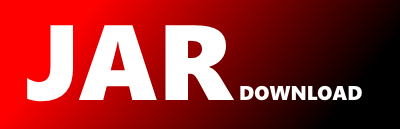
com.phloc.validation.validator.string.StringMaxLengthValidator Maven / Gradle / Ivy
/**
* Copyright (C) 2006-2014 phloc systems
* http://www.phloc.com
* office[at]phloc[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.phloc.validation.validator.string;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.phloc.commons.equals.EqualsUtils;
import com.phloc.commons.hash.HashCodeGenerator;
import com.phloc.commons.name.IHasDisplayText;
import com.phloc.commons.string.StringHelper;
import com.phloc.commons.string.ToStringGenerator;
import com.phloc.validation.EStandardValidationErrorTexts;
import com.phloc.validation.result.IValidationResult;
import com.phloc.validation.result.ValidationResultError;
import com.phloc.validation.result.ValidationResultSuccess;
/**
* Check whether a string fulfils a maximum length restriction.
*
* @author Philip Helger
*/
public final class StringMaxLengthValidator extends AbstractStringValidator
{
private final int m_nMaxLength;
private final IHasDisplayText m_aErrorText;
public StringMaxLengthValidator (@Nonnegative final int nMaxLength)
{
this (nMaxLength, null);
}
/**
* Constructor with custom error message.
*
* @param nMaxLength
* The maximum allowed string length. Must be > 0.
* @param aErrorText
* Optional error text. May be null
.
*/
public StringMaxLengthValidator (@Nonnegative final int nMaxLength, @Nullable final IHasDisplayText aErrorText)
{
if (nMaxLength <= 0)
throw new IllegalArgumentException ("max length must be > 0! Is: " + nMaxLength);
m_nMaxLength = nMaxLength;
m_aErrorText = aErrorText;
}
@Nonnull
public IValidationResult validate (@Nullable final String sValue)
{
if (StringHelper.getLength (sValue) <= m_nMaxLength)
return ValidationResultSuccess.getInstance ();
return m_aErrorText != null ? new ValidationResultError (m_aErrorText)
: new ValidationResultError (EStandardValidationErrorTexts.INVALID_MAXLENGTH,
Integer.toString (m_nMaxLength));
}
@Override
public boolean equals (final Object o)
{
if (o == this)
return true;
if (!super.equals (o))
return false;
final StringMaxLengthValidator rhs = (StringMaxLengthValidator) o;
return m_nMaxLength == rhs.m_nMaxLength && EqualsUtils.equals (m_aErrorText, rhs.m_aErrorText);
}
@Override
public int hashCode ()
{
return HashCodeGenerator.getDerived (super.hashCode ()).append (m_nMaxLength).append (m_aErrorText).getHashCode ();
}
@Override
public String toString ()
{
return ToStringGenerator.getDerived (super.toString ())
.append ("maxLength", m_nMaxLength)
.appendIfNotNull ("errorText", m_aErrorText)
.toString ();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy