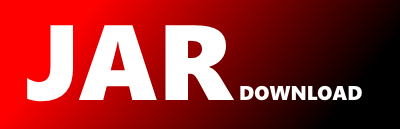
personthecat.catlib.serialization.codec.SimpleEitherCodec Maven / Gradle / Ivy
package personthecat.catlib.serialization.codec;
import com.mojang.datafixers.util.Pair;
import com.mojang.serialization.Codec;
import com.mojang.serialization.DataResult;
import com.mojang.serialization.DynamicOps;
import com.mojang.serialization.Encoder;
import java.util.function.Function;
@SuppressWarnings("unused")
public class SimpleEitherCodec implements Codec {
private final Codec extends A> first;
private final Codec extends A> second;
private final Function> encoder;
public SimpleEitherCodec(final Codec extends A> first, final Codec extends A> second) {
this(first, second, wrapDowncast(a -> first));
}
public SimpleEitherCodec(
final Codec extends A> first, final Codec extends A> second, final Function> encoder) {
this.first = first;
this.second = second;
this.encoder = encoder;
}
public SimpleEitherCodec withEncoder(final Encoder extends A> encoder) {
return this.withEncoder(a -> encoder);
}
public SimpleEitherCodec withEncoder(final Function> encoder) {
return new SimpleEitherCodec<>(this.first, this.second, wrapDowncast(encoder));
}
@SuppressWarnings("unchecked")
private static Function> wrapDowncast(final Function> encoder) {
return a -> (Encoder) encoder.apply(a);
}
@Override
@SuppressWarnings("OptionalGetWithoutIsPresent")
public DataResult> decode(final DynamicOps ops, final T input) {
final DataResult> r1 = downcast(this.first.decode(ops, input));
if (r1.result().isPresent()) {
return r1;
}
final DataResult> r2 = downcast(this.second.decode(ops, input));
if (r2.result().isPresent()) {
return r2;
}
final String m1 = r1.error().get().message();
final String m2 = r2.error().get().message();
return DataResult.error("Fix either: [\"" + m1 + "\",\"" + m2 + "\"]");
}
@SuppressWarnings("unchecked")
private static DataResult> downcast(final DataResult> pair) {
return (DataResult>) (Object) pair;
}
@Override
public DataResult encode(final A input, final DynamicOps ops, final T prefix) {
return this.encoder.apply(input).encode(input, ops, prefix);
}
@Override
public String toString() {
return "SimpleEither[" + this.first + " | " + this.second + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy