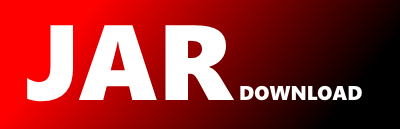
com.phloc.web.smtp.failed.FailedMailQueue Maven / Gradle / Ivy
/**
* Copyright (C) 2006-2015 phloc systems
* http://www.phloc.com
* office[at]phloc[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.phloc.web.smtp.failed;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.locks.ReadWriteLock;
import java.util.concurrent.locks.ReentrantReadWriteLock;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.annotation.concurrent.GuardedBy;
import javax.annotation.concurrent.ThreadSafe;
import com.phloc.commons.ValueEnforcer;
import com.phloc.commons.annotations.MustBeLocked;
import com.phloc.commons.annotations.MustBeLocked.ELockType;
import com.phloc.commons.annotations.ReturnsMutableCopy;
import com.phloc.commons.collections.ContainerHelper;
import com.phloc.commons.stats.IStatisticsHandlerCounter;
import com.phloc.commons.stats.StatisticsManager;
import com.phloc.commons.string.StringHelper;
import com.phloc.commons.string.ToStringGenerator;
/**
* This is a singleton object that keeps all the mails that could not be send.
*
* @author Philip Helger
*/
@ThreadSafe
public class FailedMailQueue implements Serializable
{
private static final IStatisticsHandlerCounter s_aStatsCountAdd = StatisticsManager.getCounterHandler (FailedMailQueue.class.getName () +
"$add");
private static final IStatisticsHandlerCounter s_aStatsCountRemove = StatisticsManager.getCounterHandler (FailedMailQueue.class.getName () +
"$remove");
protected final ReadWriteLock m_aRWLock = new ReentrantReadWriteLock ();
@GuardedBy ("m_aRWLock")
private final Map m_aMap = new LinkedHashMap ();
public FailedMailQueue ()
{}
@MustBeLocked (ELockType.WRITE)
protected void internalAdd (@Nonnull final FailedMailData aFailedMailData)
{
m_aMap.put (aFailedMailData.getID (), aFailedMailData);
s_aStatsCountAdd.increment ();
}
public void add (@Nonnull final FailedMailData aFailedMailData)
{
ValueEnforcer.notNull (aFailedMailData, "FailedMailData");
m_aRWLock.writeLock ().lock ();
try
{
internalAdd (aFailedMailData);
}
finally
{
m_aRWLock.writeLock ().unlock ();
}
}
@Nullable
@MustBeLocked (ELockType.WRITE)
protected FailedMailData internalRemove (@Nullable final String sID)
{
final FailedMailData ret = m_aMap.remove (sID);
if (ret != null)
s_aStatsCountRemove.increment ();
return ret;
}
/**
* Remove the failed mail at the given index.
*
* @param sID
* The ID of the failed mail data to be removed.
* @return null
if no such data exists
*/
@Nullable
public FailedMailData remove (@Nullable final String sID)
{
if (StringHelper.hasNoText (sID))
return null;
m_aRWLock.writeLock ().lock ();
try
{
return internalRemove (sID);
}
finally
{
m_aRWLock.writeLock ().unlock ();
}
}
@Nonnegative
@MustBeLocked (ELockType.READ)
protected int internalSize ()
{
return m_aMap.size ();
}
@Nonnegative
public int size ()
{
m_aRWLock.readLock ().lock ();
try
{
return internalSize ();
}
finally
{
m_aRWLock.readLock ().unlock ();
}
}
@Nullable
@MustBeLocked (ELockType.READ)
protected FailedMailData internalGetFailedMailOfID (@Nullable final String sID)
{
return m_aMap.get (sID);
}
@Nullable
public FailedMailData getFailedMailOfID (@Nullable final String sID)
{
m_aRWLock.readLock ().lock ();
try
{
return internalGetFailedMailOfID (sID);
}
finally
{
m_aRWLock.readLock ().unlock ();
}
}
@Nonnegative
@MustBeLocked (ELockType.READ)
protected int internalGetFailedMailCount ()
{
return m_aMap.size ();
}
@Nonnegative
public int getFailedMailCount ()
{
m_aRWLock.readLock ().lock ();
try
{
return internalGetFailedMailCount ();
}
finally
{
m_aRWLock.readLock ().unlock ();
}
}
@Nonnull
@ReturnsMutableCopy
@MustBeLocked (ELockType.READ)
protected List internalGetAllFailedMails ()
{
return ContainerHelper.newList (m_aMap.values ());
}
@Nonnull
@ReturnsMutableCopy
public List getAllFailedMails ()
{
m_aRWLock.readLock ().lock ();
try
{
return internalGetAllFailedMails ();
}
finally
{
m_aRWLock.readLock ().unlock ();
}
}
@Nonnull
@ReturnsMutableCopy
@MustBeLocked (ELockType.WRITE)
protected List internalRemoveAll ()
{
final List aTempList = new ArrayList (m_aMap.size ());
if (!m_aMap.isEmpty ())
{
aTempList.addAll (m_aMap.values ());
m_aMap.clear ();
}
return aTempList;
}
/**
* Remove and return all failed mails.
*
* @return All currently available failed mails. Never null
.
*/
@Nonnull
@ReturnsMutableCopy
public List removeAll ()
{
m_aRWLock.writeLock ().lock ();
try
{
return internalRemoveAll ();
}
finally
{
m_aRWLock.writeLock ().unlock ();
}
}
@Override
public String toString ()
{
return new ToStringGenerator (this).append ("map", m_aMap).toString ();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy