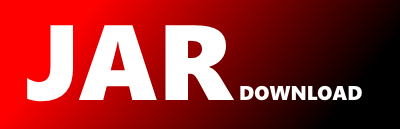
com.phloc.html.js.builder.jquery.IJQuerySelector Maven / Gradle / Ivy
/**
* Copyright (C) 2006-2015 phloc systems
* http://www.phloc.com
* office[at]phloc[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.phloc.html.js.builder.jquery;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nonnull;
import com.phloc.html.js.IJSCodeProvider;
import com.phloc.html.js.builder.IJSExpression;
/**
* A single jQuery selector
*
* @author Philip Helger
*/
public interface IJQuerySelector extends IJSCodeProvider
{
/**
* @return The contained expression that was used as the parameter to this
* selector. Never null
.
*/
@Nonnull
IJSExpression getExpression ();
/**
* Get chained with the passed selector
*
* @param aRhsSelector
* Other selector
* @return thisrhs
*/
@Nonnull
@CheckReturnValue
IJQuerySelector chain (@Nonnull IJQuerySelector aRhsSelector);
/**
* Make a multiple selector
*
* @param aRhsSelector
* Other selector
* @return this, rhs
*/
@Nonnull
@CheckReturnValue
IJQuerySelector multiple (@Nonnull IJQuerySelector aRhsSelector);
/**
* Make a child selector
*
* @param aRhsSelector
* Other selector
* @return this > rhs
*/
@Nonnull
@CheckReturnValue
IJQuerySelector child (@Nonnull IJQuerySelector aRhsSelector);
/**
* Make a descendant selector
*
* @param aRhsSelector
* Other selector
* @return this rhs
*/
@Nonnull
@CheckReturnValue
IJQuerySelector descendant (@Nonnull IJQuerySelector aRhsSelector);
/**
* Make a next adjacent selector
*
* @param aRhsSelector
* Other selector
* @return this + rhs
*/
@Nonnull
@CheckReturnValue
IJQuerySelector nextAdjacent (@Nonnull IJQuerySelector aRhsSelector);
/**
* Make a next siblings selector
*
* @param aRhsSelector
* Other selector
* @return this ~ rhs
*/
@Nonnull
@CheckReturnValue
IJQuerySelector nextSiblings (@Nonnull IJQuerySelector aRhsSelector);
/**
* Chain a animated selector
*
* @return this:animated
*/
@Nonnull
@CheckReturnValue
IJQuerySelector animated ();
/**
* Chain a button selector
*
* @return this:button
*/
@Nonnull
@CheckReturnValue
IJQuerySelector button ();
/**
* Chain a checkbox selector
*
* @return this:checkbox
*/
@Nonnull
@CheckReturnValue
IJQuerySelector checkbox ();
/**
* Chain a checked selector
*
* @return this:checked
*/
@Nonnull
@CheckReturnValue
IJQuerySelector checked ();
/**
* Chain a disabled selector
*
* @return this:disabled
*/
@Nonnull
@CheckReturnValue
IJQuerySelector disabled ();
/**
* Chain a empty selector
*
* @return this:empty
*/
@Nonnull
@CheckReturnValue
IJQuerySelector empty ();
/**
* Chain a enabled selector
*
* @return this:enabled
*/
@Nonnull
@CheckReturnValue
IJQuerySelector enabled ();
/**
* Chain a even selector
*
* @return this:even
*/
@Nonnull
@CheckReturnValue
IJQuerySelector even ();
/**
* Chain a file selector
*
* @return this:file
*/
@Nonnull
@CheckReturnValue
IJQuerySelector file ();
/**
* Chain a first selector
*
* @return this:first
*/
@Nonnull
@CheckReturnValue
IJQuerySelector first ();
/**
* Chain a first-child selector
*
* @return this:first-child
*/
@Nonnull
@CheckReturnValue
IJQuerySelector first_child ();
/**
* Chain a first-of-type selector
*
* @return this:first-of-type
*/
@Nonnull
@CheckReturnValue
IJQuerySelector first_of_type ();
/**
* Chain a focus selector
*
* @return this:focus
*/
@Nonnull
@CheckReturnValue
IJQuerySelector focus ();
/**
* Chain a header selector
*
* @return this:header
*/
@Nonnull
@CheckReturnValue
IJQuerySelector header ();
/**
* Chain a hidden selector
*
* @return this:hidden
*/
@Nonnull
@CheckReturnValue
IJQuerySelector hidden ();
/**
* Chain a image selector
*
* @return this:image
*/
@Nonnull
@CheckReturnValue
IJQuerySelector image ();
/**
* Chain a input selector
*
* @return this:input
*/
@Nonnull
@CheckReturnValue
IJQuerySelector input ();
/**
* Chain a last selector
*
* @return this:last
*/
@Nonnull
@CheckReturnValue
IJQuerySelector last ();
/**
* Chain a last-child selector
*
* @return this:last-child
*/
@Nonnull
@CheckReturnValue
IJQuerySelector last_child ();
/**
* Chain a last-of-type selector
*
* @return this:last-of-type
*/
@Nonnull
@CheckReturnValue
IJQuerySelector last_of_type ();
/**
* Chain a odd selector
*
* @return this:odd
*/
@Nonnull
@CheckReturnValue
IJQuerySelector odd ();
/**
* Chain a only-child selector
*
* @return this:only-child
*/
@Nonnull
@CheckReturnValue
IJQuerySelector only_child ();
/**
* Chain a only-of-type selector
*
* @return this:only-of-type
*/
@Nonnull
@CheckReturnValue
IJQuerySelector only_of_type ();
/**
* Chain a parent selector
*
* @return this:parent
*/
@Nonnull
@CheckReturnValue
IJQuerySelector parent ();
/**
* Chain a password selector
*
* @return this:password
*/
@Nonnull
@CheckReturnValue
IJQuerySelector password ();
/**
* Chain a radio selector
*
* @return this:radio
*/
@Nonnull
@CheckReturnValue
IJQuerySelector radio ();
/**
* Chain a reset selector
*
* @return this:reset
*/
@Nonnull
@CheckReturnValue
IJQuerySelector reset ();
/**
* Chain a root selector
*
* @return this:root
*/
@Nonnull
@CheckReturnValue
IJQuerySelector root ();
/**
* Chain a selected selector
*
* @return this:selected
*/
@Nonnull
@CheckReturnValue
IJQuerySelector selected ();
/**
* Chain a submit selector
*
* @return this:submit
*/
@Nonnull
@CheckReturnValue
IJQuerySelector submit ();
/**
* Chain a target selector
*
* @return this:target
*/
@Nonnull
@CheckReturnValue
IJQuerySelector target ();
/**
* Chain a text selector
*
* @return this:text
*/
@Nonnull
@CheckReturnValue
IJQuerySelector text ();
/**
* Chain a visible selector
*
* @return this:visible
*/
@Nonnull
@CheckReturnValue
IJQuerySelector visible ();
/**
* Create an invocation of this selector
*
* @return $(selectorString)
*/
@Nonnull
JQueryInvocation invoke ();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy