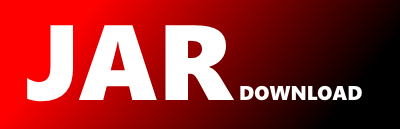
com.phloc.web.fileupload.util.AbstractLimitedInputStream Maven / Gradle / Ivy
Show all versions of phloc-web Show documentation
/**
* Copyright (C) 2006-2015 phloc systems
* http://www.phloc.com
* office[at]phloc[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.phloc.web.fileupload.util;
import java.io.FilterInputStream;
import java.io.IOException;
import java.io.InputStream;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import com.phloc.commons.ValueEnforcer;
/**
* An input stream, which limits its data size. This stream is used, if the
* content length is unknown.
*/
public abstract class AbstractLimitedInputStream extends FilterInputStream implements ICloseable
{
/**
* The maximum size of an item, in bytes.
*/
private final long m_nSizeMax;
/**
* The current number of bytes.
*/
private long m_nCount;
/**
* Whether this stream is already closed.
*/
private boolean m_bClosed;
/**
* Creates a new instance.
*
* @param pIn
* The input stream, which shall be limited.
* @param pSizeMax
* The limit; no more than this number of bytes shall be returned by
* the source stream.
*/
public AbstractLimitedInputStream (final InputStream pIn, final long pSizeMax)
{
super (pIn);
m_nSizeMax = pSizeMax;
}
/**
* Called to indicate, that the input streams limit has been exceeded.
*
* @param pSizeMax
* The input streams limit, in bytes.
* @param pCount
* The actual number of bytes.
* @throws IOException
* The called method is expected to raise an IOException.
*/
protected abstract void raiseError (long pSizeMax, long pCount) throws IOException;
/**
* Called to check, whether the input streams limit is reached.
*
* @throws IOException
* The given limit is exceeded.
*/
private void _checkLimit () throws IOException
{
if (m_nCount > m_nSizeMax)
{
raiseError (m_nSizeMax, m_nCount);
}
}
/**
* Reads the next byte of data from this input stream. The value byte is
* returned as an int
in the range 0
to
* 255
. If no byte is available because the end of the stream has
* been reached, the value -1
is returned. This method blocks
* until input data is available, the end of the stream is detected, or an
* exception is thrown.
*
* This method simply performs in.read()
and returns the result.
*
* @return the next byte of data, or -1
if the end of the stream
* is reached.
* @exception IOException
* if an I/O error occurs.
* @see java.io.FilterInputStream
*/
@Override
public int read () throws IOException
{
final int res = super.read ();
if (res != -1)
{
m_nCount++;
_checkLimit ();
}
return res;
}
/**
* Reads up to len
bytes of data from this input stream into an
* array of bytes. If len
is not zero, the method blocks until
* some input is available; otherwise, no bytes are read and 0
is
* returned.
*
* This method simply performs in.read(b, off, len)
and returns
* the result.
*
* @param b
* the buffer into which the data is read.
* @param nOfs
* The start offset in the destination array b
.
* @param nLen
* the maximum number of bytes read.
* @return the total number of bytes read into the buffer, or -1
* if there is no more data because the end of the stream has been
* reached.
* @exception IndexOutOfBoundsException
* If off
is negative, len
is negative,
* or len
is greater than b.length - off
* @exception IOException
* if an I/O error occurs.
* @see java.io.FilterInputStream
*/
@Override
public int read (@Nonnull final byte [] b, @Nonnegative final int nOfs, @Nonnegative final int nLen) throws IOException
{
ValueEnforcer.isArrayOfsLen (b, nOfs, nLen);
final int res = super.read (b, nOfs, nLen);
if (res > 0)
{
m_nCount += res;
_checkLimit ();
}
return res;
}
/**
* Returns, whether this stream is already closed.
*
* @return True, if the stream is closed, otherwise false.
* @throws IOException
* An I/O error occurred.
*/
public boolean isClosed () throws IOException
{
return m_bClosed;
}
/**
* Closes this input stream and releases any system resources associated with
* the stream. This method simply performs in.close()
.
*
* @exception IOException
* if an I/O error occurs.
* @see java.io.FilterInputStream
*/
@Override
public void close () throws IOException
{
m_bClosed = true;
super.close ();
}
}