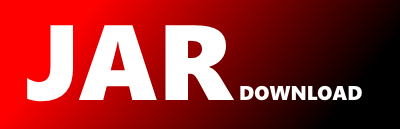
com.phloc.web.smtp.transport.MailTransport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of phloc-web Show documentation
Show all versions of phloc-web Show documentation
Library with basic web functionality
/**
* Copyright (C) 2006-2015 phloc systems
* http://www.phloc.com
* office[at]phloc[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.phloc.web.smtp.transport;
import java.util.Arrays;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Properties;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.mail.AuthenticationFailedException;
import javax.mail.Message.RecipientType;
import javax.mail.MessagingException;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.event.ConnectionListener;
import javax.mail.event.TransportEvent;
import javax.mail.event.TransportListener;
import javax.mail.internet.MimeMessage;
import javax.net.ssl.SSLSocketFactory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.phloc.commons.GlobalDebug;
import com.phloc.commons.ValueEnforcer;
import com.phloc.commons.annotations.ReturnsMutableCopy;
import com.phloc.commons.collections.ContainerHelper;
import com.phloc.commons.hash.HashCodeGenerator;
import com.phloc.commons.stats.IStatisticsHandlerCounter;
import com.phloc.commons.stats.StatisticsManager;
import com.phloc.commons.string.StringHelper;
import com.phloc.commons.string.ToStringGenerator;
import com.phloc.datetime.PDTFactory;
import com.phloc.web.WebExceptionHelper;
import com.phloc.web.smtp.EmailGlobalSettings;
import com.phloc.web.smtp.IEmailData;
import com.phloc.web.smtp.IEmailDataTransportListener;
import com.phloc.web.smtp.ISMTPSettings;
/**
* The wrapper around the main javax.mail transport
*
* @author Philip Helger
*/
final class MailTransport
{
public static final String SMTP_PROTOCOL = "smtp";
public static final String SMTPS_PROTOCOL = "smtps";
private static final IStatisticsHandlerCounter s_aStatsCountSuccess = StatisticsManager.getCounterHandler (MailTransport.class);
private static final IStatisticsHandlerCounter s_aStatsCountFailed = StatisticsManager.getCounterHandler (MailTransport.class.getName () +
"$failed");
private static final Logger s_aLogger = LoggerFactory.getLogger (MailTransport.class);
private static final String HEADER_MESSAGE_ID = "Message-ID";
private final ISMTPSettings m_aSMTPSettings;
private final boolean m_bSMTPS;
private final Properties m_aMailProperties = new Properties ();
private final Session m_aSession;
public MailTransport (@Nonnull final ISMTPSettings aSettings)
{
ValueEnforcer.notNull (aSettings, "Settings");
m_aSMTPSettings = aSettings;
m_bSMTPS = aSettings.isSSLEnabled () || aSettings.isSTARTTLSEnabled ();
// Enable SSL?
if (aSettings.isSSLEnabled ())
m_aMailProperties.setProperty (ESMTPTransportProperty.SSL_ENABLE.getPropertyName (m_bSMTPS),
Boolean.TRUE.toString ());
// Check if authentication is required
if (StringHelper.hasText (aSettings.getUserName ()))
m_aMailProperties.setProperty (ESMTPTransportProperty.AUTH.getPropertyName (m_bSMTPS), Boolean.TRUE.toString ());
// Enable STARTTLS?
if (aSettings.isSTARTTLSEnabled ())
m_aMailProperties.setProperty (ESMTPTransportProperty.STARTTLS_ENABLE.getPropertyName (m_bSMTPS),
Boolean.TRUE.toString ());
if (m_bSMTPS)
{
m_aMailProperties.setProperty (ESMTPTransportProperty.SSL_SOCKETFACTORY_CLASS.getPropertyName (m_bSMTPS),
SSLSocketFactory.class.getName ());
m_aMailProperties.setProperty (ESMTPTransportProperty.SSL_SOCKETFACTORY_PORT.getPropertyName (m_bSMTPS),
Integer.toString (aSettings.getPort ()));
}
// Set connection timeout
final long nConnectionTimeoutMilliSecs = aSettings.getConnectionTimeoutMilliSecs ();
if (nConnectionTimeoutMilliSecs > 0)
m_aMailProperties.setProperty (ESMTPTransportProperty.CONNECTIONTIMEOUT.getPropertyName (m_bSMTPS),
Long.toString (nConnectionTimeoutMilliSecs));
// Set socket timeout
final long nTimeoutMilliSecs = aSettings.getTimeoutMilliSecs ();
if (nTimeoutMilliSecs > 0)
m_aMailProperties.setProperty (ESMTPTransportProperty.TIMEOUT.getPropertyName (m_bSMTPS),
Long.toString (nTimeoutMilliSecs));
if (false)
m_aMailProperties.setProperty (ESMTPTransportProperty.REPORTSUCCESS.getPropertyName (m_bSMTPS),
Boolean.TRUE.toString ());
// Debug flag
m_aMailProperties.setProperty ("mail.debug.auth", Boolean.toString (GlobalDebug.isDebugMode ()));
if (s_aLogger.isDebugEnabled ())
s_aLogger.debug ("Mail properties: " + m_aMailProperties);
// Create session based on properties
m_aSession = Session.getInstance (m_aMailProperties);
// Set after eventual properties are set, because in setJavaMailProperties,
// the session is reset!
m_aSession.setDebug (GlobalDebug.isDebugMode ());
}
@Nonnull
public ISMTPSettings getSMTPSettings ()
{
return m_aSMTPSettings;
}
@Nonnull
@ReturnsMutableCopy
public Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy