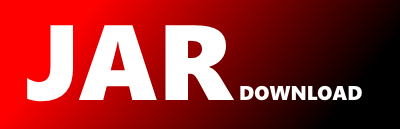
com.peterphi.std.guice.common.serviceprops.composite.GuiceConfig Maven / Gradle / Ivy
package com.peterphi.std.guice.common.serviceprops.composite;
import com.peterphi.std.io.PropertyFile;
import org.apache.commons.lang.StringUtils;
import org.apache.commons.lang.text.StrSubstitutor;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import java.util.function.Predicate;
public class GuiceConfig
{
private final Map properties = new HashMap<>();
private final Map overrides = new HashMap<>(0);
private final StrSubstitutor substitutor = new StrSubstitutor(new GuiceConfigVariableResolver(this));
public GuiceConfig()
{
}
public GuiceConfig(final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy