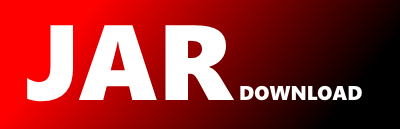
com.phloc.ebinterface.EEbInterfaceVersion Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of phloc-ebinterface Show documentation
Show all versions of phloc-ebinterface Show documentation
ebInterface wrapper library to easily read and write ebInterface documents
The newest version!
/**
* Copyright (C) 2006-2014 phloc systems
* http://www.phloc.com
* office[at]phloc[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.phloc.ebinterface;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.phloc.commons.annotations.Nonempty;
import com.phloc.commons.io.IReadableResource;
import com.phloc.commons.jaxb.utils.AbstractJAXBMarshaller;
import com.phloc.commons.string.StringHelper;
/**
* This enumeration encapsulates the supported ebInterface versions in a common
* way.
*
* @author Philip Helger
*/
public enum EEbInterfaceVersion
{
V30 (CEbInterface.EBINTERFACE_30_NS, CEbInterface.EBINTERFACE_30_XSLT)
{
@Override
public EbInterface30Marshaller createMarshaller ()
{
return new EbInterface30Marshaller ();
}
},
V302 (CEbInterface.EBINTERFACE_302_NS, CEbInterface.EBINTERFACE_302_XSLT)
{
@Override
public EbInterface302Marshaller createMarshaller ()
{
return new EbInterface302Marshaller ();
}
},
V40 (CEbInterface.EBINTERFACE_40_NS, CEbInterface.EBINTERFACE_40_XSLT)
{
@Override
public EbInterface40Marshaller createMarshaller ()
{
return new EbInterface40Marshaller ();
}
},
V41 (CEbInterface.EBINTERFACE_41_NS, CEbInterface.EBINTERFACE_41_XSLT)
{
@Override
public EbInterface41Marshaller createMarshaller ()
{
return new EbInterface41Marshaller ();
}
};
private final String m_sNamespaceURI;
private final IReadableResource m_aXSLTRes;
private EEbInterfaceVersion (@Nonnull @Nonempty final String sNamespaceURI, @Nonnull final IReadableResource aXSLTRes)
{
m_sNamespaceURI = sNamespaceURI;
m_aXSLTRes = aXSLTRes;
}
/**
* @return The namespace URI for this ebInterface version
*/
@Nonnull
@Nonempty
public String getNamespaceURI ()
{
return m_sNamespaceURI;
}
/**
* @return The resource to be used to visualize ebInterface invoices of this
* version
*/
@Nonnull
public IReadableResource getXSLTResource ()
{
return m_aXSLTRes;
}
/**
* @return A new marshaller that reads and writes the respective invoice
* objects. Each time this method is invoked, a new marshaller is
* created.
*/
@Nonnull
public abstract AbstractJAXBMarshaller > createMarshaller ();
/**
* Get the ebInterface version that matches the specified namespace.
*
* @param sNamespaceURI
* The namespace URI to resolve. May be null
.
* @return null
if the passed namespace URI could not be resolved
* to a supported ebInterface version.
*/
@Nullable
public static EEbInterfaceVersion getFromNamespaceURIOrNull (@Nullable final String sNamespaceURI)
{
if (StringHelper.hasText (sNamespaceURI))
for (final EEbInterfaceVersion eVersion : values ())
if (sNamespaceURI.equals (eVersion.getNamespaceURI ()))
return eVersion;
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy