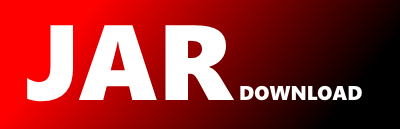
com.phloc.html.hc.IHCHasChildrenMutable Maven / Gradle / Ivy
package com.phloc.html.hc;
import java.util.Comparator;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.phloc.commons.annotations.DevelopersNote;
import com.phloc.commons.annotations.Nonempty;
import com.phloc.html.EHTMLElement;
import com.phloc.html.hc.impl.HCNodeList;
/**
* Base interface for HC nodes that have mutable children.
*
* @author Philip Helger
*/
public interface IHCHasChildrenMutable , CHILDTYPE extends IHCNode> extends IHCHasChildren
{
/**
* @param aNode
* Child to add. May be null
.
* @return this
*/
@Nonnull
THISTYPE addChild (@Nullable CHILDTYPE aNode);
/**
* @param nIndex
* The index to where the element should be inserted.
* @param aNode
* Child to add. May be null
.
* @return this
*/
@Nonnull
THISTYPE addChild (@Nonnegative int nIndex, @Nullable CHILDTYPE aNode);
/**
* Use {@link #addChild(IHCNode)} instead.
*
* @param aChild
* The child to add. May be null
.
* @return this
*/
@Deprecated
@DevelopersNote ("Use addChild instead")
THISTYPE addChildren (@Nullable CHILDTYPE aChild);
/**
* @param aChildren
* Children to add. May be null
.
* @return this
*/
@Nonnull
THISTYPE addChildren (@Nullable CHILDTYPE... aChildren);
/**
* @param aChildren
* Children to add. May be null
.
* @return this
*/
@Nonnull
THISTYPE addChildren (@Nullable Iterable extends CHILDTYPE> aChildren);
/**
* @param aChild
* Child to add. May be null
.
* @param
* The type to add. Needs to be a IHCNode or a sub class.
* @return the added child
*/
@Nullable
V addAndReturnChild (@Nullable V aChild);
/**
* @param nIndex
* The index where the element should be added. Always ≥ 0.
* @param aChild
* Child to add. May be null
.
* @param
* The type to add. Needs to be a IHCNode or a sub class.
* @return the added child
*/
@Nullable
V addAndReturnChild (@Nonnegative int nIndex, @Nullable V aChild);
/**
* Remove the child at the specified index.
*
* @param nIndex
* The index to use. Must be ≥ 0.
* @return this
*/
@Nonnull
THISTYPE removeChild (@Nonnegative int nIndex);
/**
* Remove the passed direct child object.
*
* @param aNode
* The node to be removed. May not be null
.
* @return this
*/
@Nonnull
THISTYPE removeChild (@Nullable CHILDTYPE aNode);
/**
* Remove all children of this object.
*
* @return this
*/
@Nonnull
THISTYPE removeAllChildren ();
/**
* Check if any of the specified elements is contained as a child of this.
*
* @param aElements
* The elements to check. May neither be null
nor empty.
* @return true
if at least one of the specified elements is
* contained, false
otherwise.
*/
boolean recursiveContainsChildWithTagName (@Nonnull @Nonempty EHTMLElement... aElements);
/**
* Sort all children with the passed comparator
*
* @param aComparator
* The comparator to be used. May not be null
.
* @return this
*/
@Nonnull
THISTYPE sortAllChildren (@Nonnull Comparator super CHILDTYPE> aComparator);
/**
* @return A new node list with all contained children. Never
* null
but maybe empty.
*/
@Nonnull
HCNodeList getAllChildrenAsNodeList ();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy