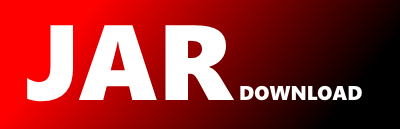
org.oclc.purl.dsdl.svrl.ActivePattern Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of phloc-schematron Show documentation
Show all versions of phloc-schematron Show documentation
Library for validating XML documents with Schematron
package org.oclc.purl.dsdl.svrl;
import java.io.Serializable;
import javax.annotation.Nullable;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlID;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import com.phloc.commons.equals.EqualsUtils;
import com.phloc.commons.hash.HashCodeGenerator;
import com.phloc.commons.string.ToStringGenerator;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="id" type="{http://www.w3.org/2001/XMLSchema}ID" />
* <attribute name="name" type="{http://www.w3.org/2001/XMLSchema}anySimpleType" />
* <attribute name="role" type="{http://www.w3.org/2001/XMLSchema}NMTOKEN" />
* <attribute name="document" type="{http://www.w3.org/2001/XMLSchema}anySimpleType" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
@XmlRootElement(name = "active-pattern")
public class ActivePattern implements Serializable
{
@XmlAttribute(name = "id")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlID
@XmlSchemaType(name = "ID")
private String id;
@XmlAttribute(name = "name")
@XmlSchemaType(name = "anySimpleType")
private String name;
@XmlAttribute(name = "role")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
private String role;
@XmlAttribute(name = "document")
@XmlSchemaType(name = "anySimpleType")
private String document;
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(
@Nullable
String value) {
this.id = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(
@Nullable
String value) {
this.name = value;
}
/**
* Gets the value of the role property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getRole() {
return role;
}
/**
* Sets the value of the role property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRole(
@Nullable
String value) {
this.role = value;
}
/**
* Gets the value of the document property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getDocument() {
return document;
}
/**
* Sets the value of the document property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDocument(
@Nullable
String value) {
this.document = value;
}
/**
* Created by phloc-jaxb22-plugin -Xphloc-equalshashcode
*
*/
@Override
public boolean equals(final Object o) {
if (o == this) {
return true;
}
if ((o == null)||(!getClass().equals(o.getClass()))) {
return false;
}
final ActivePattern rhs = ((ActivePattern) o);
if (!EqualsUtils.equals(id, rhs.id)) {
return false;
}
if (!EqualsUtils.equals(name, rhs.name)) {
return false;
}
if (!EqualsUtils.equals(role, rhs.role)) {
return false;
}
if (!EqualsUtils.equals(document, rhs.document)) {
return false;
}
return true;
}
/**
* Created by phloc-jaxb22-plugin -Xphloc-equalshashcode
*
*/
@Override
public int hashCode() {
return new HashCodeGenerator(this).append(id).append(name).append(role).append(document).getHashCode();
}
/**
* Created by phloc-jaxb22-plugin -Xphloc-tostring
*
*/
@Override
public String toString() {
return new ToStringGenerator(this).append("id", id).append("name", name).append("role", role).append("document", document).toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy