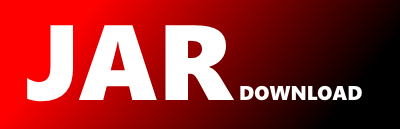
com.phloc.scopes.domain.ISessionScope Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of phloc-scopes Show documentation
Show all versions of phloc-scopes Show documentation
Library for handling scopes in a generic way
/**
* Copyright (C) 2006-2015 phloc systems
* http://www.phloc.com
* office[at]phloc[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.phloc.scopes.domain;
import java.util.Map;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.phloc.commons.annotations.Nonempty;
import com.phloc.commons.state.EContinue;
import com.phloc.scopes.IScope;
/**
* Interface for a single session scope object.
*
* @author Philip Helger
*/
public interface ISessionScope extends IScope
{
/**
* A special internal method that destroys the session. This is especially
* relevant for session web scope, because it is all done via the invalidation
* of the underlying HTTP session.
*
* @return {@link EContinue#BREAK} to indicate that the regular destruction
* should not be performed!
*/
@Nonnull
EContinue selfDestruct ();
/**
* Create the unique ID, under which a session application scope will be
* created within this scope. The default implementation is
* getID () + "." + sApplicationID
.
*
* @param sApplicationID
* The application ID to be used. May neither be null
nor
* empty.
* @return The application scope ID to be used.
* @see #getApplicationIDFromApplicationScopeID(String) to get the application
* ID from an application scope ID (reverse operation)
*/
@Nonnull
@Nonempty
String createApplicationScopeID (@Nonnull @Nonempty String sApplicationID);
/**
* Extract the application ID from an application scope ID.
*
* @param sApplicationScopeID
* The application scope ID to use. May be null
.
* @return null
if no application ID could be extracted
* @see #createApplicationScopeID(String) To creation an application scope ID
* from an application ID
*/
@Nullable
String getApplicationIDFromApplicationScopeID (@Nullable String sApplicationScopeID);
/**
* Create an application specific scope within the session.
*
* @param sApplicationID
* The application ID to use. May not be null
.
* @param bCreateIfNotExisting
* Create the session application scope if does not yet exist. If
* false
and the scope does not exist than
* null
is returned.
* @return null
if bCreateIfNotExisting is false
and
* the scope is not present
*/
@Nullable
ISessionApplicationScope getSessionApplicationScope (@Nonnull @Nonempty String sApplicationID,
boolean bCreateIfNotExisting);
/**
* Restore a persisted session application scope
*
* @param sScopeID
* The ID of the restored application scope. May neither be
* null
nor empty.
* @param aScope
* The scope to be restored. May not be null
.
*/
void restoreSessionApplicationScope (@Nonnull @Nonempty String sScopeID, @Nonnull ISessionApplicationScope aScope);
/**
* @return A non-null
map with all available session application
* scopes. The key is the application ID and the value is the scope.
*/
@Nonnull
Map getAllSessionApplicationScopes ();
/**
* @return The number of contained session application scopes. Always ≥ 0.
*/
@Nonnegative
int getSessionApplicationScopeCount ();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy