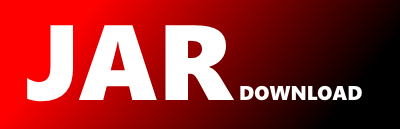
com.phloc.html.js.builder.jquery.JQuery Maven / Gradle / Ivy
/**
* Copyright (C) 2006-2015 phloc systems
* http://www.phloc.com
* office[at]phloc[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.phloc.html.js.builder.jquery;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.atomic.AtomicBoolean;
import javax.annotation.Nonnull;
import javax.annotation.concurrent.Immutable;
import org.w3c.dom.Node;
import com.phloc.commons.ValueEnforcer;
import com.phloc.commons.annotations.Nonempty;
import com.phloc.commons.annotations.PresentForCodeCoverage;
import com.phloc.commons.collections.pair.IReadonlyPair;
import com.phloc.commons.collections.pair.ReadonlyPair;
import com.phloc.commons.id.IHasID;
import com.phloc.commons.microdom.IMicroNode;
import com.phloc.commons.microdom.serialize.MicroWriter;
import com.phloc.commons.xml.serialize.XMLWriter;
import com.phloc.html.EHTMLElement;
import com.phloc.html.css.ICSSClassProvider;
import com.phloc.html.hc.IHCElement;
import com.phloc.html.hc.IHCNode;
import com.phloc.html.hc.conversion.HCSettings;
import com.phloc.html.js.IJSCodeProvider;
import com.phloc.html.js.builder.IJSExpression;
import com.phloc.html.js.builder.JSAnonymousFunction;
import com.phloc.html.js.builder.JSAssocArray;
import com.phloc.html.js.builder.JSExpr;
import com.phloc.html.js.builder.JSFunction;
import com.phloc.html.js.builder.html.JSHtml;
import com.phloc.json.IJSON;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
/**
* Wrapper around jQuery to allow for easy function calls
*
* @author Philip Helger
*/
@Immutable
@SuppressFBWarnings ("NM_METHOD_NAMING_CONVENTION")
public final class JQuery
{
public static final AtomicBoolean s_aUseDollar = new AtomicBoolean (true);
@SuppressWarnings ("unused")
@PresentForCodeCoverage
private static final JQuery s_aInstance = new JQuery ();
private JQuery ()
{}
/**
* Globally decide, whether to use "$" or "jQuery" to access jQuery function
*
* @param bUseDollar
* true
to use "$", false
to use "jQuery"
*/
public static void setUseDollarForJQuery (final boolean bUseDollar)
{
s_aUseDollar.set (bUseDollar);
}
/**
* @return true
if "$" is used, false
if "jQuery" is
* used for the global jQuery function
*/
public static boolean isUseDollarForJQuery ()
{
return s_aUseDollar.get ();
}
/**
* @return $
or jQuery
as a function
*/
@Nonnull
public static JSFunction jQueryFunction ()
{
return new JSFunction (isUseDollarForJQuery () ? "$" : "jQuery");
}
/**
* @return a {@link JQueryInvocation} with an arbitrary expression.
* $(expr)
*/
@Nonnull
public static JQueryInvocation jQuery (@Nonnull final IJSExpression aExpr)
{
return new JQueryInvocation (jQueryFunction ()).arg (aExpr);
}
/**
* @param sHTML
* HTML code to use
* @return a {@link JQueryInvocation} with an HTML String.
* $(html)
*/
@Nonnull
public static JQueryInvocation jQuery (@Nonnull final String sHTML)
{
return jQuery (JSExpr.lit (sHTML));
}
/**
* @param aHCNode
* HTML code to use
* @return a {@link JQueryInvocation} with an HTML String.
* $(html)
*/
@Nonnull
public static JQueryInvocation jQuery (@Nonnull final IHCNode aHCNode)
{
return jQuery (HCSettings.getAsHTMLStringWithoutNamespaces (aHCNode));
}
/**
* @return a {@link JQueryInvocation} with an HTML document element.
* $(document)
*/
@Nonnull
public static JQueryInvocation jQueryDocument ()
{
return jQuery (JSHtml.document ());
}
/**
* @return a {@link JQueryInvocation} with this
:
* $(this)
*/
@Nonnull
public static JQueryInvocation jQueryThis ()
{
return jQuery (JSExpr.THIS);
}
// Special cases
/**
* @param aArgs
* The array with the AJAX arguments
* @return $.ajax
*/
@Nonnull
public static JQueryInvocation ajax (@Nonnull final JSAssocArray aArgs)
{
return ajax ().arg (aArgs);
}
/**
* @param sEval
* JS code to be evaluated
* @return $.globalEval
*/
@Nonnull
public static JQueryInvocation globalEval (@Nonnull final String sEval)
{
return globalEval ().arg (sEval);
}
/**
* @param aEval
* JS code to be evaluated
* @return $.globalEval
*/
@Nonnull
public static JQueryInvocation globalEval (@Nonnull final IJSExpression aEval)
{
return globalEval ().arg (aEval);
}
@Nonnull
public static JQueryInvocation parseHTML (@Nonnull final Node aHTML)
{
return parseHTML (XMLWriter.getXMLString (aHTML));
}
@Nonnull
public static JQueryInvocation parseHTML (@Nonnull final IMicroNode aHTML)
{
return parseHTML (MicroWriter.getXMLString (aHTML));
}
@Nonnull
public static JQueryInvocation parseHTML (@Nonnull final IHCNode aHTML)
{
return parseHTML (HCSettings.getAsHTMLStringWithoutNamespaces (aHTML));
}
@Nonnull
public static JQueryInvocation parseHTML (@Nonnull final String sHTML)
{
return parseHTML (JSExpr.lit (sHTML));
}
@Nonnull
public static JQueryInvocation parseHTML (@Nonnull final IJSExpression aExpr)
{
return parseHTML ().arg (aExpr);
}
@Nonnull
public static JQueryInvocation parseJSON (@Nonnull final IJSON aJson)
{
return parseJSON (aJson.getJSONString ());
}
@Nonnull
public static JQueryInvocation parseJSON (@Nonnull final String sJSON)
{
return parseJSON (JSExpr.lit (sJSON));
}
@Nonnull
public static JQueryInvocation parseJSON (@Nonnull final IJSExpression aExpr)
{
return parseJSON ().arg (aExpr);
}
@Nonnull
public static JQueryInvocation parseXML (@Nonnull final Node aXML)
{
return parseXML (XMLWriter.getXMLString (aXML));
}
@Nonnull
public static JQueryInvocation parseXML (@Nonnull final IMicroNode aXML)
{
return parseXML (MicroWriter.getXMLString (aXML));
}
@Nonnull
public static JQueryInvocation parseXML (@Nonnull final String sXML)
{
return parseXML (JSExpr.lit (sXML));
}
@Nonnull
public static JQueryInvocation parseXML (@Nonnull final IJSExpression aExpr)
{
return parseXML ().arg (aExpr);
}
// selectors start here
/**
* Get the result of a jQuery selection
*
* @param sID
* The ID to be selected. May not be null
.
* @return A jQuery invocation with the passed ID: $('#id')
*/
@Nonnull
public static JQueryInvocation idRef (@Nonnull @Nonempty final String sID)
{
return JQuerySelector.id (sID).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param aID
* The ID to be selected. May not be null
.
* @return A jQuery invocation with the passed ID: $('#'+id)
*/
@Nonnull
public static JQueryInvocation idRef (@Nonnull final IJSExpression aID)
{
return JQuerySelector.id (aID).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param aIDProvider
* The provider that has the ID to be selected. May not be
* null
.
* @return A jQuery invocation with the passed ID: $('#id')
*/
@Nonnull
public static JQueryInvocation idRef (@Nonnull @Nonempty final IHasID aIDProvider)
{
return JQuerySelector.id (aIDProvider).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param aElement
* The element that has the ID to be selected. May not be
* null
.
* @return A jQuery invocation with the passed ID: $('#id')
*/
@Nonnull
public static JQueryInvocation idRef (@Nonnull @Nonempty final IHCElement > aElement)
{
return JQuerySelector.id (aElement).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param aIDs
* The IDs to be selected. May not be null
.
* @return A jQuery invocation with the passed IDs:
* $('#id1,#id2,#id3')
*/
@Nonnull
public static JQueryInvocation idRefMultiple (@Nonnull @Nonempty final String... aIDs)
{
ValueEnforcer.notEmpty (aIDs, "IDs");
final List aSelectors = new ArrayList ();
for (final String sID : aIDs)
aSelectors.add (JQuerySelector.id (sID));
return JQuerySelector.multiple (aSelectors).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param aIDs
* The IDs to be selected. May not be null
.
* @return A jQuery invocation with the passed IDs:
* $('#id1,#id2,#id3')
*/
@Nonnull
public static JQueryInvocation idRefMultiple (@Nonnull @Nonempty final IHasID ... aIDs)
{
ValueEnforcer.notEmptyNoNullValue (aIDs, "IDs");
final List aSelectors = new ArrayList ();
for (final IHasID aID : aIDs)
aSelectors.add (JQuerySelector.id (aID));
return JQuerySelector.multiple (aSelectors).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param aIDs
* The IDs to be selected. May not be null
.
* @return A jQuery invocation with the passed IDs:
* $('#id1,#id2,#id3')
*/
@Nonnull
public static JQueryInvocation idRefMultiple (@Nonnull @Nonempty final IJSExpression... aIDs)
{
ValueEnforcer.notEmptyNoNullValue (aIDs, "IDs");
final List aSelectors = new ArrayList ();
for (final IJSExpression aID : aIDs)
aSelectors.add (JQuerySelector.id (aID));
return JQuerySelector.multiple (aSelectors).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param aIDs
* The elements to be selected. May not be null
.
* @return A jQuery invocation with the passed IDs:
* $('#id1,#id2,#id3')
*/
@Nonnull
public static JQueryInvocation idRefMultiple (@Nonnull @Nonempty final IHCElement >... aIDs)
{
ValueEnforcer.notEmptyNoNullValue (aIDs, "IDs");
final List aSelectors = new ArrayList ();
for (final IHCElement > aID : aIDs)
aSelectors.add (JQuerySelector.id (aID));
return JQuerySelector.multiple (aSelectors).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param aIDs
* The IDs to be selected. May not be null
.
* @return A jQuery invocation with the passed IDs:
* $('#id1,#id2,#id3')
*/
@Nonnull
public static JQueryInvocation idRefMultiple (@Nonnull @Nonempty final Iterable aIDs)
{
ValueEnforcer.notEmpty (aIDs, "IDs");
final List aSelectors = new ArrayList ();
for (final String sID : aIDs)
aSelectors.add (JQuerySelector.id (sID));
return JQuerySelector.multiple (aSelectors).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param aCSSClass
* The class to be selected. May not be null
.
* @return A jQuery invocation with the passed class: $('.class')
*/
@Nonnull
public static JQueryInvocation classRef (@Nonnull final ICSSClassProvider aCSSClass)
{
return JQuerySelector.clazz (aCSSClass).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param aCSSClasses
* The classes to be selected. May not be null
.
* @return A jQuery invocation with the passed classes:
* $('.class1,.class2,.class3')
*/
@Nonnull
public static JQueryInvocation classRefMultiple (@Nonnull @Nonempty final ICSSClassProvider... aCSSClasses)
{
ValueEnforcer.notEmpty (aCSSClasses, "CSSClasses");
final List aSelectors = new ArrayList ();
for (final ICSSClassProvider aCSSClass : aCSSClasses)
aSelectors.add (JQuerySelector.clazz (aCSSClass));
return JQuerySelector.multiple (aSelectors).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param aCSSClasses
* The classes to be selected. May not be null
.
* @return A jQuery invocation with the passed classes:
* $('.class1,.class2,.class3')
*/
@Nonnull
public static JQueryInvocation classRefMultiple (@Nonnull @Nonempty final Iterable extends ICSSClassProvider> aCSSClasses)
{
ValueEnforcer.notEmpty (aCSSClasses, "CSSClasses");
final List aSelectors = new ArrayList ();
for (final ICSSClassProvider aCSSClass : aCSSClasses)
aSelectors.add (JQuerySelector.clazz (aCSSClass));
return JQuerySelector.multiple (aSelectors).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param eElement
* The HTML element to be selected. May not be null
.
* @return A jQuery invocation with the passed element:
* $('element')
*/
@Nonnull
public static JQueryInvocation elementNameRef (@Nonnull final EHTMLElement eElement)
{
return JQuerySelector.element (eElement).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param sElementName
* The HTML element to be selected. May not be null
.
* @return A jQuery invocation with the passed element:
* $('element')
*/
@Nonnull
public static JQueryInvocation elementNameRef (@Nonnull @Nonempty final String sElementName)
{
return JQuerySelector.element (sElementName).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param eElement
* The HTML element to be selected. May not be null
.
* @param aSelector
* The additional selector to be appended to the element. E.g an ID or
* a class selector. May not be null
.
* @return A jQuery invocation with the passed element and selector:
* $('element#id')
or $('element.class')
*/
@Nonnull
public static JQueryInvocation elementNameRef (@Nonnull final EHTMLElement eElement,
@Nonnull final IJQuerySelector aSelector)
{
return JQuerySelector.element (eElement).chain (aSelector).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param sElementName
* The HTML element to be selected. May not be null
.
* @param aSelector
* The additional selector to be appended to the element. E.g an ID or
* a class selector. May not be null
.
* @return A jQuery invocation with the passed element and selector:
* $('element#id')
or $('element.class')
*/
@Nonnull
public static JQueryInvocation elementNameRef (@Nonnull @Nonempty final String sElementName,
@Nonnull final IJQuerySelector aSelector)
{
return JQuerySelector.element (sElementName).chain (aSelector).invoke ();
}
/**
* Get the result of a jQuery selection
*
* @param eElement
* The HTML element to be selected. May not be null
.
* @param sID
* The ID to be appended to the element. May not be null
.
* @return A jQuery invocation with the passed element and ID:
* $('element#id')
*/
@Nonnull
public static JQueryInvocation elementNameWithIDRef (@Nonnull final EHTMLElement eElement,
@Nonnull @Nonempty final String sID)
{
return elementNameRef (eElement, JQuerySelector.id (sID));
}
/**
* Get the result of a jQuery selection
*
* @param sElementName
* The HTML element to be selected. May not be null
.
* @param sID
* The ID to be appended to the element. May not be null
.
* @return A jQuery invocation with the passed element and ID:
* $('element#id')
*/
@Nonnull
public static JQueryInvocation elementNameWithIDRef (@Nonnull @Nonempty final String sElementName,
@Nonnull @Nonempty final String sID)
{
return elementNameRef (sElementName, JQuerySelector.id (sID));
}
/**
* Get the result of a jQuery selection
*
* @param eElement
* The HTML element to be selected. May not be null
.
* @param aCSSClass
* The class to be appended to the element. May not be
* null
.
* @return A jQuery invocation with the passed element and class:
* $('element.class')
*/
@Nonnull
public static JQueryInvocation elementNameWithClassRef (@Nonnull final EHTMLElement eElement,
@Nonnull final ICSSClassProvider aCSSClass)
{
return elementNameRef (eElement, JQuerySelector.clazz (aCSSClass));
}
/**
* Get the result of a jQuery selection
*
* @param sElementName
* The HTML element to be selected. May not be null
.
* @param aCSSClass
* The class to be appended to the element. May not be
* null
.
* @return A jQuery invocation with the passed element and class:
* $('element.class')
*/
@Nonnull
public static JQueryInvocation elementNameWithClassRef (@Nonnull @Nonempty final String sElementName,
@Nonnull final ICSSClassProvider aCSSClass)
{
return elementNameRef (sElementName, JQuerySelector.clazz (aCSSClass));
}
/**
* @return A pair consisting of the invocation and the anonymous function that
* can be filled with code to be executed.
*/
@Nonnull
public static IReadonlyPair onDocumentReady ()
{
final JSAnonymousFunction aAnonFunction = new JSAnonymousFunction ();
final JQueryInvocation aInvocation = jQueryDocument ().ready (aAnonFunction);
return ReadonlyPair.create (aInvocation, aAnonFunction);
}
/**
* Add onDocumentReady call with a single statement
*
* @param aJSCodeProvider
* The statement to be executed on document ready
* @return The invocation object
*/
@Nonnull
public static JQueryInvocation onDocumentReady (@Nonnull final IJSCodeProvider aJSCodeProvider)
{
final IReadonlyPair aPair = onDocumentReady ();
aPair.getSecond ().body ().add (aJSCodeProvider);
return aPair.getFirst ();
}
// Everything starting from here is automatically generated:
/**
* @return The invocation of the static jQuery function
* jQuery.Callbacks()
with return type Callbacks
* @since jQuery 1.7
*/
@Nonnull
public static JQueryInvocation Callbacks ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "Callbacks");
}
/**
* @return The invocation of the static jQuery function
* jQuery.Deferred()
with return type Deferred
* @since jQuery 1.5
*/
@Nonnull
public static JQueryInvocation Deferred ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "Deferred");
}
/**
* @return The invocation of the static jQuery function
* jQuery.ajax()
with return type jqXHR
*/
@Nonnull
public static JQueryInvocation ajax ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "ajax");
}
/**
* @return The invocation of the static jQuery function
* jQuery.ajaxPrefilter()
with return type undefined
* @since jQuery 1.5
*/
@Nonnull
public static JQueryInvocation ajaxPrefilter ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "ajaxPrefilter");
}
/**
* @return The invocation of the static jQuery function
* jQuery.ajaxSetup()
with return type
* @since jQuery 1.1
*/
@Nonnull
public static JQueryInvocation ajaxSetup ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "ajaxSetup");
}
/**
* @return The invocation of the static jQuery function
* jQuery.ajaxTransport()
with return type undefined
* @since jQuery 1.5
*/
@Nonnull
public static JQueryInvocation ajaxTransport ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "ajaxTransport");
}
/**
* @return The invocation of the static jQuery function
* jQuery.contains()
with return type Boolean
* @since jQuery 1.4
*/
@Nonnull
public static JQueryInvocation contains ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "contains");
}
/**
* @return The invocation of the static jQuery function
* jQuery.data()
with return type Object
*/
@Nonnull
public static JQueryInvocation data ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "data");
}
/**
* @return The invocation of the static jQuery function
* jQuery.dequeue()
with return type undefined
* @since jQuery 1.3
*/
@Nonnull
public static JQueryInvocation dequeue ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "dequeue");
}
/**
* @return The invocation of the static jQuery function
* jQuery.each()
with return type Object
*/
@Nonnull
public static JQueryInvocation each ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "each");
}
/**
* @return The invocation of the static jQuery function
* jQuery.error()
with return type
* @since jQuery 1.4.1
*/
@Nonnull
public static JQueryInvocation error ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "error");
}
/**
* @return The invocation of the static jQuery function
* jQuery.extend()
with return type Object
*/
@Nonnull
public static JQueryInvocation extend ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "extend");
}
/**
* @return The invocation of the static jQuery function
* jQuery.fn.extend()
with return type Object
*/
@Nonnull
public static JQueryInvocation fn_extend ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "fn.extend");
}
/**
* @return The invocation of the static jQuery function
* jQuery.get()
with return type jqXHR
*/
@Nonnull
public static JQueryInvocation get ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "get");
}
/**
* @return The invocation of the static jQuery function
* jQuery.getJSON()
with return type jqXHR
*/
@Nonnull
public static JQueryInvocation getJSON ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "getJSON");
}
/**
* @return The invocation of the static jQuery function
* jQuery.getScript()
with return type jqXHR
*/
@Nonnull
public static JQueryInvocation getScript ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "getScript");
}
/**
* @return The invocation of the static jQuery function
* jQuery.globalEval()
with return type
* @since jQuery 1.0.4
*/
@Nonnull
public static JQueryInvocation globalEval ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "globalEval");
}
/**
* @return The invocation of the static jQuery function
* jQuery.grep()
with return type Array
*/
@Nonnull
public static JQueryInvocation grep ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "grep");
}
/**
* @return The invocation of the static jQuery function
* jQuery.hasData()
with return type Boolean
* @since jQuery 1.5
*/
@Nonnull
public static JQueryInvocation hasData ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "hasData");
}
/**
* @return The invocation of the static jQuery function
* jQuery.holdReady()
with return type undefined
* @since jQuery 1.6
*/
@Nonnull
public static JQueryInvocation holdReady ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "holdReady");
}
/**
* @return The invocation of the static jQuery function
* jQuery.inArray()
with return type Number
* @since jQuery 1.2
*/
@Nonnull
public static JQueryInvocation inArray ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "inArray");
}
/**
* @return The invocation of the static jQuery function
* jQuery.isArray()
with return type boolean
* @since jQuery 1.3
*/
@Nonnull
public static JQueryInvocation isArray ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "isArray");
}
/**
* @return The invocation of the static jQuery function
* jQuery.isEmptyObject()
with return type Boolean
* @since jQuery 1.4
*/
@Nonnull
public static JQueryInvocation isEmptyObject ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "isEmptyObject");
}
/**
* @return The invocation of the static jQuery function
* jQuery.isFunction()
with return type boolean
* @since jQuery 1.2
*/
@Nonnull
public static JQueryInvocation isFunction ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "isFunction");
}
/**
* @return The invocation of the static jQuery function
* jQuery.isNumeric()
with return type Boolean
* @since jQuery 1.7
*/
@Nonnull
public static JQueryInvocation isNumeric ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "isNumeric");
}
/**
* @return The invocation of the static jQuery function
* jQuery.isPlainObject()
with return type Boolean
* @since jQuery 1.4
*/
@Nonnull
public static JQueryInvocation isPlainObject ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "isPlainObject");
}
/**
* @return The invocation of the static jQuery function
* jQuery.isWindow()
with return type boolean
* @since jQuery 1.4.3
*/
@Nonnull
public static JQueryInvocation isWindow ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "isWindow");
}
/**
* @return The invocation of the static jQuery function
* jQuery.isXMLDoc()
with return type Boolean
* @since jQuery 1.1.4
*/
@Nonnull
public static JQueryInvocation isXMLDoc ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "isXMLDoc");
}
/**
* @return The invocation of the static jQuery function
* jQuery.makeArray()
with return type Array
* @since jQuery 1.2
*/
@Nonnull
public static JQueryInvocation makeArray ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "makeArray");
}
/**
* @return The invocation of the static jQuery function
* jQuery.map()
with return type Array
*/
@Nonnull
public static JQueryInvocation map ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "map");
}
/**
* @return The invocation of the static jQuery function
* jQuery.merge()
with return type Array
*/
@Nonnull
public static JQueryInvocation merge ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "merge");
}
/**
* @return The invocation of the static jQuery function
* jQuery.noConflict()
with return type Object
*/
@Nonnull
public static JQueryInvocation noConflict ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "noConflict");
}
/**
* @return The invocation of the static jQuery function
* jQuery.noop()
with return type undefined
* @since jQuery 1.4
*/
@Nonnull
public static JQueryInvocation noop ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "noop");
}
/**
* @return The invocation of the static jQuery function
* jQuery.now()
with return type Number
* @since jQuery 1.4.3
*/
@Nonnull
public static JQueryInvocation now ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "now");
}
/**
* @return The invocation of the static jQuery function
* jQuery.param()
with return type String
*/
@Nonnull
public static JQueryInvocation param ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "param");
}
/**
* @return The invocation of the static jQuery function
* jQuery.parseHTML()
with return type Array
* @since jQuery 1.8
*/
@Nonnull
public static JQueryInvocation parseHTML ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "parseHTML");
}
/**
* @return The invocation of the static jQuery function
* jQuery.parseJSON()
with return type Object
* @since jQuery 1.4.1
*/
@Nonnull
public static JQueryInvocation parseJSON ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "parseJSON");
}
/**
* @return The invocation of the static jQuery function
* jQuery.parseXML()
with return type XMLDocument
* @since jQuery 1.5
*/
@Nonnull
public static JQueryInvocation parseXML ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "parseXML");
}
/**
* @return The invocation of the static jQuery function
* jQuery.post()
with return type jqXHR
*/
@Nonnull
public static JQueryInvocation post ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "post");
}
/**
* @return The invocation of the static jQuery function
* jQuery.proxy()
with return type Function
*/
@Nonnull
public static JQueryInvocation proxy ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "proxy");
}
/**
* @return The invocation of the static jQuery function
* jQuery.queue()
with return type Array or jQuery
*/
@Nonnull
public static JQueryInvocation queue ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "queue");
}
/**
* @return The invocation of the static jQuery function
* jQuery.removeData()
with return type jQuery
* @since jQuery 1.2.3
*/
@Nonnull
public static JQueryInvocation removeData ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "removeData");
}
/**
* @return The invocation of the static jQuery function
* jQuery.sub()
with return type jQuery
* @deprecated Deprecated since jQuery 1.7
* @since jQuery 1.5
*/
@Nonnull
@Deprecated
public static JQueryInvocation sub ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "sub");
}
/**
* @return The invocation of the static jQuery function
* jQuery.trim()
with return type String
*/
@Nonnull
public static JQueryInvocation trim ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "trim");
}
/**
* @return The invocation of the static jQuery function
* jQuery.type()
with return type String
* @since jQuery 1.4.3
*/
@Nonnull
public static JQueryInvocation type ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "type");
}
/**
* @return The invocation of the static jQuery function
* jQuery.unique()
with return type Array
* @since jQuery 1.1.3
*/
@Nonnull
public static JQueryInvocation unique ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "unique");
}
/**
* @return The invocation of the static jQuery function
* jQuery.when()
with return type Promise
* @since jQuery 1.5
*/
@Nonnull
public static JQueryInvocation when ()
{
return new JQueryInvocation (JQueryProperty.jQueryField (), "when");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy