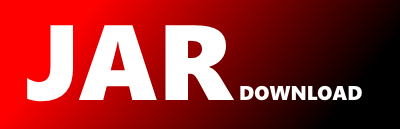
com.phloc.json.impl.value.JSONPropertyValueList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of phloc-json Show documentation
Show all versions of phloc-json Show documentation
Library for read and writing JSON objects in a typesafe manner
/**
* Copyright (C) 2006-2015 phloc systems
* http://www.phloc.com
* office[at]phloc[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.phloc.json.impl.value;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.phloc.commons.annotations.ReturnsMutableCopy;
import com.phloc.commons.collections.ContainerHelper;
import com.phloc.json.IJSONObject;
import com.phloc.json.IJSONPropertyValue;
import com.phloc.json.IJSONPropertyValueList;
import com.phloc.json.impl.CJSONConstants;
/**
* Default implementation of {@link IJSONPropertyValueList}
*
* @author Boris Gregorcic
* @param
* The inner data type
*/
public class JSONPropertyValueList > extends AbstractJSONPropertyValue > implements IJSONPropertyValueList
{
private static final long serialVersionUID = 6549899874312269115L;
/**
* Default ctor
*/
public JSONPropertyValueList ()
{
super (new ArrayList ());
}
/**
* Ctor
*
* @param aList
* List to use as the basis
*/
public JSONPropertyValueList (@Nullable final Iterable extends DATATYPE> aList)
{
super (ContainerHelper.newList (aList));
}
@Override
@Nonnull
public JSONPropertyValueList addValue (@Nonnull final DATATYPE aValue)
{
if (aValue == null)
{
throw new NullPointerException ("value"); //$NON-NLS-1$
}
getData ().add (aValue);
return this;
}
@Override
@Nonnull
public JSONPropertyValueList addAllValues (@Nonnull final Collection extends DATATYPE> aValues)
{
if (aValues == null)
{
throw new NullPointerException ("values"); //$NON-NLS-1$
}
getData ().addAll (aValues);
return this;
}
@Override
@Nonnull
@ReturnsMutableCopy
public List getValues ()
{
return ContainerHelper.newList (getData ());
}
@Override
@Nonnull
@ReturnsMutableCopy
public List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy