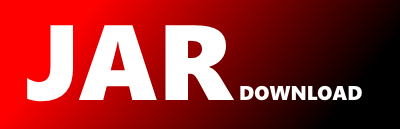
chapi.ast.antlr.KotlinParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of chapi-ast-kotlin Show documentation
Show all versions of chapi-ast-kotlin Show documentation
Chapi is A common language meta information convertor, convert different languages to same meta-data model
// Generated from KotlinParser.g4 by ANTLR 4.13.1
package chapi.ast.antlr;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast", "CheckReturnValue"})
public class KotlinParser extends Parser {
static { RuntimeMetaData.checkVersion("4.13.1", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
ShebangLine=1, DelimitedComment=2, LineComment=3, WS=4, NL=5, RESERVED=6,
DOT=7, COMMA=8, LPAREN=9, RPAREN=10, LSQUARE=11, RSQUARE=12, LCURL=13,
RCURL=14, MULT=15, MOD=16, DIV=17, ADD=18, SUB=19, INCR=20, DECR=21, CONJ=22,
DISJ=23, EXCL_WS=24, EXCL_NO_WS=25, COLON=26, SEMICOLON=27, ASSIGNMENT=28,
ADD_ASSIGNMENT=29, SUB_ASSIGNMENT=30, MULT_ASSIGNMENT=31, DIV_ASSIGNMENT=32,
MOD_ASSIGNMENT=33, ARROW=34, DOUBLE_ARROW=35, RANGE=36, COLONCOLON=37,
DOUBLE_SEMICOLON=38, HASH=39, AT_NO_WS=40, AT_POST_WS=41, AT_PRE_WS=42,
AT_BOTH_WS=43, QUEST_WS=44, QUEST_NO_WS=45, LANGLE=46, RANGLE=47, LE=48,
GE=49, EXCL_EQ=50, EXCL_EQEQ=51, AS_SAFE=52, EQEQ=53, EQEQEQ=54, SINGLE_QUOTE=55,
RETURN_AT=56, CONTINUE_AT=57, BREAK_AT=58, THIS_AT=59, SUPER_AT=60, FILE=61,
FIELD=62, PROPERTY=63, GET=64, SET=65, RECEIVER=66, PARAM=67, SETPARAM=68,
DELEGATE=69, PACKAGE=70, IMPORT=71, CLASS=72, INTERFACE=73, FUN=74, OBJECT=75,
VAL=76, VAR=77, TYPE_ALIAS=78, CONSTRUCTOR=79, BY=80, COMPANION=81, INIT=82,
THIS=83, SUPER=84, TYPEOF=85, WHERE=86, IF=87, ELSE=88, WHEN=89, TRY=90,
CATCH=91, FINALLY=92, FOR=93, DO=94, WHILE=95, THROW=96, RETURN=97, CONTINUE=98,
BREAK=99, AS=100, IS=101, IN=102, NOT_IS=103, NOT_IN=104, OUT=105, DYNAMIC=106,
PUBLIC=107, PRIVATE=108, PROTECTED=109, INTERNAL=110, ENUM=111, SEALED=112,
ANNOTATION=113, DATA=114, INNER=115, VALUE=116, TAILREC=117, OPERATOR=118,
INLINE=119, INFIX=120, EXTERNAL=121, SUSPEND=122, OVERRIDE=123, ABSTRACT=124,
FINAL=125, OPEN=126, CONST=127, LATEINIT=128, VARARG=129, NOINLINE=130,
CROSSINLINE=131, REIFIED=132, EXPECT=133, ACTUAL=134, RealLiteral=135,
FloatLiteral=136, DoubleLiteral=137, IntegerLiteral=138, HexLiteral=139,
BinLiteral=140, UnsignedLiteral=141, LongLiteral=142, BooleanLiteral=143,
NullLiteral=144, CharacterLiteral=145, Identifier=146, IdentifierOrSoftKey=147,
FieldIdentifier=148, QUOTE_OPEN=149, TRIPLE_QUOTE_OPEN=150, UNICODE_CLASS_LL=151,
UNICODE_CLASS_LM=152, UNICODE_CLASS_LO=153, UNICODE_CLASS_LT=154, UNICODE_CLASS_LU=155,
UNICODE_CLASS_ND=156, UNICODE_CLASS_NL=157, QUOTE_CLOSE=158, LineStrRef=159,
LineStrText=160, LineStrEscapedChar=161, LineStrExprStart=162, TRIPLE_QUOTE_CLOSE=163,
MultiLineStringQuote=164, MultiLineStrRef=165, MultiLineStrText=166, MultiLineStrExprStart=167,
Inside_Comment=168, Inside_WS=169, Inside_NL=170, ErrorCharacter=171;
public static final int
RULE_kotlinFile = 0, RULE_script = 1, RULE_shebangLine = 2, RULE_fileAnnotation = 3,
RULE_packageHeader = 4, RULE_importList = 5, RULE_importHeader = 6, RULE_importAlias = 7,
RULE_topLevelObject = 8, RULE_typeAlias = 9, RULE_declaration = 10, RULE_classDeclaration = 11,
RULE_primaryConstructor = 12, RULE_classBody = 13, RULE_classParameters = 14,
RULE_classParameter = 15, RULE_delegationSpecifiers = 16, RULE_delegationSpecifier = 17,
RULE_constructorInvocation = 18, RULE_annotatedDelegationSpecifier = 19,
RULE_explicitDelegation = 20, RULE_typeParameters = 21, RULE_typeParameter = 22,
RULE_typeConstraints = 23, RULE_typeConstraint = 24, RULE_classMemberDeclarations = 25,
RULE_classMemberDeclaration = 26, RULE_anonymousInitializer = 27, RULE_companionObject = 28,
RULE_functionValueParameters = 29, RULE_functionValueParameter = 30, RULE_functionDeclaration = 31,
RULE_functionBody = 32, RULE_variableDeclaration = 33, RULE_multiVariableDeclaration = 34,
RULE_propertyDeclaration = 35, RULE_propertyDelegate = 36, RULE_getter = 37,
RULE_setter = 38, RULE_parametersWithOptionalType = 39, RULE_functionValueParameterWithOptionalType = 40,
RULE_parameterWithOptionalType = 41, RULE_parameter = 42, RULE_objectDeclaration = 43,
RULE_secondaryConstructor = 44, RULE_constructorDelegationCall = 45, RULE_enumClassBody = 46,
RULE_enumEntries = 47, RULE_enumEntry = 48, RULE_type = 49, RULE_typeReference = 50,
RULE_nullableType = 51, RULE_quest = 52, RULE_userType = 53, RULE_simpleUserType = 54,
RULE_typeProjection = 55, RULE_typeProjectionModifiers = 56, RULE_typeProjectionModifier = 57,
RULE_functionType = 58, RULE_functionTypeParameters = 59, RULE_parenthesizedType = 60,
RULE_receiverType = 61, RULE_parenthesizedUserType = 62, RULE_statements = 63,
RULE_statement = 64, RULE_label = 65, RULE_controlStructureBody = 66,
RULE_block = 67, RULE_loopStatement = 68, RULE_forStatement = 69, RULE_whileStatement = 70,
RULE_doWhileStatement = 71, RULE_assignment = 72, RULE_semi = 73, RULE_semis = 74,
RULE_expression = 75, RULE_disjunction = 76, RULE_conjunction = 77, RULE_equality = 78,
RULE_comparison = 79, RULE_genericCallLikeComparison = 80, RULE_infixOperation = 81,
RULE_elvisExpression = 82, RULE_elvis = 83, RULE_infixFunctionCall = 84,
RULE_rangeExpression = 85, RULE_additiveExpression = 86, RULE_multiplicativeExpression = 87,
RULE_asExpression = 88, RULE_prefixUnaryExpression = 89, RULE_unaryPrefix = 90,
RULE_postfixUnaryExpression = 91, RULE_postfixUnarySuffix = 92, RULE_directlyAssignableExpression = 93,
RULE_parenthesizedDirectlyAssignableExpression = 94, RULE_assignableExpression = 95,
RULE_parenthesizedAssignableExpression = 96, RULE_assignableSuffix = 97,
RULE_indexingSuffix = 98, RULE_navigationSuffix = 99, RULE_callSuffix = 100,
RULE_annotatedLambda = 101, RULE_typeArguments = 102, RULE_valueArguments = 103,
RULE_valueArgument = 104, RULE_primaryExpression = 105, RULE_parenthesizedExpression = 106,
RULE_collectionLiteral = 107, RULE_literalConstant = 108, RULE_stringLiteral = 109,
RULE_lineStringLiteral = 110, RULE_multiLineStringLiteral = 111, RULE_lineStringContent = 112,
RULE_lineStringExpression = 113, RULE_multiLineStringContent = 114, RULE_multiLineStringExpression = 115,
RULE_lambdaLiteral = 116, RULE_lambdaParameters = 117, RULE_lambdaParameter = 118,
RULE_anonymousFunction = 119, RULE_functionLiteral = 120, RULE_objectLiteral = 121,
RULE_thisExpression = 122, RULE_superExpression = 123, RULE_ifExpression = 124,
RULE_whenSubject = 125, RULE_whenExpression = 126, RULE_whenEntry = 127,
RULE_whenCondition = 128, RULE_rangeTest = 129, RULE_typeTest = 130, RULE_tryExpression = 131,
RULE_catchBlock = 132, RULE_finallyBlock = 133, RULE_jumpExpression = 134,
RULE_callableReference = 135, RULE_assignmentAndOperator = 136, RULE_equalityOperator = 137,
RULE_comparisonOperator = 138, RULE_inOperator = 139, RULE_isOperator = 140,
RULE_additiveOperator = 141, RULE_multiplicativeOperator = 142, RULE_asOperator = 143,
RULE_prefixUnaryOperator = 144, RULE_postfixUnaryOperator = 145, RULE_excl = 146,
RULE_memberAccessOperator = 147, RULE_safeNav = 148, RULE_modifiers = 149,
RULE_parameterModifiers = 150, RULE_modifier = 151, RULE_typeModifiers = 152,
RULE_typeModifier = 153, RULE_classModifier = 154, RULE_memberModifier = 155,
RULE_visibilityModifier = 156, RULE_varianceModifier = 157, RULE_typeParameterModifiers = 158,
RULE_typeParameterModifier = 159, RULE_functionModifier = 160, RULE_propertyModifier = 161,
RULE_inheritanceModifier = 162, RULE_parameterModifier = 163, RULE_reificationModifier = 164,
RULE_platformModifier = 165, RULE_annotation = 166, RULE_singleAnnotation = 167,
RULE_multiAnnotation = 168, RULE_annotationUseSiteTarget = 169, RULE_unescapedAnnotation = 170,
RULE_simpleIdentifier = 171, RULE_identifier = 172;
private static String[] makeRuleNames() {
return new String[] {
"kotlinFile", "script", "shebangLine", "fileAnnotation", "packageHeader",
"importList", "importHeader", "importAlias", "topLevelObject", "typeAlias",
"declaration", "classDeclaration", "primaryConstructor", "classBody",
"classParameters", "classParameter", "delegationSpecifiers", "delegationSpecifier",
"constructorInvocation", "annotatedDelegationSpecifier", "explicitDelegation",
"typeParameters", "typeParameter", "typeConstraints", "typeConstraint",
"classMemberDeclarations", "classMemberDeclaration", "anonymousInitializer",
"companionObject", "functionValueParameters", "functionValueParameter",
"functionDeclaration", "functionBody", "variableDeclaration", "multiVariableDeclaration",
"propertyDeclaration", "propertyDelegate", "getter", "setter", "parametersWithOptionalType",
"functionValueParameterWithOptionalType", "parameterWithOptionalType",
"parameter", "objectDeclaration", "secondaryConstructor", "constructorDelegationCall",
"enumClassBody", "enumEntries", "enumEntry", "type", "typeReference",
"nullableType", "quest", "userType", "simpleUserType", "typeProjection",
"typeProjectionModifiers", "typeProjectionModifier", "functionType",
"functionTypeParameters", "parenthesizedType", "receiverType", "parenthesizedUserType",
"statements", "statement", "label", "controlStructureBody", "block",
"loopStatement", "forStatement", "whileStatement", "doWhileStatement",
"assignment", "semi", "semis", "expression", "disjunction", "conjunction",
"equality", "comparison", "genericCallLikeComparison", "infixOperation",
"elvisExpression", "elvis", "infixFunctionCall", "rangeExpression", "additiveExpression",
"multiplicativeExpression", "asExpression", "prefixUnaryExpression",
"unaryPrefix", "postfixUnaryExpression", "postfixUnarySuffix", "directlyAssignableExpression",
"parenthesizedDirectlyAssignableExpression", "assignableExpression",
"parenthesizedAssignableExpression", "assignableSuffix", "indexingSuffix",
"navigationSuffix", "callSuffix", "annotatedLambda", "typeArguments",
"valueArguments", "valueArgument", "primaryExpression", "parenthesizedExpression",
"collectionLiteral", "literalConstant", "stringLiteral", "lineStringLiteral",
"multiLineStringLiteral", "lineStringContent", "lineStringExpression",
"multiLineStringContent", "multiLineStringExpression", "lambdaLiteral",
"lambdaParameters", "lambdaParameter", "anonymousFunction", "functionLiteral",
"objectLiteral", "thisExpression", "superExpression", "ifExpression",
"whenSubject", "whenExpression", "whenEntry", "whenCondition", "rangeTest",
"typeTest", "tryExpression", "catchBlock", "finallyBlock", "jumpExpression",
"callableReference", "assignmentAndOperator", "equalityOperator", "comparisonOperator",
"inOperator", "isOperator", "additiveOperator", "multiplicativeOperator",
"asOperator", "prefixUnaryOperator", "postfixUnaryOperator", "excl",
"memberAccessOperator", "safeNav", "modifiers", "parameterModifiers",
"modifier", "typeModifiers", "typeModifier", "classModifier", "memberModifier",
"visibilityModifier", "varianceModifier", "typeParameterModifiers", "typeParameterModifier",
"functionModifier", "propertyModifier", "inheritanceModifier", "parameterModifier",
"reificationModifier", "platformModifier", "annotation", "singleAnnotation",
"multiAnnotation", "annotationUseSiteTarget", "unescapedAnnotation",
"simpleIdentifier", "identifier"
};
}
public static final String[] ruleNames = makeRuleNames();
private static String[] makeLiteralNames() {
return new String[] {
null, null, null, null, null, null, "'...'", "'.'", "','", "'('", "')'",
"'['", "']'", "'{'", "'}'", "'*'", "'%'", "'/'", "'+'", "'-'", "'++'",
"'--'", "'&&'", "'||'", null, "'!'", "':'", "';'", "'='", "'+='", "'-='",
"'*='", "'/='", "'%='", "'->'", "'=>'", "'..'", "'::'", "';;'", "'#'",
"'@'", null, null, null, null, "'?'", "'<'", "'>'", "'<='", "'>='", "'!='",
"'!=='", "'as?'", "'=='", "'==='", "'''", null, null, null, null, null,
"'file'", "'field'", "'property'", "'get'", "'set'", "'receiver'", "'param'",
"'setparam'", "'delegate'", "'package'", "'import'", "'class'", "'interface'",
"'fun'", "'object'", "'val'", "'var'", "'typealias'", "'constructor'",
"'by'", "'companion'", "'init'", "'this'", "'super'", "'typeof'", "'where'",
"'if'", "'else'", "'when'", "'try'", "'catch'", "'finally'", "'for'",
"'do'", "'while'", "'throw'", "'return'", "'continue'", "'break'", "'as'",
"'is'", "'in'", null, null, "'out'", "'dynamic'", "'public'", "'private'",
"'protected'", "'internal'", "'enum'", "'sealed'", "'annotation'", "'data'",
"'inner'", "'value'", "'tailrec'", "'operator'", "'inline'", "'infix'",
"'external'", "'suspend'", "'override'", "'abstract'", "'final'", "'open'",
"'const'", "'lateinit'", "'vararg'", "'noinline'", "'crossinline'", "'reified'",
"'expect'", "'actual'", null, null, null, null, null, null, null, null,
null, "'null'", null, null, null, null, null, "'\"\"\"'"
};
}
private static final String[] _LITERAL_NAMES = makeLiteralNames();
private static String[] makeSymbolicNames() {
return new String[] {
null, "ShebangLine", "DelimitedComment", "LineComment", "WS", "NL", "RESERVED",
"DOT", "COMMA", "LPAREN", "RPAREN", "LSQUARE", "RSQUARE", "LCURL", "RCURL",
"MULT", "MOD", "DIV", "ADD", "SUB", "INCR", "DECR", "CONJ", "DISJ", "EXCL_WS",
"EXCL_NO_WS", "COLON", "SEMICOLON", "ASSIGNMENT", "ADD_ASSIGNMENT", "SUB_ASSIGNMENT",
"MULT_ASSIGNMENT", "DIV_ASSIGNMENT", "MOD_ASSIGNMENT", "ARROW", "DOUBLE_ARROW",
"RANGE", "COLONCOLON", "DOUBLE_SEMICOLON", "HASH", "AT_NO_WS", "AT_POST_WS",
"AT_PRE_WS", "AT_BOTH_WS", "QUEST_WS", "QUEST_NO_WS", "LANGLE", "RANGLE",
"LE", "GE", "EXCL_EQ", "EXCL_EQEQ", "AS_SAFE", "EQEQ", "EQEQEQ", "SINGLE_QUOTE",
"RETURN_AT", "CONTINUE_AT", "BREAK_AT", "THIS_AT", "SUPER_AT", "FILE",
"FIELD", "PROPERTY", "GET", "SET", "RECEIVER", "PARAM", "SETPARAM", "DELEGATE",
"PACKAGE", "IMPORT", "CLASS", "INTERFACE", "FUN", "OBJECT", "VAL", "VAR",
"TYPE_ALIAS", "CONSTRUCTOR", "BY", "COMPANION", "INIT", "THIS", "SUPER",
"TYPEOF", "WHERE", "IF", "ELSE", "WHEN", "TRY", "CATCH", "FINALLY", "FOR",
"DO", "WHILE", "THROW", "RETURN", "CONTINUE", "BREAK", "AS", "IS", "IN",
"NOT_IS", "NOT_IN", "OUT", "DYNAMIC", "PUBLIC", "PRIVATE", "PROTECTED",
"INTERNAL", "ENUM", "SEALED", "ANNOTATION", "DATA", "INNER", "VALUE",
"TAILREC", "OPERATOR", "INLINE", "INFIX", "EXTERNAL", "SUSPEND", "OVERRIDE",
"ABSTRACT", "FINAL", "OPEN", "CONST", "LATEINIT", "VARARG", "NOINLINE",
"CROSSINLINE", "REIFIED", "EXPECT", "ACTUAL", "RealLiteral", "FloatLiteral",
"DoubleLiteral", "IntegerLiteral", "HexLiteral", "BinLiteral", "UnsignedLiteral",
"LongLiteral", "BooleanLiteral", "NullLiteral", "CharacterLiteral", "Identifier",
"IdentifierOrSoftKey", "FieldIdentifier", "QUOTE_OPEN", "TRIPLE_QUOTE_OPEN",
"UNICODE_CLASS_LL", "UNICODE_CLASS_LM", "UNICODE_CLASS_LO", "UNICODE_CLASS_LT",
"UNICODE_CLASS_LU", "UNICODE_CLASS_ND", "UNICODE_CLASS_NL", "QUOTE_CLOSE",
"LineStrRef", "LineStrText", "LineStrEscapedChar", "LineStrExprStart",
"TRIPLE_QUOTE_CLOSE", "MultiLineStringQuote", "MultiLineStrRef", "MultiLineStrText",
"MultiLineStrExprStart", "Inside_Comment", "Inside_WS", "Inside_NL",
"ErrorCharacter"
};
}
private static final String[] _SYMBOLIC_NAMES = makeSymbolicNames();
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "KotlinParser.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public KotlinParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
@SuppressWarnings("CheckReturnValue")
public static class KotlinFileContext extends ParserRuleContext {
public PackageHeaderContext packageHeader() {
return getRuleContext(PackageHeaderContext.class,0);
}
public ImportListContext importList() {
return getRuleContext(ImportListContext.class,0);
}
public TerminalNode EOF() { return getToken(KotlinParser.EOF, 0); }
public ShebangLineContext shebangLine() {
return getRuleContext(ShebangLineContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public List fileAnnotation() {
return getRuleContexts(FileAnnotationContext.class);
}
public FileAnnotationContext fileAnnotation(int i) {
return getRuleContext(FileAnnotationContext.class,i);
}
public List topLevelObject() {
return getRuleContexts(TopLevelObjectContext.class);
}
public TopLevelObjectContext topLevelObject(int i) {
return getRuleContext(TopLevelObjectContext.class,i);
}
public KotlinFileContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_kotlinFile; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterKotlinFile(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitKotlinFile(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitKotlinFile(this);
else return visitor.visitChildren(this);
}
}
public final KotlinFileContext kotlinFile() throws RecognitionException {
KotlinFileContext _localctx = new KotlinFileContext(_ctx, getState());
enterRule(_localctx, 0, RULE_kotlinFile);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(347);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ShebangLine) {
{
setState(346);
shebangLine();
}
}
setState(352);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(349);
match(NL);
}
}
setState(354);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(358);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,2,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(355);
fileAnnotation();
}
}
}
setState(360);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,2,_ctx);
}
setState(361);
packageHeader();
setState(362);
importList();
setState(366);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==AT_NO_WS || _la==AT_PRE_WS || ((((_la - 72)) & ~0x3f) == 0 && ((1L << (_la - 72)) & 8070450497888190591L) != 0)) {
{
{
setState(363);
topLevelObject();
}
}
setState(368);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(369);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ScriptContext extends ParserRuleContext {
public PackageHeaderContext packageHeader() {
return getRuleContext(PackageHeaderContext.class,0);
}
public ImportListContext importList() {
return getRuleContext(ImportListContext.class,0);
}
public TerminalNode EOF() { return getToken(KotlinParser.EOF, 0); }
public ShebangLineContext shebangLine() {
return getRuleContext(ShebangLineContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public List fileAnnotation() {
return getRuleContexts(FileAnnotationContext.class);
}
public FileAnnotationContext fileAnnotation(int i) {
return getRuleContext(FileAnnotationContext.class,i);
}
public List statement() {
return getRuleContexts(StatementContext.class);
}
public StatementContext statement(int i) {
return getRuleContext(StatementContext.class,i);
}
public List semi() {
return getRuleContexts(SemiContext.class);
}
public SemiContext semi(int i) {
return getRuleContext(SemiContext.class,i);
}
public ScriptContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_script; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterScript(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitScript(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitScript(this);
else return visitor.visitChildren(this);
}
}
public final ScriptContext script() throws RecognitionException {
ScriptContext _localctx = new ScriptContext(_ctx, getState());
enterRule(_localctx, 2, RULE_script);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(372);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ShebangLine) {
{
setState(371);
shebangLine();
}
}
setState(377);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(374);
match(NL);
}
}
setState(379);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(383);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,6,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(380);
fileAnnotation();
}
}
}
setState(385);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,6,_ctx);
}
setState(386);
packageHeader();
setState(387);
importList();
setState(393);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & -72051958986561024L) != 0) || ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -2130322653249L) != 0) || ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & 6814975L) != 0)) {
{
{
setState(388);
statement();
setState(389);
semi();
}
}
setState(395);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(396);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShebangLineContext extends ParserRuleContext {
public TerminalNode ShebangLine() { return getToken(KotlinParser.ShebangLine, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public ShebangLineContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_shebangLine; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterShebangLine(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitShebangLine(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitShebangLine(this);
else return visitor.visitChildren(this);
}
}
public final ShebangLineContext shebangLine() throws RecognitionException {
ShebangLineContext _localctx = new ShebangLineContext(_ctx, getState());
enterRule(_localctx, 4, RULE_shebangLine);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(398);
match(ShebangLine);
setState(400);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(399);
match(NL);
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(402);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,8,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FileAnnotationContext extends ParserRuleContext {
public TerminalNode FILE() { return getToken(KotlinParser.FILE, 0); }
public TerminalNode COLON() { return getToken(KotlinParser.COLON, 0); }
public TerminalNode AT_NO_WS() { return getToken(KotlinParser.AT_NO_WS, 0); }
public TerminalNode AT_PRE_WS() { return getToken(KotlinParser.AT_PRE_WS, 0); }
public TerminalNode LSQUARE() { return getToken(KotlinParser.LSQUARE, 0); }
public TerminalNode RSQUARE() { return getToken(KotlinParser.RSQUARE, 0); }
public List unescapedAnnotation() {
return getRuleContexts(UnescapedAnnotationContext.class);
}
public UnescapedAnnotationContext unescapedAnnotation(int i) {
return getRuleContext(UnescapedAnnotationContext.class,i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public FileAnnotationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_fileAnnotation; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterFileAnnotation(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitFileAnnotation(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitFileAnnotation(this);
else return visitor.visitChildren(this);
}
}
public final FileAnnotationContext fileAnnotation() throws RecognitionException {
FileAnnotationContext _localctx = new FileAnnotationContext(_ctx, getState());
enterRule(_localctx, 6, RULE_fileAnnotation);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(404);
_la = _input.LA(1);
if ( !(_la==AT_NO_WS || _la==AT_PRE_WS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(405);
match(FILE);
setState(409);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(406);
match(NL);
}
}
setState(411);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(412);
match(COLON);
setState(416);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(413);
match(NL);
}
}
setState(418);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(428);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LSQUARE:
{
setState(419);
match(LSQUARE);
setState(421);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(420);
unescapedAnnotation();
}
}
setState(423);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( ((((_la - 61)) & ~0x3f) == 0 && ((1L << (_la - 61)) & -17588927330817L) != 0) || ((((_la - 125)) & ~0x3f) == 0 && ((1L << (_la - 125)) & 2098175L) != 0) );
setState(425);
match(RSQUARE);
}
break;
case FILE:
case FIELD:
case PROPERTY:
case GET:
case SET:
case RECEIVER:
case PARAM:
case SETPARAM:
case DELEGATE:
case IMPORT:
case CONSTRUCTOR:
case BY:
case COMPANION:
case INIT:
case WHERE:
case CATCH:
case FINALLY:
case OUT:
case DYNAMIC:
case PUBLIC:
case PRIVATE:
case PROTECTED:
case INTERNAL:
case ENUM:
case SEALED:
case ANNOTATION:
case DATA:
case INNER:
case VALUE:
case TAILREC:
case OPERATOR:
case INLINE:
case INFIX:
case EXTERNAL:
case SUSPEND:
case OVERRIDE:
case ABSTRACT:
case FINAL:
case OPEN:
case CONST:
case LATEINIT:
case VARARG:
case NOINLINE:
case CROSSINLINE:
case REIFIED:
case EXPECT:
case ACTUAL:
case Identifier:
{
setState(427);
unescapedAnnotation();
}
break;
default:
throw new NoViableAltException(this);
}
setState(433);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(430);
match(NL);
}
}
setState(435);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PackageHeaderContext extends ParserRuleContext {
public TerminalNode PACKAGE() { return getToken(KotlinParser.PACKAGE, 0); }
public IdentifierContext identifier() {
return getRuleContext(IdentifierContext.class,0);
}
public SemiContext semi() {
return getRuleContext(SemiContext.class,0);
}
public PackageHeaderContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_packageHeader; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterPackageHeader(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitPackageHeader(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitPackageHeader(this);
else return visitor.visitChildren(this);
}
}
public final PackageHeaderContext packageHeader() throws RecognitionException {
PackageHeaderContext _localctx = new PackageHeaderContext(_ctx, getState());
enterRule(_localctx, 8, RULE_packageHeader);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(441);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PACKAGE) {
{
setState(436);
match(PACKAGE);
setState(437);
identifier();
setState(439);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,14,_ctx) ) {
case 1:
{
setState(438);
semi();
}
break;
}
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ImportListContext extends ParserRuleContext {
public List importHeader() {
return getRuleContexts(ImportHeaderContext.class);
}
public ImportHeaderContext importHeader(int i) {
return getRuleContext(ImportHeaderContext.class,i);
}
public ImportListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_importList; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterImportList(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitImportList(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitImportList(this);
else return visitor.visitChildren(this);
}
}
public final ImportListContext importList() throws RecognitionException {
ImportListContext _localctx = new ImportListContext(_ctx, getState());
enterRule(_localctx, 10, RULE_importList);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(446);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,16,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(443);
importHeader();
}
}
}
setState(448);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,16,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ImportHeaderContext extends ParserRuleContext {
public TerminalNode IMPORT() { return getToken(KotlinParser.IMPORT, 0); }
public IdentifierContext identifier() {
return getRuleContext(IdentifierContext.class,0);
}
public TerminalNode DOT() { return getToken(KotlinParser.DOT, 0); }
public TerminalNode MULT() { return getToken(KotlinParser.MULT, 0); }
public ImportAliasContext importAlias() {
return getRuleContext(ImportAliasContext.class,0);
}
public SemiContext semi() {
return getRuleContext(SemiContext.class,0);
}
public ImportHeaderContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_importHeader; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterImportHeader(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitImportHeader(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitImportHeader(this);
else return visitor.visitChildren(this);
}
}
public final ImportHeaderContext importHeader() throws RecognitionException {
ImportHeaderContext _localctx = new ImportHeaderContext(_ctx, getState());
enterRule(_localctx, 12, RULE_importHeader);
try {
enterOuterAlt(_localctx, 1);
{
setState(449);
match(IMPORT);
setState(450);
identifier();
setState(454);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DOT:
{
setState(451);
match(DOT);
setState(452);
match(MULT);
}
break;
case AS:
{
setState(453);
importAlias();
}
break;
case EOF:
case NL:
case LPAREN:
case LSQUARE:
case LCURL:
case ADD:
case SUB:
case INCR:
case DECR:
case EXCL_WS:
case EXCL_NO_WS:
case SEMICOLON:
case COLONCOLON:
case AT_NO_WS:
case AT_PRE_WS:
case RETURN_AT:
case CONTINUE_AT:
case BREAK_AT:
case THIS_AT:
case SUPER_AT:
case FILE:
case FIELD:
case PROPERTY:
case GET:
case SET:
case RECEIVER:
case PARAM:
case SETPARAM:
case DELEGATE:
case IMPORT:
case CLASS:
case INTERFACE:
case FUN:
case OBJECT:
case VAL:
case VAR:
case TYPE_ALIAS:
case CONSTRUCTOR:
case BY:
case COMPANION:
case INIT:
case THIS:
case SUPER:
case WHERE:
case IF:
case WHEN:
case TRY:
case CATCH:
case FINALLY:
case FOR:
case DO:
case WHILE:
case THROW:
case RETURN:
case CONTINUE:
case BREAK:
case OUT:
case DYNAMIC:
case PUBLIC:
case PRIVATE:
case PROTECTED:
case INTERNAL:
case ENUM:
case SEALED:
case ANNOTATION:
case DATA:
case INNER:
case VALUE:
case TAILREC:
case OPERATOR:
case INLINE:
case INFIX:
case EXTERNAL:
case SUSPEND:
case OVERRIDE:
case ABSTRACT:
case FINAL:
case OPEN:
case CONST:
case LATEINIT:
case VARARG:
case NOINLINE:
case CROSSINLINE:
case REIFIED:
case EXPECT:
case ACTUAL:
case RealLiteral:
case IntegerLiteral:
case HexLiteral:
case BinLiteral:
case UnsignedLiteral:
case LongLiteral:
case BooleanLiteral:
case NullLiteral:
case CharacterLiteral:
case Identifier:
case QUOTE_OPEN:
case TRIPLE_QUOTE_OPEN:
break;
default:
break;
}
setState(457);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,18,_ctx) ) {
case 1:
{
setState(456);
semi();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ImportAliasContext extends ParserRuleContext {
public TerminalNode AS() { return getToken(KotlinParser.AS, 0); }
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public ImportAliasContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_importAlias; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterImportAlias(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitImportAlias(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitImportAlias(this);
else return visitor.visitChildren(this);
}
}
public final ImportAliasContext importAlias() throws RecognitionException {
ImportAliasContext _localctx = new ImportAliasContext(_ctx, getState());
enterRule(_localctx, 14, RULE_importAlias);
try {
enterOuterAlt(_localctx, 1);
{
setState(459);
match(AS);
setState(460);
simpleIdentifier();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TopLevelObjectContext extends ParserRuleContext {
public DeclarationContext declaration() {
return getRuleContext(DeclarationContext.class,0);
}
public SemisContext semis() {
return getRuleContext(SemisContext.class,0);
}
public TopLevelObjectContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_topLevelObject; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterTopLevelObject(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitTopLevelObject(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitTopLevelObject(this);
else return visitor.visitChildren(this);
}
}
public final TopLevelObjectContext topLevelObject() throws RecognitionException {
TopLevelObjectContext _localctx = new TopLevelObjectContext(_ctx, getState());
enterRule(_localctx, 16, RULE_topLevelObject);
try {
enterOuterAlt(_localctx, 1);
{
setState(462);
declaration();
setState(464);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,19,_ctx) ) {
case 1:
{
setState(463);
semis();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeAliasContext extends ParserRuleContext {
public TerminalNode TYPE_ALIAS() { return getToken(KotlinParser.TYPE_ALIAS, 0); }
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public TerminalNode ASSIGNMENT() { return getToken(KotlinParser.ASSIGNMENT, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public ModifiersContext modifiers() {
return getRuleContext(ModifiersContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public TypeParametersContext typeParameters() {
return getRuleContext(TypeParametersContext.class,0);
}
public TypeAliasContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_typeAlias; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterTypeAlias(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitTypeAlias(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitTypeAlias(this);
else return visitor.visitChildren(this);
}
}
public final TypeAliasContext typeAlias() throws RecognitionException {
TypeAliasContext _localctx = new TypeAliasContext(_ctx, getState());
enterRule(_localctx, 18, RULE_typeAlias);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(467);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AT_NO_WS || _la==AT_PRE_WS || ((((_la - 107)) & ~0x3f) == 0 && ((1L << (_la - 107)) & 234881023L) != 0)) {
{
setState(466);
modifiers();
}
}
setState(469);
match(TYPE_ALIAS);
setState(473);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(470);
match(NL);
}
}
setState(475);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(476);
simpleIdentifier();
setState(484);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,23,_ctx) ) {
case 1:
{
setState(480);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(477);
match(NL);
}
}
setState(482);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(483);
typeParameters();
}
break;
}
setState(489);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(486);
match(NL);
}
}
setState(491);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(492);
match(ASSIGNMENT);
setState(496);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(493);
match(NL);
}
}
setState(498);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(499);
type();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DeclarationContext extends ParserRuleContext {
public ClassDeclarationContext classDeclaration() {
return getRuleContext(ClassDeclarationContext.class,0);
}
public ObjectDeclarationContext objectDeclaration() {
return getRuleContext(ObjectDeclarationContext.class,0);
}
public FunctionDeclarationContext functionDeclaration() {
return getRuleContext(FunctionDeclarationContext.class,0);
}
public PropertyDeclarationContext propertyDeclaration() {
return getRuleContext(PropertyDeclarationContext.class,0);
}
public TypeAliasContext typeAlias() {
return getRuleContext(TypeAliasContext.class,0);
}
public DeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_declaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final DeclarationContext declaration() throws RecognitionException {
DeclarationContext _localctx = new DeclarationContext(_ctx, getState());
enterRule(_localctx, 20, RULE_declaration);
try {
setState(506);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,26,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(501);
classDeclaration();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(502);
objectDeclaration();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(503);
functionDeclaration();
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(504);
propertyDeclaration();
}
break;
case 5:
enterOuterAlt(_localctx, 5);
{
setState(505);
typeAlias();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ClassDeclarationContext extends ParserRuleContext {
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public TerminalNode CLASS() { return getToken(KotlinParser.CLASS, 0); }
public TerminalNode INTERFACE() { return getToken(KotlinParser.INTERFACE, 0); }
public ModifiersContext modifiers() {
return getRuleContext(ModifiersContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public TypeParametersContext typeParameters() {
return getRuleContext(TypeParametersContext.class,0);
}
public PrimaryConstructorContext primaryConstructor() {
return getRuleContext(PrimaryConstructorContext.class,0);
}
public TerminalNode COLON() { return getToken(KotlinParser.COLON, 0); }
public DelegationSpecifiersContext delegationSpecifiers() {
return getRuleContext(DelegationSpecifiersContext.class,0);
}
public TypeConstraintsContext typeConstraints() {
return getRuleContext(TypeConstraintsContext.class,0);
}
public ClassBodyContext classBody() {
return getRuleContext(ClassBodyContext.class,0);
}
public EnumClassBodyContext enumClassBody() {
return getRuleContext(EnumClassBodyContext.class,0);
}
public TerminalNode FUN() { return getToken(KotlinParser.FUN, 0); }
public ClassDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_classDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterClassDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitClassDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitClassDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final ClassDeclarationContext classDeclaration() throws RecognitionException {
ClassDeclarationContext _localctx = new ClassDeclarationContext(_ctx, getState());
enterRule(_localctx, 22, RULE_classDeclaration);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(509);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AT_NO_WS || _la==AT_PRE_WS || ((((_la - 107)) & ~0x3f) == 0 && ((1L << (_la - 107)) & 234881023L) != 0)) {
{
setState(508);
modifiers();
}
}
setState(522);
_errHandler.sync(this);
switch (_input.LA(1)) {
case CLASS:
{
setState(511);
match(CLASS);
}
break;
case INTERFACE:
case FUN:
{
setState(519);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==FUN) {
{
setState(512);
match(FUN);
setState(516);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(513);
match(NL);
}
}
setState(518);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(521);
match(INTERFACE);
}
break;
default:
throw new NoViableAltException(this);
}
setState(527);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(524);
match(NL);
}
}
setState(529);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(530);
simpleIdentifier();
setState(538);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,33,_ctx) ) {
case 1:
{
setState(534);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(531);
match(NL);
}
}
setState(536);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(537);
typeParameters();
}
break;
}
setState(547);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,35,_ctx) ) {
case 1:
{
setState(543);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(540);
match(NL);
}
}
setState(545);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(546);
primaryConstructor();
}
break;
}
setState(563);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,38,_ctx) ) {
case 1:
{
setState(552);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(549);
match(NL);
}
}
setState(554);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(555);
match(COLON);
setState(559);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,37,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(556);
match(NL);
}
}
}
setState(561);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,37,_ctx);
}
setState(562);
delegationSpecifiers();
}
break;
}
setState(572);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,40,_ctx) ) {
case 1:
{
setState(568);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(565);
match(NL);
}
}
setState(570);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(571);
typeConstraints();
}
break;
}
setState(588);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,43,_ctx) ) {
case 1:
{
setState(577);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(574);
match(NL);
}
}
setState(579);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(580);
classBody();
}
break;
case 2:
{
setState(584);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(581);
match(NL);
}
}
setState(586);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(587);
enumClassBody();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PrimaryConstructorContext extends ParserRuleContext {
public ClassParametersContext classParameters() {
return getRuleContext(ClassParametersContext.class,0);
}
public TerminalNode CONSTRUCTOR() { return getToken(KotlinParser.CONSTRUCTOR, 0); }
public ModifiersContext modifiers() {
return getRuleContext(ModifiersContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public PrimaryConstructorContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_primaryConstructor; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterPrimaryConstructor(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitPrimaryConstructor(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitPrimaryConstructor(this);
else return visitor.visitChildren(this);
}
}
public final PrimaryConstructorContext primaryConstructor() throws RecognitionException {
PrimaryConstructorContext _localctx = new PrimaryConstructorContext(_ctx, getState());
enterRule(_localctx, 24, RULE_primaryConstructor);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(600);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AT_NO_WS || _la==AT_PRE_WS || ((((_la - 79)) & ~0x3f) == 0 && ((1L << (_la - 79)) & 63050394514751489L) != 0)) {
{
setState(591);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AT_NO_WS || _la==AT_PRE_WS || ((((_la - 107)) & ~0x3f) == 0 && ((1L << (_la - 107)) & 234881023L) != 0)) {
{
setState(590);
modifiers();
}
}
setState(593);
match(CONSTRUCTOR);
setState(597);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(594);
match(NL);
}
}
setState(599);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(602);
classParameters();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ClassBodyContext extends ParserRuleContext {
public TerminalNode LCURL() { return getToken(KotlinParser.LCURL, 0); }
public ClassMemberDeclarationsContext classMemberDeclarations() {
return getRuleContext(ClassMemberDeclarationsContext.class,0);
}
public TerminalNode RCURL() { return getToken(KotlinParser.RCURL, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public ClassBodyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_classBody; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterClassBody(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitClassBody(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitClassBody(this);
else return visitor.visitChildren(this);
}
}
public final ClassBodyContext classBody() throws RecognitionException {
ClassBodyContext _localctx = new ClassBodyContext(_ctx, getState());
enterRule(_localctx, 26, RULE_classBody);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(604);
match(LCURL);
setState(608);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,47,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(605);
match(NL);
}
}
}
setState(610);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,47,_ctx);
}
setState(611);
classMemberDeclarations();
setState(615);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(612);
match(NL);
}
}
setState(617);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(618);
match(RCURL);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ClassParametersContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(KotlinParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(KotlinParser.RPAREN, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public List classParameter() {
return getRuleContexts(ClassParameterContext.class);
}
public ClassParameterContext classParameter(int i) {
return getRuleContext(ClassParameterContext.class,i);
}
public List COMMA() { return getTokens(KotlinParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(KotlinParser.COMMA, i);
}
public ClassParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_classParameters; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterClassParameters(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitClassParameters(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitClassParameters(this);
else return visitor.visitChildren(this);
}
}
public final ClassParametersContext classParameters() throws RecognitionException {
ClassParametersContext _localctx = new ClassParametersContext(_ctx, getState());
enterRule(_localctx, 28, RULE_classParameters);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(620);
match(LPAREN);
setState(624);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,49,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(621);
match(NL);
}
}
}
setState(626);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,49,_ctx);
}
setState(656);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,55,_ctx) ) {
case 1:
{
setState(627);
classParameter();
setState(644);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,52,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(631);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(628);
match(NL);
}
}
setState(633);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(634);
match(COMMA);
setState(638);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,51,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(635);
match(NL);
}
}
}
setState(640);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,51,_ctx);
}
setState(641);
classParameter();
}
}
}
setState(646);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,52,_ctx);
}
setState(654);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,54,_ctx) ) {
case 1:
{
setState(650);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(647);
match(NL);
}
}
setState(652);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(653);
match(COMMA);
}
break;
}
}
break;
}
setState(661);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(658);
match(NL);
}
}
setState(663);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(664);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ClassParameterContext extends ParserRuleContext {
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public TerminalNode COLON() { return getToken(KotlinParser.COLON, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public ModifiersContext modifiers() {
return getRuleContext(ModifiersContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public TerminalNode ASSIGNMENT() { return getToken(KotlinParser.ASSIGNMENT, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode VAL() { return getToken(KotlinParser.VAL, 0); }
public TerminalNode VAR() { return getToken(KotlinParser.VAR, 0); }
public ClassParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_classParameter; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterClassParameter(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitClassParameter(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitClassParameter(this);
else return visitor.visitChildren(this);
}
}
public final ClassParameterContext classParameter() throws RecognitionException {
ClassParameterContext _localctx = new ClassParameterContext(_ctx, getState());
enterRule(_localctx, 30, RULE_classParameter);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(667);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,57,_ctx) ) {
case 1:
{
setState(666);
modifiers();
}
break;
}
setState(670);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==VAL || _la==VAR) {
{
setState(669);
_la = _input.LA(1);
if ( !(_la==VAL || _la==VAR) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(675);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(672);
match(NL);
}
}
setState(677);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(678);
simpleIdentifier();
setState(679);
match(COLON);
setState(683);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(680);
match(NL);
}
}
setState(685);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(686);
type();
setState(701);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,63,_ctx) ) {
case 1:
{
setState(690);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(687);
match(NL);
}
}
setState(692);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(693);
match(ASSIGNMENT);
setState(697);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(694);
match(NL);
}
}
setState(699);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(700);
expression();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DelegationSpecifiersContext extends ParserRuleContext {
public List annotatedDelegationSpecifier() {
return getRuleContexts(AnnotatedDelegationSpecifierContext.class);
}
public AnnotatedDelegationSpecifierContext annotatedDelegationSpecifier(int i) {
return getRuleContext(AnnotatedDelegationSpecifierContext.class,i);
}
public List COMMA() { return getTokens(KotlinParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(KotlinParser.COMMA, i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public DelegationSpecifiersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_delegationSpecifiers; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterDelegationSpecifiers(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitDelegationSpecifiers(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitDelegationSpecifiers(this);
else return visitor.visitChildren(this);
}
}
public final DelegationSpecifiersContext delegationSpecifiers() throws RecognitionException {
DelegationSpecifiersContext _localctx = new DelegationSpecifiersContext(_ctx, getState());
enterRule(_localctx, 32, RULE_delegationSpecifiers);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(703);
annotatedDelegationSpecifier();
setState(720);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,66,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(707);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(704);
match(NL);
}
}
setState(709);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(710);
match(COMMA);
setState(714);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,65,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(711);
match(NL);
}
}
}
setState(716);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,65,_ctx);
}
setState(717);
annotatedDelegationSpecifier();
}
}
}
setState(722);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,66,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DelegationSpecifierContext extends ParserRuleContext {
public ConstructorInvocationContext constructorInvocation() {
return getRuleContext(ConstructorInvocationContext.class,0);
}
public ExplicitDelegationContext explicitDelegation() {
return getRuleContext(ExplicitDelegationContext.class,0);
}
public UserTypeContext userType() {
return getRuleContext(UserTypeContext.class,0);
}
public FunctionTypeContext functionType() {
return getRuleContext(FunctionTypeContext.class,0);
}
public DelegationSpecifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_delegationSpecifier; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterDelegationSpecifier(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitDelegationSpecifier(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitDelegationSpecifier(this);
else return visitor.visitChildren(this);
}
}
public final DelegationSpecifierContext delegationSpecifier() throws RecognitionException {
DelegationSpecifierContext _localctx = new DelegationSpecifierContext(_ctx, getState());
enterRule(_localctx, 34, RULE_delegationSpecifier);
try {
setState(727);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,67,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(723);
constructorInvocation();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(724);
explicitDelegation();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(725);
userType();
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(726);
functionType();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ConstructorInvocationContext extends ParserRuleContext {
public UserTypeContext userType() {
return getRuleContext(UserTypeContext.class,0);
}
public ValueArgumentsContext valueArguments() {
return getRuleContext(ValueArgumentsContext.class,0);
}
public ConstructorInvocationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_constructorInvocation; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterConstructorInvocation(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitConstructorInvocation(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitConstructorInvocation(this);
else return visitor.visitChildren(this);
}
}
public final ConstructorInvocationContext constructorInvocation() throws RecognitionException {
ConstructorInvocationContext _localctx = new ConstructorInvocationContext(_ctx, getState());
enterRule(_localctx, 36, RULE_constructorInvocation);
try {
enterOuterAlt(_localctx, 1);
{
setState(729);
userType();
setState(730);
valueArguments();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AnnotatedDelegationSpecifierContext extends ParserRuleContext {
public DelegationSpecifierContext delegationSpecifier() {
return getRuleContext(DelegationSpecifierContext.class,0);
}
public List annotation() {
return getRuleContexts(AnnotationContext.class);
}
public AnnotationContext annotation(int i) {
return getRuleContext(AnnotationContext.class,i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public AnnotatedDelegationSpecifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_annotatedDelegationSpecifier; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterAnnotatedDelegationSpecifier(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitAnnotatedDelegationSpecifier(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitAnnotatedDelegationSpecifier(this);
else return visitor.visitChildren(this);
}
}
public final AnnotatedDelegationSpecifierContext annotatedDelegationSpecifier() throws RecognitionException {
AnnotatedDelegationSpecifierContext _localctx = new AnnotatedDelegationSpecifierContext(_ctx, getState());
enterRule(_localctx, 38, RULE_annotatedDelegationSpecifier);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(735);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,68,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(732);
annotation();
}
}
}
setState(737);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,68,_ctx);
}
setState(741);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(738);
match(NL);
}
}
setState(743);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(744);
delegationSpecifier();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExplicitDelegationContext extends ParserRuleContext {
public TerminalNode BY() { return getToken(KotlinParser.BY, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public UserTypeContext userType() {
return getRuleContext(UserTypeContext.class,0);
}
public FunctionTypeContext functionType() {
return getRuleContext(FunctionTypeContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public ExplicitDelegationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_explicitDelegation; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterExplicitDelegation(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitExplicitDelegation(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitExplicitDelegation(this);
else return visitor.visitChildren(this);
}
}
public final ExplicitDelegationContext explicitDelegation() throws RecognitionException {
ExplicitDelegationContext _localctx = new ExplicitDelegationContext(_ctx, getState());
enterRule(_localctx, 40, RULE_explicitDelegation);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(748);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,70,_ctx) ) {
case 1:
{
setState(746);
userType();
}
break;
case 2:
{
setState(747);
functionType();
}
break;
}
setState(753);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(750);
match(NL);
}
}
setState(755);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(756);
match(BY);
setState(760);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(757);
match(NL);
}
}
setState(762);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(763);
expression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeParametersContext extends ParserRuleContext {
public TerminalNode LANGLE() { return getToken(KotlinParser.LANGLE, 0); }
public List typeParameter() {
return getRuleContexts(TypeParameterContext.class);
}
public TypeParameterContext typeParameter(int i) {
return getRuleContext(TypeParameterContext.class,i);
}
public TerminalNode RANGLE() { return getToken(KotlinParser.RANGLE, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public List COMMA() { return getTokens(KotlinParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(KotlinParser.COMMA, i);
}
public TypeParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_typeParameters; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterTypeParameters(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitTypeParameters(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitTypeParameters(this);
else return visitor.visitChildren(this);
}
}
public final TypeParametersContext typeParameters() throws RecognitionException {
TypeParametersContext _localctx = new TypeParametersContext(_ctx, getState());
enterRule(_localctx, 42, RULE_typeParameters);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(765);
match(LANGLE);
setState(769);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,73,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(766);
match(NL);
}
}
}
setState(771);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,73,_ctx);
}
setState(772);
typeParameter();
setState(789);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,76,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(776);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(773);
match(NL);
}
}
setState(778);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(779);
match(COMMA);
setState(783);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,75,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(780);
match(NL);
}
}
}
setState(785);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,75,_ctx);
}
setState(786);
typeParameter();
}
}
}
setState(791);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,76,_ctx);
}
setState(799);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,78,_ctx) ) {
case 1:
{
setState(795);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(792);
match(NL);
}
}
setState(797);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(798);
match(COMMA);
}
break;
}
setState(804);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(801);
match(NL);
}
}
setState(806);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(807);
match(RANGLE);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeParameterContext extends ParserRuleContext {
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public TypeParameterModifiersContext typeParameterModifiers() {
return getRuleContext(TypeParameterModifiersContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public TerminalNode COLON() { return getToken(KotlinParser.COLON, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public TypeParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_typeParameter; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterTypeParameter(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitTypeParameter(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitTypeParameter(this);
else return visitor.visitChildren(this);
}
}
public final TypeParameterContext typeParameter() throws RecognitionException {
TypeParameterContext _localctx = new TypeParameterContext(_ctx, getState());
enterRule(_localctx, 44, RULE_typeParameter);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(810);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,80,_ctx) ) {
case 1:
{
setState(809);
typeParameterModifiers();
}
break;
}
setState(815);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(812);
match(NL);
}
}
setState(817);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(818);
simpleIdentifier();
setState(833);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,84,_ctx) ) {
case 1:
{
setState(822);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(819);
match(NL);
}
}
setState(824);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(825);
match(COLON);
setState(829);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(826);
match(NL);
}
}
setState(831);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(832);
type();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeConstraintsContext extends ParserRuleContext {
public TerminalNode WHERE() { return getToken(KotlinParser.WHERE, 0); }
public List typeConstraint() {
return getRuleContexts(TypeConstraintContext.class);
}
public TypeConstraintContext typeConstraint(int i) {
return getRuleContext(TypeConstraintContext.class,i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public List COMMA() { return getTokens(KotlinParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(KotlinParser.COMMA, i);
}
public TypeConstraintsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_typeConstraints; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterTypeConstraints(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitTypeConstraints(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitTypeConstraints(this);
else return visitor.visitChildren(this);
}
}
public final TypeConstraintsContext typeConstraints() throws RecognitionException {
TypeConstraintsContext _localctx = new TypeConstraintsContext(_ctx, getState());
enterRule(_localctx, 46, RULE_typeConstraints);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(835);
match(WHERE);
setState(839);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(836);
match(NL);
}
}
setState(841);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(842);
typeConstraint();
setState(859);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,88,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(846);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(843);
match(NL);
}
}
setState(848);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(849);
match(COMMA);
setState(853);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(850);
match(NL);
}
}
setState(855);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(856);
typeConstraint();
}
}
}
setState(861);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,88,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeConstraintContext extends ParserRuleContext {
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public TerminalNode COLON() { return getToken(KotlinParser.COLON, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public List annotation() {
return getRuleContexts(AnnotationContext.class);
}
public AnnotationContext annotation(int i) {
return getRuleContext(AnnotationContext.class,i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public TypeConstraintContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_typeConstraint; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterTypeConstraint(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitTypeConstraint(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitTypeConstraint(this);
else return visitor.visitChildren(this);
}
}
public final TypeConstraintContext typeConstraint() throws RecognitionException {
TypeConstraintContext _localctx = new TypeConstraintContext(_ctx, getState());
enterRule(_localctx, 48, RULE_typeConstraint);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(865);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==AT_NO_WS || _la==AT_PRE_WS) {
{
{
setState(862);
annotation();
}
}
setState(867);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(868);
simpleIdentifier();
setState(872);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(869);
match(NL);
}
}
setState(874);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(875);
match(COLON);
setState(879);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(876);
match(NL);
}
}
setState(881);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(882);
type();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ClassMemberDeclarationsContext extends ParserRuleContext {
public List classMemberDeclaration() {
return getRuleContexts(ClassMemberDeclarationContext.class);
}
public ClassMemberDeclarationContext classMemberDeclaration(int i) {
return getRuleContext(ClassMemberDeclarationContext.class,i);
}
public List semis() {
return getRuleContexts(SemisContext.class);
}
public SemisContext semis(int i) {
return getRuleContext(SemisContext.class,i);
}
public ClassMemberDeclarationsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_classMemberDeclarations; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterClassMemberDeclarations(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitClassMemberDeclarations(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitClassMemberDeclarations(this);
else return visitor.visitChildren(this);
}
}
public final ClassMemberDeclarationsContext classMemberDeclarations() throws RecognitionException {
ClassMemberDeclarationsContext _localctx = new ClassMemberDeclarationsContext(_ctx, getState());
enterRule(_localctx, 50, RULE_classMemberDeclarations);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(890);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==AT_NO_WS || _la==AT_PRE_WS || ((((_la - 72)) & ~0x3f) == 0 && ((1L << (_la - 72)) & 8070450497888192255L) != 0)) {
{
{
setState(884);
classMemberDeclaration();
setState(886);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,92,_ctx) ) {
case 1:
{
setState(885);
semis();
}
break;
}
}
}
setState(892);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ClassMemberDeclarationContext extends ParserRuleContext {
public DeclarationContext declaration() {
return getRuleContext(DeclarationContext.class,0);
}
public CompanionObjectContext companionObject() {
return getRuleContext(CompanionObjectContext.class,0);
}
public AnonymousInitializerContext anonymousInitializer() {
return getRuleContext(AnonymousInitializerContext.class,0);
}
public SecondaryConstructorContext secondaryConstructor() {
return getRuleContext(SecondaryConstructorContext.class,0);
}
public ClassMemberDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_classMemberDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterClassMemberDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitClassMemberDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitClassMemberDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final ClassMemberDeclarationContext classMemberDeclaration() throws RecognitionException {
ClassMemberDeclarationContext _localctx = new ClassMemberDeclarationContext(_ctx, getState());
enterRule(_localctx, 52, RULE_classMemberDeclaration);
try {
setState(897);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,94,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(893);
declaration();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(894);
companionObject();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(895);
anonymousInitializer();
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(896);
secondaryConstructor();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AnonymousInitializerContext extends ParserRuleContext {
public TerminalNode INIT() { return getToken(KotlinParser.INIT, 0); }
public BlockContext block() {
return getRuleContext(BlockContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public AnonymousInitializerContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_anonymousInitializer; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterAnonymousInitializer(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitAnonymousInitializer(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitAnonymousInitializer(this);
else return visitor.visitChildren(this);
}
}
public final AnonymousInitializerContext anonymousInitializer() throws RecognitionException {
AnonymousInitializerContext _localctx = new AnonymousInitializerContext(_ctx, getState());
enterRule(_localctx, 54, RULE_anonymousInitializer);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(899);
match(INIT);
setState(903);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(900);
match(NL);
}
}
setState(905);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(906);
block();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CompanionObjectContext extends ParserRuleContext {
public TerminalNode COMPANION() { return getToken(KotlinParser.COMPANION, 0); }
public TerminalNode OBJECT() { return getToken(KotlinParser.OBJECT, 0); }
public ModifiersContext modifiers() {
return getRuleContext(ModifiersContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public TerminalNode COLON() { return getToken(KotlinParser.COLON, 0); }
public DelegationSpecifiersContext delegationSpecifiers() {
return getRuleContext(DelegationSpecifiersContext.class,0);
}
public ClassBodyContext classBody() {
return getRuleContext(ClassBodyContext.class,0);
}
public CompanionObjectContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_companionObject; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterCompanionObject(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitCompanionObject(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitCompanionObject(this);
else return visitor.visitChildren(this);
}
}
public final CompanionObjectContext companionObject() throws RecognitionException {
CompanionObjectContext _localctx = new CompanionObjectContext(_ctx, getState());
enterRule(_localctx, 56, RULE_companionObject);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(909);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AT_NO_WS || _la==AT_PRE_WS || ((((_la - 107)) & ~0x3f) == 0 && ((1L << (_la - 107)) & 234881023L) != 0)) {
{
setState(908);
modifiers();
}
}
setState(911);
match(COMPANION);
setState(915);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(912);
match(NL);
}
}
setState(917);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(918);
match(OBJECT);
setState(926);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,99,_ctx) ) {
case 1:
{
setState(922);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(919);
match(NL);
}
}
setState(924);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(925);
simpleIdentifier();
}
break;
}
setState(942);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,102,_ctx) ) {
case 1:
{
setState(931);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(928);
match(NL);
}
}
setState(933);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(934);
match(COLON);
setState(938);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,101,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(935);
match(NL);
}
}
}
setState(940);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,101,_ctx);
}
setState(941);
delegationSpecifiers();
}
break;
}
setState(951);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,104,_ctx) ) {
case 1:
{
setState(947);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(944);
match(NL);
}
}
setState(949);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(950);
classBody();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FunctionValueParametersContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(KotlinParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(KotlinParser.RPAREN, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public List functionValueParameter() {
return getRuleContexts(FunctionValueParameterContext.class);
}
public FunctionValueParameterContext functionValueParameter(int i) {
return getRuleContext(FunctionValueParameterContext.class,i);
}
public List COMMA() { return getTokens(KotlinParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(KotlinParser.COMMA, i);
}
public FunctionValueParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_functionValueParameters; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterFunctionValueParameters(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitFunctionValueParameters(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitFunctionValueParameters(this);
else return visitor.visitChildren(this);
}
}
public final FunctionValueParametersContext functionValueParameters() throws RecognitionException {
FunctionValueParametersContext _localctx = new FunctionValueParametersContext(_ctx, getState());
enterRule(_localctx, 58, RULE_functionValueParameters);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(953);
match(LPAREN);
setState(957);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,105,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(954);
match(NL);
}
}
}
setState(959);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,105,_ctx);
}
setState(989);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 40)) & ~0x3f) == 0 && ((1L << (_la - 40)) & 6834017741570053L) != 0) || ((((_la - 105)) & ~0x3f) == 0 && ((1L << (_la - 105)) & 2200096997375L) != 0)) {
{
setState(960);
functionValueParameter();
setState(977);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,108,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(964);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(961);
match(NL);
}
}
setState(966);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(967);
match(COMMA);
setState(971);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(968);
match(NL);
}
}
setState(973);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(974);
functionValueParameter();
}
}
}
setState(979);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,108,_ctx);
}
setState(987);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,110,_ctx) ) {
case 1:
{
setState(983);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(980);
match(NL);
}
}
setState(985);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(986);
match(COMMA);
}
break;
}
}
}
setState(994);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(991);
match(NL);
}
}
setState(996);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(997);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FunctionValueParameterContext extends ParserRuleContext {
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class,0);
}
public ParameterModifiersContext parameterModifiers() {
return getRuleContext(ParameterModifiersContext.class,0);
}
public TerminalNode ASSIGNMENT() { return getToken(KotlinParser.ASSIGNMENT, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public FunctionValueParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_functionValueParameter; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterFunctionValueParameter(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitFunctionValueParameter(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitFunctionValueParameter(this);
else return visitor.visitChildren(this);
}
}
public final FunctionValueParameterContext functionValueParameter() throws RecognitionException {
FunctionValueParameterContext _localctx = new FunctionValueParameterContext(_ctx, getState());
enterRule(_localctx, 60, RULE_functionValueParameter);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1000);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,113,_ctx) ) {
case 1:
{
setState(999);
parameterModifiers();
}
break;
}
setState(1002);
parameter();
setState(1017);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,116,_ctx) ) {
case 1:
{
setState(1006);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1003);
match(NL);
}
}
setState(1008);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1009);
match(ASSIGNMENT);
setState(1013);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1010);
match(NL);
}
}
setState(1015);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1016);
expression();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FunctionDeclarationContext extends ParserRuleContext {
public TerminalNode FUN() { return getToken(KotlinParser.FUN, 0); }
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public FunctionValueParametersContext functionValueParameters() {
return getRuleContext(FunctionValueParametersContext.class,0);
}
public ModifiersContext modifiers() {
return getRuleContext(ModifiersContext.class,0);
}
public TypeParametersContext typeParameters() {
return getRuleContext(TypeParametersContext.class,0);
}
public ReceiverTypeContext receiverType() {
return getRuleContext(ReceiverTypeContext.class,0);
}
public TerminalNode DOT() { return getToken(KotlinParser.DOT, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public TerminalNode COLON() { return getToken(KotlinParser.COLON, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public TypeConstraintsContext typeConstraints() {
return getRuleContext(TypeConstraintsContext.class,0);
}
public FunctionBodyContext functionBody() {
return getRuleContext(FunctionBodyContext.class,0);
}
public FunctionDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_functionDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterFunctionDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitFunctionDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitFunctionDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final FunctionDeclarationContext functionDeclaration() throws RecognitionException {
FunctionDeclarationContext _localctx = new FunctionDeclarationContext(_ctx, getState());
enterRule(_localctx, 62, RULE_functionDeclaration);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1020);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AT_NO_WS || _la==AT_PRE_WS || ((((_la - 107)) & ~0x3f) == 0 && ((1L << (_la - 107)) & 234881023L) != 0)) {
{
setState(1019);
modifiers();
}
}
setState(1022);
match(FUN);
setState(1030);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,119,_ctx) ) {
case 1:
{
setState(1026);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1023);
match(NL);
}
}
setState(1028);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1029);
typeParameters();
}
break;
}
setState(1047);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,122,_ctx) ) {
case 1:
{
setState(1035);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1032);
match(NL);
}
}
setState(1037);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1038);
receiverType();
setState(1042);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1039);
match(NL);
}
}
setState(1044);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1045);
match(DOT);
}
break;
}
setState(1052);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1049);
match(NL);
}
}
setState(1054);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1055);
simpleIdentifier();
setState(1059);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1056);
match(NL);
}
}
setState(1061);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1062);
functionValueParameters();
setState(1077);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,127,_ctx) ) {
case 1:
{
setState(1066);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1063);
match(NL);
}
}
setState(1068);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1069);
match(COLON);
setState(1073);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1070);
match(NL);
}
}
setState(1075);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1076);
type();
}
break;
}
setState(1086);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,129,_ctx) ) {
case 1:
{
setState(1082);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1079);
match(NL);
}
}
setState(1084);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1085);
typeConstraints();
}
break;
}
setState(1095);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,131,_ctx) ) {
case 1:
{
setState(1091);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1088);
match(NL);
}
}
setState(1093);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1094);
functionBody();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FunctionBodyContext extends ParserRuleContext {
public BlockContext block() {
return getRuleContext(BlockContext.class,0);
}
public TerminalNode ASSIGNMENT() { return getToken(KotlinParser.ASSIGNMENT, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public FunctionBodyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_functionBody; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterFunctionBody(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitFunctionBody(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitFunctionBody(this);
else return visitor.visitChildren(this);
}
}
public final FunctionBodyContext functionBody() throws RecognitionException {
FunctionBodyContext _localctx = new FunctionBodyContext(_ctx, getState());
enterRule(_localctx, 64, RULE_functionBody);
int _la;
try {
setState(1106);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LCURL:
enterOuterAlt(_localctx, 1);
{
setState(1097);
block();
}
break;
case ASSIGNMENT:
enterOuterAlt(_localctx, 2);
{
setState(1098);
match(ASSIGNMENT);
setState(1102);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1099);
match(NL);
}
}
setState(1104);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1105);
expression();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class VariableDeclarationContext extends ParserRuleContext {
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public List annotation() {
return getRuleContexts(AnnotationContext.class);
}
public AnnotationContext annotation(int i) {
return getRuleContext(AnnotationContext.class,i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public TerminalNode COLON() { return getToken(KotlinParser.COLON, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public VariableDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_variableDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterVariableDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitVariableDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitVariableDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final VariableDeclarationContext variableDeclaration() throws RecognitionException {
VariableDeclarationContext _localctx = new VariableDeclarationContext(_ctx, getState());
enterRule(_localctx, 66, RULE_variableDeclaration);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1111);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==AT_NO_WS || _la==AT_PRE_WS) {
{
{
setState(1108);
annotation();
}
}
setState(1113);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1117);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1114);
match(NL);
}
}
setState(1119);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1120);
simpleIdentifier();
setState(1135);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,138,_ctx) ) {
case 1:
{
setState(1124);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1121);
match(NL);
}
}
setState(1126);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1127);
match(COLON);
setState(1131);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1128);
match(NL);
}
}
setState(1133);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1134);
type();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MultiVariableDeclarationContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(KotlinParser.LPAREN, 0); }
public List variableDeclaration() {
return getRuleContexts(VariableDeclarationContext.class);
}
public VariableDeclarationContext variableDeclaration(int i) {
return getRuleContext(VariableDeclarationContext.class,i);
}
public TerminalNode RPAREN() { return getToken(KotlinParser.RPAREN, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public List COMMA() { return getTokens(KotlinParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(KotlinParser.COMMA, i);
}
public MultiVariableDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_multiVariableDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterMultiVariableDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitMultiVariableDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitMultiVariableDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final MultiVariableDeclarationContext multiVariableDeclaration() throws RecognitionException {
MultiVariableDeclarationContext _localctx = new MultiVariableDeclarationContext(_ctx, getState());
enterRule(_localctx, 68, RULE_multiVariableDeclaration);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1137);
match(LPAREN);
setState(1141);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,139,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1138);
match(NL);
}
}
}
setState(1143);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,139,_ctx);
}
setState(1144);
variableDeclaration();
setState(1161);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,142,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1148);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1145);
match(NL);
}
}
setState(1150);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1151);
match(COMMA);
setState(1155);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,141,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1152);
match(NL);
}
}
}
setState(1157);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,141,_ctx);
}
setState(1158);
variableDeclaration();
}
}
}
setState(1163);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,142,_ctx);
}
setState(1171);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,144,_ctx) ) {
case 1:
{
setState(1167);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1164);
match(NL);
}
}
setState(1169);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1170);
match(COMMA);
}
break;
}
setState(1176);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1173);
match(NL);
}
}
setState(1178);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1179);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PropertyDeclarationContext extends ParserRuleContext {
public TerminalNode VAL() { return getToken(KotlinParser.VAL, 0); }
public TerminalNode VAR() { return getToken(KotlinParser.VAR, 0); }
public ModifiersContext modifiers() {
return getRuleContext(ModifiersContext.class,0);
}
public TypeParametersContext typeParameters() {
return getRuleContext(TypeParametersContext.class,0);
}
public ReceiverTypeContext receiverType() {
return getRuleContext(ReceiverTypeContext.class,0);
}
public TerminalNode DOT() { return getToken(KotlinParser.DOT, 0); }
public TypeConstraintsContext typeConstraints() {
return getRuleContext(TypeConstraintsContext.class,0);
}
public TerminalNode SEMICOLON() { return getToken(KotlinParser.SEMICOLON, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public MultiVariableDeclarationContext multiVariableDeclaration() {
return getRuleContext(MultiVariableDeclarationContext.class,0);
}
public VariableDeclarationContext variableDeclaration() {
return getRuleContext(VariableDeclarationContext.class,0);
}
public TerminalNode ASSIGNMENT() { return getToken(KotlinParser.ASSIGNMENT, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public PropertyDelegateContext propertyDelegate() {
return getRuleContext(PropertyDelegateContext.class,0);
}
public GetterContext getter() {
return getRuleContext(GetterContext.class,0);
}
public SetterContext setter() {
return getRuleContext(SetterContext.class,0);
}
public SemiContext semi() {
return getRuleContext(SemiContext.class,0);
}
public PropertyDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_propertyDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterPropertyDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitPropertyDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitPropertyDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final PropertyDeclarationContext propertyDeclaration() throws RecognitionException {
PropertyDeclarationContext _localctx = new PropertyDeclarationContext(_ctx, getState());
enterRule(_localctx, 70, RULE_propertyDeclaration);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1182);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AT_NO_WS || _la==AT_PRE_WS || ((((_la - 107)) & ~0x3f) == 0 && ((1L << (_la - 107)) & 234881023L) != 0)) {
{
setState(1181);
modifiers();
}
}
setState(1184);
_la = _input.LA(1);
if ( !(_la==VAL || _la==VAR) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1192);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,148,_ctx) ) {
case 1:
{
setState(1188);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1185);
match(NL);
}
}
setState(1190);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1191);
typeParameters();
}
break;
}
setState(1209);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,151,_ctx) ) {
case 1:
{
setState(1197);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1194);
match(NL);
}
}
setState(1199);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1200);
receiverType();
setState(1204);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1201);
match(NL);
}
}
setState(1206);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1207);
match(DOT);
}
break;
}
{
setState(1214);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,152,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1211);
match(NL);
}
}
}
setState(1216);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,152,_ctx);
}
setState(1219);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LPAREN:
{
setState(1217);
multiVariableDeclaration();
}
break;
case NL:
case AT_NO_WS:
case AT_PRE_WS:
case FILE:
case FIELD:
case PROPERTY:
case GET:
case SET:
case RECEIVER:
case PARAM:
case SETPARAM:
case DELEGATE:
case IMPORT:
case CONSTRUCTOR:
case BY:
case COMPANION:
case INIT:
case WHERE:
case CATCH:
case FINALLY:
case OUT:
case DYNAMIC:
case PUBLIC:
case PRIVATE:
case PROTECTED:
case INTERNAL:
case ENUM:
case SEALED:
case ANNOTATION:
case DATA:
case INNER:
case VALUE:
case TAILREC:
case OPERATOR:
case INLINE:
case INFIX:
case EXTERNAL:
case SUSPEND:
case OVERRIDE:
case ABSTRACT:
case FINAL:
case OPEN:
case CONST:
case LATEINIT:
case VARARG:
case NOINLINE:
case CROSSINLINE:
case REIFIED:
case EXPECT:
case ACTUAL:
case Identifier:
{
setState(1218);
variableDeclaration();
}
break;
default:
throw new NoViableAltException(this);
}
}
setState(1228);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,155,_ctx) ) {
case 1:
{
setState(1224);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1221);
match(NL);
}
}
setState(1226);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1227);
typeConstraints();
}
break;
}
setState(1247);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,159,_ctx) ) {
case 1:
{
setState(1233);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1230);
match(NL);
}
}
setState(1235);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1245);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ASSIGNMENT:
{
setState(1236);
match(ASSIGNMENT);
setState(1240);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1237);
match(NL);
}
}
setState(1242);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1243);
expression();
}
break;
case BY:
{
setState(1244);
propertyDelegate();
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
}
setState(1255);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,161,_ctx) ) {
case 1:
{
setState(1250);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(1249);
match(NL);
}
}
setState(1252);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==NL );
setState(1254);
match(SEMICOLON);
}
break;
}
setState(1260);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,162,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1257);
match(NL);
}
}
}
setState(1262);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,162,_ctx);
}
setState(1293);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,171,_ctx) ) {
case 1:
{
setState(1264);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,163,_ctx) ) {
case 1:
{
setState(1263);
getter();
}
break;
}
setState(1276);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,166,_ctx) ) {
case 1:
{
setState(1269);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,164,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1266);
match(NL);
}
}
}
setState(1271);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,164,_ctx);
}
setState(1273);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - -1)) & ~0x3f) == 0 && ((1L << (_la - -1)) & 268435521L) != 0)) {
{
setState(1272);
semi();
}
}
setState(1275);
setter();
}
break;
}
}
break;
case 2:
{
setState(1279);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,167,_ctx) ) {
case 1:
{
setState(1278);
setter();
}
break;
}
setState(1291);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,170,_ctx) ) {
case 1:
{
setState(1284);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,168,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1281);
match(NL);
}
}
}
setState(1286);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,168,_ctx);
}
setState(1288);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - -1)) & ~0x3f) == 0 && ((1L << (_la - -1)) & 268435521L) != 0)) {
{
setState(1287);
semi();
}
}
setState(1290);
getter();
}
break;
}
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PropertyDelegateContext extends ParserRuleContext {
public TerminalNode BY() { return getToken(KotlinParser.BY, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public PropertyDelegateContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_propertyDelegate; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterPropertyDelegate(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitPropertyDelegate(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitPropertyDelegate(this);
else return visitor.visitChildren(this);
}
}
public final PropertyDelegateContext propertyDelegate() throws RecognitionException {
PropertyDelegateContext _localctx = new PropertyDelegateContext(_ctx, getState());
enterRule(_localctx, 72, RULE_propertyDelegate);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1295);
match(BY);
setState(1299);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1296);
match(NL);
}
}
setState(1301);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1302);
expression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GetterContext extends ParserRuleContext {
public TerminalNode GET() { return getToken(KotlinParser.GET, 0); }
public ModifiersContext modifiers() {
return getRuleContext(ModifiersContext.class,0);
}
public TerminalNode LPAREN() { return getToken(KotlinParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(KotlinParser.RPAREN, 0); }
public FunctionBodyContext functionBody() {
return getRuleContext(FunctionBodyContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public TerminalNode COLON() { return getToken(KotlinParser.COLON, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public GetterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_getter; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterGetter(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitGetter(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitGetter(this);
else return visitor.visitChildren(this);
}
}
public final GetterContext getter() throws RecognitionException {
GetterContext _localctx = new GetterContext(_ctx, getState());
enterRule(_localctx, 74, RULE_getter);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1305);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AT_NO_WS || _la==AT_PRE_WS || ((((_la - 107)) & ~0x3f) == 0 && ((1L << (_la - 107)) & 234881023L) != 0)) {
{
setState(1304);
modifiers();
}
}
setState(1307);
match(GET);
setState(1345);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,180,_ctx) ) {
case 1:
{
setState(1311);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1308);
match(NL);
}
}
setState(1313);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1314);
match(LPAREN);
setState(1318);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1315);
match(NL);
}
}
setState(1320);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1321);
match(RPAREN);
setState(1336);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,178,_ctx) ) {
case 1:
{
setState(1325);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1322);
match(NL);
}
}
setState(1327);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1328);
match(COLON);
setState(1332);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1329);
match(NL);
}
}
setState(1334);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1335);
type();
}
break;
}
setState(1341);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1338);
match(NL);
}
}
setState(1343);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1344);
functionBody();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SetterContext extends ParserRuleContext {
public TerminalNode SET() { return getToken(KotlinParser.SET, 0); }
public ModifiersContext modifiers() {
return getRuleContext(ModifiersContext.class,0);
}
public TerminalNode LPAREN() { return getToken(KotlinParser.LPAREN, 0); }
public FunctionValueParameterWithOptionalTypeContext functionValueParameterWithOptionalType() {
return getRuleContext(FunctionValueParameterWithOptionalTypeContext.class,0);
}
public TerminalNode RPAREN() { return getToken(KotlinParser.RPAREN, 0); }
public FunctionBodyContext functionBody() {
return getRuleContext(FunctionBodyContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public TerminalNode COMMA() { return getToken(KotlinParser.COMMA, 0); }
public TerminalNode COLON() { return getToken(KotlinParser.COLON, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public SetterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_setter; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterSetter(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitSetter(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitSetter(this);
else return visitor.visitChildren(this);
}
}
public final SetterContext setter() throws RecognitionException {
SetterContext _localctx = new SetterContext(_ctx, getState());
enterRule(_localctx, 76, RULE_setter);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1348);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AT_NO_WS || _la==AT_PRE_WS || ((((_la - 107)) & ~0x3f) == 0 && ((1L << (_la - 107)) & 234881023L) != 0)) {
{
setState(1347);
modifiers();
}
}
setState(1350);
match(SET);
setState(1405);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,191,_ctx) ) {
case 1:
{
setState(1354);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1351);
match(NL);
}
}
setState(1356);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1357);
match(LPAREN);
setState(1361);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1358);
match(NL);
}
}
setState(1363);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1364);
functionValueParameterWithOptionalType();
setState(1372);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,185,_ctx) ) {
case 1:
{
setState(1368);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1365);
match(NL);
}
}
setState(1370);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1371);
match(COMMA);
}
break;
}
setState(1377);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1374);
match(NL);
}
}
setState(1379);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1380);
match(RPAREN);
setState(1395);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,189,_ctx) ) {
case 1:
{
setState(1384);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1381);
match(NL);
}
}
setState(1386);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1387);
match(COLON);
setState(1391);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1388);
match(NL);
}
}
setState(1393);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1394);
type();
}
break;
}
setState(1400);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1397);
match(NL);
}
}
setState(1402);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1403);
functionBody();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParametersWithOptionalTypeContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(KotlinParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(KotlinParser.RPAREN, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public List functionValueParameterWithOptionalType() {
return getRuleContexts(FunctionValueParameterWithOptionalTypeContext.class);
}
public FunctionValueParameterWithOptionalTypeContext functionValueParameterWithOptionalType(int i) {
return getRuleContext(FunctionValueParameterWithOptionalTypeContext.class,i);
}
public List COMMA() { return getTokens(KotlinParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(KotlinParser.COMMA, i);
}
public ParametersWithOptionalTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parametersWithOptionalType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterParametersWithOptionalType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitParametersWithOptionalType(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitParametersWithOptionalType(this);
else return visitor.visitChildren(this);
}
}
public final ParametersWithOptionalTypeContext parametersWithOptionalType() throws RecognitionException {
ParametersWithOptionalTypeContext _localctx = new ParametersWithOptionalTypeContext(_ctx, getState());
enterRule(_localctx, 78, RULE_parametersWithOptionalType);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1407);
match(LPAREN);
setState(1411);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,192,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1408);
match(NL);
}
}
}
setState(1413);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,192,_ctx);
}
setState(1443);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 40)) & ~0x3f) == 0 && ((1L << (_la - 40)) & 6834017741570053L) != 0) || ((((_la - 105)) & ~0x3f) == 0 && ((1L << (_la - 105)) & 2200096997375L) != 0)) {
{
setState(1414);
functionValueParameterWithOptionalType();
setState(1431);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,195,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1418);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1415);
match(NL);
}
}
setState(1420);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1421);
match(COMMA);
setState(1425);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1422);
match(NL);
}
}
setState(1427);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1428);
functionValueParameterWithOptionalType();
}
}
}
setState(1433);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,195,_ctx);
}
setState(1441);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,197,_ctx) ) {
case 1:
{
setState(1437);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1434);
match(NL);
}
}
setState(1439);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1440);
match(COMMA);
}
break;
}
}
}
setState(1448);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1445);
match(NL);
}
}
setState(1450);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1451);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FunctionValueParameterWithOptionalTypeContext extends ParserRuleContext {
public ParameterWithOptionalTypeContext parameterWithOptionalType() {
return getRuleContext(ParameterWithOptionalTypeContext.class,0);
}
public ParameterModifiersContext parameterModifiers() {
return getRuleContext(ParameterModifiersContext.class,0);
}
public TerminalNode ASSIGNMENT() { return getToken(KotlinParser.ASSIGNMENT, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public FunctionValueParameterWithOptionalTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_functionValueParameterWithOptionalType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterFunctionValueParameterWithOptionalType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitFunctionValueParameterWithOptionalType(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitFunctionValueParameterWithOptionalType(this);
else return visitor.visitChildren(this);
}
}
public final FunctionValueParameterWithOptionalTypeContext functionValueParameterWithOptionalType() throws RecognitionException {
FunctionValueParameterWithOptionalTypeContext _localctx = new FunctionValueParameterWithOptionalTypeContext(_ctx, getState());
enterRule(_localctx, 80, RULE_functionValueParameterWithOptionalType);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1454);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,200,_ctx) ) {
case 1:
{
setState(1453);
parameterModifiers();
}
break;
}
setState(1456);
parameterWithOptionalType();
setState(1471);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,203,_ctx) ) {
case 1:
{
setState(1460);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1457);
match(NL);
}
}
setState(1462);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1463);
match(ASSIGNMENT);
setState(1467);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1464);
match(NL);
}
}
setState(1469);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1470);
expression();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParameterWithOptionalTypeContext extends ParserRuleContext {
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public TerminalNode COLON() { return getToken(KotlinParser.COLON, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public ParameterWithOptionalTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parameterWithOptionalType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterParameterWithOptionalType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitParameterWithOptionalType(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitParameterWithOptionalType(this);
else return visitor.visitChildren(this);
}
}
public final ParameterWithOptionalTypeContext parameterWithOptionalType() throws RecognitionException {
ParameterWithOptionalTypeContext _localctx = new ParameterWithOptionalTypeContext(_ctx, getState());
enterRule(_localctx, 82, RULE_parameterWithOptionalType);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1473);
simpleIdentifier();
setState(1477);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,204,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1474);
match(NL);
}
}
}
setState(1479);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,204,_ctx);
}
setState(1488);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COLON) {
{
setState(1480);
match(COLON);
setState(1484);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1481);
match(NL);
}
}
setState(1486);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1487);
type();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParameterContext extends ParserRuleContext {
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public TerminalNode COLON() { return getToken(KotlinParser.COLON, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public ParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parameter; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterParameter(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitParameter(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitParameter(this);
else return visitor.visitChildren(this);
}
}
public final ParameterContext parameter() throws RecognitionException {
ParameterContext _localctx = new ParameterContext(_ctx, getState());
enterRule(_localctx, 84, RULE_parameter);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1490);
simpleIdentifier();
setState(1494);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1491);
match(NL);
}
}
setState(1496);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1497);
match(COLON);
setState(1501);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1498);
match(NL);
}
}
setState(1503);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1504);
type();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ObjectDeclarationContext extends ParserRuleContext {
public TerminalNode OBJECT() { return getToken(KotlinParser.OBJECT, 0); }
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public ModifiersContext modifiers() {
return getRuleContext(ModifiersContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public TerminalNode COLON() { return getToken(KotlinParser.COLON, 0); }
public DelegationSpecifiersContext delegationSpecifiers() {
return getRuleContext(DelegationSpecifiersContext.class,0);
}
public ClassBodyContext classBody() {
return getRuleContext(ClassBodyContext.class,0);
}
public ObjectDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_objectDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterObjectDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitObjectDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitObjectDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final ObjectDeclarationContext objectDeclaration() throws RecognitionException {
ObjectDeclarationContext _localctx = new ObjectDeclarationContext(_ctx, getState());
enterRule(_localctx, 86, RULE_objectDeclaration);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1507);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AT_NO_WS || _la==AT_PRE_WS || ((((_la - 107)) & ~0x3f) == 0 && ((1L << (_la - 107)) & 234881023L) != 0)) {
{
setState(1506);
modifiers();
}
}
setState(1509);
match(OBJECT);
setState(1513);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1510);
match(NL);
}
}
setState(1515);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1516);
simpleIdentifier();
setState(1531);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,213,_ctx) ) {
case 1:
{
setState(1520);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1517);
match(NL);
}
}
setState(1522);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1523);
match(COLON);
setState(1527);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,212,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1524);
match(NL);
}
}
}
setState(1529);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,212,_ctx);
}
setState(1530);
delegationSpecifiers();
}
break;
}
setState(1540);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,215,_ctx) ) {
case 1:
{
setState(1536);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1533);
match(NL);
}
}
setState(1538);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1539);
classBody();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SecondaryConstructorContext extends ParserRuleContext {
public TerminalNode CONSTRUCTOR() { return getToken(KotlinParser.CONSTRUCTOR, 0); }
public FunctionValueParametersContext functionValueParameters() {
return getRuleContext(FunctionValueParametersContext.class,0);
}
public ModifiersContext modifiers() {
return getRuleContext(ModifiersContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public TerminalNode COLON() { return getToken(KotlinParser.COLON, 0); }
public ConstructorDelegationCallContext constructorDelegationCall() {
return getRuleContext(ConstructorDelegationCallContext.class,0);
}
public BlockContext block() {
return getRuleContext(BlockContext.class,0);
}
public SecondaryConstructorContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_secondaryConstructor; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterSecondaryConstructor(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitSecondaryConstructor(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitSecondaryConstructor(this);
else return visitor.visitChildren(this);
}
}
public final SecondaryConstructorContext secondaryConstructor() throws RecognitionException {
SecondaryConstructorContext _localctx = new SecondaryConstructorContext(_ctx, getState());
enterRule(_localctx, 88, RULE_secondaryConstructor);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1543);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AT_NO_WS || _la==AT_PRE_WS || ((((_la - 107)) & ~0x3f) == 0 && ((1L << (_la - 107)) & 234881023L) != 0)) {
{
setState(1542);
modifiers();
}
}
setState(1545);
match(CONSTRUCTOR);
setState(1549);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1546);
match(NL);
}
}
setState(1551);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1552);
functionValueParameters();
setState(1567);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,220,_ctx) ) {
case 1:
{
setState(1556);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1553);
match(NL);
}
}
setState(1558);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1559);
match(COLON);
setState(1563);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1560);
match(NL);
}
}
setState(1565);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1566);
constructorDelegationCall();
}
break;
}
setState(1572);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,221,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1569);
match(NL);
}
}
}
setState(1574);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,221,_ctx);
}
setState(1576);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LCURL) {
{
setState(1575);
block();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ConstructorDelegationCallContext extends ParserRuleContext {
public ValueArgumentsContext valueArguments() {
return getRuleContext(ValueArgumentsContext.class,0);
}
public TerminalNode THIS() { return getToken(KotlinParser.THIS, 0); }
public TerminalNode SUPER() { return getToken(KotlinParser.SUPER, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public ConstructorDelegationCallContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_constructorDelegationCall; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterConstructorDelegationCall(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitConstructorDelegationCall(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitConstructorDelegationCall(this);
else return visitor.visitChildren(this);
}
}
public final ConstructorDelegationCallContext constructorDelegationCall() throws RecognitionException {
ConstructorDelegationCallContext _localctx = new ConstructorDelegationCallContext(_ctx, getState());
enterRule(_localctx, 90, RULE_constructorDelegationCall);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1578);
_la = _input.LA(1);
if ( !(_la==THIS || _la==SUPER) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1582);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1579);
match(NL);
}
}
setState(1584);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1585);
valueArguments();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class EnumClassBodyContext extends ParserRuleContext {
public TerminalNode LCURL() { return getToken(KotlinParser.LCURL, 0); }
public TerminalNode RCURL() { return getToken(KotlinParser.RCURL, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public EnumEntriesContext enumEntries() {
return getRuleContext(EnumEntriesContext.class,0);
}
public TerminalNode SEMICOLON() { return getToken(KotlinParser.SEMICOLON, 0); }
public ClassMemberDeclarationsContext classMemberDeclarations() {
return getRuleContext(ClassMemberDeclarationsContext.class,0);
}
public EnumClassBodyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_enumClassBody; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterEnumClassBody(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitEnumClassBody(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitEnumClassBody(this);
else return visitor.visitChildren(this);
}
}
public final EnumClassBodyContext enumClassBody() throws RecognitionException {
EnumClassBodyContext _localctx = new EnumClassBodyContext(_ctx, getState());
enterRule(_localctx, 92, RULE_enumClassBody);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1587);
match(LCURL);
setState(1591);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,224,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1588);
match(NL);
}
}
}
setState(1593);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,224,_ctx);
}
setState(1595);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 40)) & ~0x3f) == 0 && ((1L << (_la - 40)) & 6834017741570053L) != 0) || ((((_la - 105)) & ~0x3f) == 0 && ((1L << (_la - 105)) & 2200096997375L) != 0)) {
{
setState(1594);
enumEntries();
}
}
setState(1611);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,228,_ctx) ) {
case 1:
{
setState(1600);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1597);
match(NL);
}
}
setState(1602);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1603);
match(SEMICOLON);
setState(1607);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,227,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1604);
match(NL);
}
}
}
setState(1609);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,227,_ctx);
}
setState(1610);
classMemberDeclarations();
}
break;
}
setState(1616);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1613);
match(NL);
}
}
setState(1618);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1619);
match(RCURL);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class EnumEntriesContext extends ParserRuleContext {
public List enumEntry() {
return getRuleContexts(EnumEntryContext.class);
}
public EnumEntryContext enumEntry(int i) {
return getRuleContext(EnumEntryContext.class,i);
}
public List COMMA() { return getTokens(KotlinParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(KotlinParser.COMMA, i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public EnumEntriesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_enumEntries; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterEnumEntries(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitEnumEntries(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitEnumEntries(this);
else return visitor.visitChildren(this);
}
}
public final EnumEntriesContext enumEntries() throws RecognitionException {
EnumEntriesContext _localctx = new EnumEntriesContext(_ctx, getState());
enterRule(_localctx, 94, RULE_enumEntries);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1621);
enumEntry();
setState(1638);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,232,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1625);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1622);
match(NL);
}
}
setState(1627);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1628);
match(COMMA);
setState(1632);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1629);
match(NL);
}
}
setState(1634);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1635);
enumEntry();
}
}
}
setState(1640);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,232,_ctx);
}
setState(1644);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,233,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1641);
match(NL);
}
}
}
setState(1646);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,233,_ctx);
}
setState(1648);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA) {
{
setState(1647);
match(COMMA);
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class EnumEntryContext extends ParserRuleContext {
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public ModifiersContext modifiers() {
return getRuleContext(ModifiersContext.class,0);
}
public ValueArgumentsContext valueArguments() {
return getRuleContext(ValueArgumentsContext.class,0);
}
public ClassBodyContext classBody() {
return getRuleContext(ClassBodyContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public EnumEntryContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_enumEntry; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterEnumEntry(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitEnumEntry(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitEnumEntry(this);
else return visitor.visitChildren(this);
}
}
public final EnumEntryContext enumEntry() throws RecognitionException {
EnumEntryContext _localctx = new EnumEntryContext(_ctx, getState());
enterRule(_localctx, 96, RULE_enumEntry);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1657);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,236,_ctx) ) {
case 1:
{
setState(1650);
modifiers();
setState(1654);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1651);
match(NL);
}
}
setState(1656);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
break;
}
setState(1659);
simpleIdentifier();
setState(1667);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,238,_ctx) ) {
case 1:
{
setState(1663);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1660);
match(NL);
}
}
setState(1665);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1666);
valueArguments();
}
break;
}
setState(1676);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,240,_ctx) ) {
case 1:
{
setState(1672);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1669);
match(NL);
}
}
setState(1674);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1675);
classBody();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeContext extends ParserRuleContext {
public ParenthesizedTypeContext parenthesizedType() {
return getRuleContext(ParenthesizedTypeContext.class,0);
}
public NullableTypeContext nullableType() {
return getRuleContext(NullableTypeContext.class,0);
}
public TypeReferenceContext typeReference() {
return getRuleContext(TypeReferenceContext.class,0);
}
public FunctionTypeContext functionType() {
return getRuleContext(FunctionTypeContext.class,0);
}
public TypeModifiersContext typeModifiers() {
return getRuleContext(TypeModifiersContext.class,0);
}
public TypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_type; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitType(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitType(this);
else return visitor.visitChildren(this);
}
}
public final TypeContext type() throws RecognitionException {
TypeContext _localctx = new TypeContext(_ctx, getState());
enterRule(_localctx, 98, RULE_type);
try {
enterOuterAlt(_localctx, 1);
{
setState(1679);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,241,_ctx) ) {
case 1:
{
setState(1678);
typeModifiers();
}
break;
}
setState(1685);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,242,_ctx) ) {
case 1:
{
setState(1681);
parenthesizedType();
}
break;
case 2:
{
setState(1682);
nullableType();
}
break;
case 3:
{
setState(1683);
typeReference();
}
break;
case 4:
{
setState(1684);
functionType();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeReferenceContext extends ParserRuleContext {
public UserTypeContext userType() {
return getRuleContext(UserTypeContext.class,0);
}
public TerminalNode DYNAMIC() { return getToken(KotlinParser.DYNAMIC, 0); }
public TypeReferenceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_typeReference; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterTypeReference(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitTypeReference(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitTypeReference(this);
else return visitor.visitChildren(this);
}
}
public final TypeReferenceContext typeReference() throws RecognitionException {
TypeReferenceContext _localctx = new TypeReferenceContext(_ctx, getState());
enterRule(_localctx, 100, RULE_typeReference);
try {
setState(1689);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,243,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(1687);
userType();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(1688);
match(DYNAMIC);
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NullableTypeContext extends ParserRuleContext {
public TypeReferenceContext typeReference() {
return getRuleContext(TypeReferenceContext.class,0);
}
public ParenthesizedTypeContext parenthesizedType() {
return getRuleContext(ParenthesizedTypeContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public List quest() {
return getRuleContexts(QuestContext.class);
}
public QuestContext quest(int i) {
return getRuleContext(QuestContext.class,i);
}
public NullableTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_nullableType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterNullableType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitNullableType(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitNullableType(this);
else return visitor.visitChildren(this);
}
}
public final NullableTypeContext nullableType() throws RecognitionException {
NullableTypeContext _localctx = new NullableTypeContext(_ctx, getState());
enterRule(_localctx, 102, RULE_nullableType);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1693);
_errHandler.sync(this);
switch (_input.LA(1)) {
case FILE:
case FIELD:
case PROPERTY:
case GET:
case SET:
case RECEIVER:
case PARAM:
case SETPARAM:
case DELEGATE:
case IMPORT:
case CONSTRUCTOR:
case BY:
case COMPANION:
case INIT:
case WHERE:
case CATCH:
case FINALLY:
case OUT:
case DYNAMIC:
case PUBLIC:
case PRIVATE:
case PROTECTED:
case INTERNAL:
case ENUM:
case SEALED:
case ANNOTATION:
case DATA:
case INNER:
case VALUE:
case TAILREC:
case OPERATOR:
case INLINE:
case INFIX:
case EXTERNAL:
case SUSPEND:
case OVERRIDE:
case ABSTRACT:
case FINAL:
case OPEN:
case CONST:
case LATEINIT:
case VARARG:
case NOINLINE:
case CROSSINLINE:
case REIFIED:
case EXPECT:
case ACTUAL:
case Identifier:
{
setState(1691);
typeReference();
}
break;
case LPAREN:
{
setState(1692);
parenthesizedType();
}
break;
default:
throw new NoViableAltException(this);
}
setState(1698);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1695);
match(NL);
}
}
setState(1700);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1702);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(1701);
quest();
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(1704);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,246,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class QuestContext extends ParserRuleContext {
public TerminalNode QUEST_NO_WS() { return getToken(KotlinParser.QUEST_NO_WS, 0); }
public TerminalNode QUEST_WS() { return getToken(KotlinParser.QUEST_WS, 0); }
public QuestContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_quest; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterQuest(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitQuest(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitQuest(this);
else return visitor.visitChildren(this);
}
}
public final QuestContext quest() throws RecognitionException {
QuestContext _localctx = new QuestContext(_ctx, getState());
enterRule(_localctx, 104, RULE_quest);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1706);
_la = _input.LA(1);
if ( !(_la==QUEST_WS || _la==QUEST_NO_WS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UserTypeContext extends ParserRuleContext {
public List simpleUserType() {
return getRuleContexts(SimpleUserTypeContext.class);
}
public SimpleUserTypeContext simpleUserType(int i) {
return getRuleContext(SimpleUserTypeContext.class,i);
}
public List DOT() { return getTokens(KotlinParser.DOT); }
public TerminalNode DOT(int i) {
return getToken(KotlinParser.DOT, i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public UserTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_userType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterUserType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitUserType(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitUserType(this);
else return visitor.visitChildren(this);
}
}
public final UserTypeContext userType() throws RecognitionException {
UserTypeContext _localctx = new UserTypeContext(_ctx, getState());
enterRule(_localctx, 106, RULE_userType);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1708);
simpleUserType();
setState(1725);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,249,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1712);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1709);
match(NL);
}
}
setState(1714);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1715);
match(DOT);
setState(1719);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1716);
match(NL);
}
}
setState(1721);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1722);
simpleUserType();
}
}
}
setState(1727);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,249,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SimpleUserTypeContext extends ParserRuleContext {
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public TypeArgumentsContext typeArguments() {
return getRuleContext(TypeArgumentsContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public SimpleUserTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleUserType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterSimpleUserType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitSimpleUserType(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitSimpleUserType(this);
else return visitor.visitChildren(this);
}
}
public final SimpleUserTypeContext simpleUserType() throws RecognitionException {
SimpleUserTypeContext _localctx = new SimpleUserTypeContext(_ctx, getState());
enterRule(_localctx, 108, RULE_simpleUserType);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1728);
simpleIdentifier();
setState(1736);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,251,_ctx) ) {
case 1:
{
setState(1732);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1729);
match(NL);
}
}
setState(1734);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1735);
typeArguments();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeProjectionContext extends ParserRuleContext {
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public TypeProjectionModifiersContext typeProjectionModifiers() {
return getRuleContext(TypeProjectionModifiersContext.class,0);
}
public TerminalNode MULT() { return getToken(KotlinParser.MULT, 0); }
public TypeProjectionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_typeProjection; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterTypeProjection(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitTypeProjection(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitTypeProjection(this);
else return visitor.visitChildren(this);
}
}
public final TypeProjectionContext typeProjection() throws RecognitionException {
TypeProjectionContext _localctx = new TypeProjectionContext(_ctx, getState());
enterRule(_localctx, 110, RULE_typeProjection);
try {
setState(1743);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LPAREN:
case AT_NO_WS:
case AT_PRE_WS:
case FILE:
case FIELD:
case PROPERTY:
case GET:
case SET:
case RECEIVER:
case PARAM:
case SETPARAM:
case DELEGATE:
case IMPORT:
case CONSTRUCTOR:
case BY:
case COMPANION:
case INIT:
case WHERE:
case CATCH:
case FINALLY:
case IN:
case OUT:
case DYNAMIC:
case PUBLIC:
case PRIVATE:
case PROTECTED:
case INTERNAL:
case ENUM:
case SEALED:
case ANNOTATION:
case DATA:
case INNER:
case VALUE:
case TAILREC:
case OPERATOR:
case INLINE:
case INFIX:
case EXTERNAL:
case SUSPEND:
case OVERRIDE:
case ABSTRACT:
case FINAL:
case OPEN:
case CONST:
case LATEINIT:
case VARARG:
case NOINLINE:
case CROSSINLINE:
case REIFIED:
case EXPECT:
case ACTUAL:
case Identifier:
enterOuterAlt(_localctx, 1);
{
setState(1739);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,252,_ctx) ) {
case 1:
{
setState(1738);
typeProjectionModifiers();
}
break;
}
setState(1741);
type();
}
break;
case MULT:
enterOuterAlt(_localctx, 2);
{
setState(1742);
match(MULT);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeProjectionModifiersContext extends ParserRuleContext {
public List typeProjectionModifier() {
return getRuleContexts(TypeProjectionModifierContext.class);
}
public TypeProjectionModifierContext typeProjectionModifier(int i) {
return getRuleContext(TypeProjectionModifierContext.class,i);
}
public TypeProjectionModifiersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_typeProjectionModifiers; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterTypeProjectionModifiers(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitTypeProjectionModifiers(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitTypeProjectionModifiers(this);
else return visitor.visitChildren(this);
}
}
public final TypeProjectionModifiersContext typeProjectionModifiers() throws RecognitionException {
TypeProjectionModifiersContext _localctx = new TypeProjectionModifiersContext(_ctx, getState());
enterRule(_localctx, 112, RULE_typeProjectionModifiers);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1746);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(1745);
typeProjectionModifier();
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(1748);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,254,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeProjectionModifierContext extends ParserRuleContext {
public VarianceModifierContext varianceModifier() {
return getRuleContext(VarianceModifierContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public AnnotationContext annotation() {
return getRuleContext(AnnotationContext.class,0);
}
public TypeProjectionModifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_typeProjectionModifier; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterTypeProjectionModifier(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitTypeProjectionModifier(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitTypeProjectionModifier(this);
else return visitor.visitChildren(this);
}
}
public final TypeProjectionModifierContext typeProjectionModifier() throws RecognitionException {
TypeProjectionModifierContext _localctx = new TypeProjectionModifierContext(_ctx, getState());
enterRule(_localctx, 114, RULE_typeProjectionModifier);
int _la;
try {
setState(1758);
_errHandler.sync(this);
switch (_input.LA(1)) {
case IN:
case OUT:
enterOuterAlt(_localctx, 1);
{
setState(1750);
varianceModifier();
setState(1754);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1751);
match(NL);
}
}
setState(1756);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
break;
case AT_NO_WS:
case AT_PRE_WS:
enterOuterAlt(_localctx, 2);
{
setState(1757);
annotation();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FunctionTypeContext extends ParserRuleContext {
public FunctionTypeParametersContext functionTypeParameters() {
return getRuleContext(FunctionTypeParametersContext.class,0);
}
public TerminalNode ARROW() { return getToken(KotlinParser.ARROW, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public ReceiverTypeContext receiverType() {
return getRuleContext(ReceiverTypeContext.class,0);
}
public TerminalNode DOT() { return getToken(KotlinParser.DOT, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public FunctionTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_functionType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterFunctionType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitFunctionType(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitFunctionType(this);
else return visitor.visitChildren(this);
}
}
public final FunctionTypeContext functionType() throws RecognitionException {
FunctionTypeContext _localctx = new FunctionTypeContext(_ctx, getState());
enterRule(_localctx, 116, RULE_functionType);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1774);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,259,_ctx) ) {
case 1:
{
setState(1760);
receiverType();
setState(1764);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1761);
match(NL);
}
}
setState(1766);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1767);
match(DOT);
setState(1771);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1768);
match(NL);
}
}
setState(1773);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
break;
}
setState(1776);
functionTypeParameters();
setState(1780);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1777);
match(NL);
}
}
setState(1782);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1783);
match(ARROW);
setState(1787);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1784);
match(NL);
}
}
setState(1789);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1790);
type();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FunctionTypeParametersContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(KotlinParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(KotlinParser.RPAREN, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public List parameter() {
return getRuleContexts(ParameterContext.class);
}
public ParameterContext parameter(int i) {
return getRuleContext(ParameterContext.class,i);
}
public List type() {
return getRuleContexts(TypeContext.class);
}
public TypeContext type(int i) {
return getRuleContext(TypeContext.class,i);
}
public List COMMA() { return getTokens(KotlinParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(KotlinParser.COMMA, i);
}
public FunctionTypeParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_functionTypeParameters; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterFunctionTypeParameters(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitFunctionTypeParameters(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitFunctionTypeParameters(this);
else return visitor.visitChildren(this);
}
}
public final FunctionTypeParametersContext functionTypeParameters() throws RecognitionException {
FunctionTypeParametersContext _localctx = new FunctionTypeParametersContext(_ctx, getState());
enterRule(_localctx, 118, RULE_functionTypeParameters);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1792);
match(LPAREN);
setState(1796);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,262,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1793);
match(NL);
}
}
}
setState(1798);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,262,_ctx);
}
setState(1801);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,263,_ctx) ) {
case 1:
{
setState(1799);
parameter();
}
break;
case 2:
{
setState(1800);
type();
}
break;
}
setState(1822);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,267,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1806);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1803);
match(NL);
}
}
setState(1808);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1809);
match(COMMA);
setState(1813);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1810);
match(NL);
}
}
setState(1815);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1818);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,266,_ctx) ) {
case 1:
{
setState(1816);
parameter();
}
break;
case 2:
{
setState(1817);
type();
}
break;
}
}
}
}
setState(1824);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,267,_ctx);
}
setState(1832);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,269,_ctx) ) {
case 1:
{
setState(1828);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1825);
match(NL);
}
}
setState(1830);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1831);
match(COMMA);
}
break;
}
setState(1837);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1834);
match(NL);
}
}
setState(1839);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1840);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParenthesizedTypeContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(KotlinParser.LPAREN, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public TerminalNode RPAREN() { return getToken(KotlinParser.RPAREN, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public ParenthesizedTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parenthesizedType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterParenthesizedType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitParenthesizedType(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitParenthesizedType(this);
else return visitor.visitChildren(this);
}
}
public final ParenthesizedTypeContext parenthesizedType() throws RecognitionException {
ParenthesizedTypeContext _localctx = new ParenthesizedTypeContext(_ctx, getState());
enterRule(_localctx, 120, RULE_parenthesizedType);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1842);
match(LPAREN);
setState(1846);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1843);
match(NL);
}
}
setState(1848);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1849);
type();
setState(1853);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1850);
match(NL);
}
}
setState(1855);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1856);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReceiverTypeContext extends ParserRuleContext {
public ParenthesizedTypeContext parenthesizedType() {
return getRuleContext(ParenthesizedTypeContext.class,0);
}
public NullableTypeContext nullableType() {
return getRuleContext(NullableTypeContext.class,0);
}
public TypeReferenceContext typeReference() {
return getRuleContext(TypeReferenceContext.class,0);
}
public TypeModifiersContext typeModifiers() {
return getRuleContext(TypeModifiersContext.class,0);
}
public ReceiverTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_receiverType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterReceiverType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitReceiverType(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitReceiverType(this);
else return visitor.visitChildren(this);
}
}
public final ReceiverTypeContext receiverType() throws RecognitionException {
ReceiverTypeContext _localctx = new ReceiverTypeContext(_ctx, getState());
enterRule(_localctx, 122, RULE_receiverType);
try {
enterOuterAlt(_localctx, 1);
{
setState(1859);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,273,_ctx) ) {
case 1:
{
setState(1858);
typeModifiers();
}
break;
}
setState(1864);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,274,_ctx) ) {
case 1:
{
setState(1861);
parenthesizedType();
}
break;
case 2:
{
setState(1862);
nullableType();
}
break;
case 3:
{
setState(1863);
typeReference();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParenthesizedUserTypeContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(KotlinParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(KotlinParser.RPAREN, 0); }
public UserTypeContext userType() {
return getRuleContext(UserTypeContext.class,0);
}
public ParenthesizedUserTypeContext parenthesizedUserType() {
return getRuleContext(ParenthesizedUserTypeContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public ParenthesizedUserTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parenthesizedUserType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterParenthesizedUserType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitParenthesizedUserType(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitParenthesizedUserType(this);
else return visitor.visitChildren(this);
}
}
public final ParenthesizedUserTypeContext parenthesizedUserType() throws RecognitionException {
ParenthesizedUserTypeContext _localctx = new ParenthesizedUserTypeContext(_ctx, getState());
enterRule(_localctx, 124, RULE_parenthesizedUserType);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1866);
match(LPAREN);
setState(1870);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1867);
match(NL);
}
}
setState(1872);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1875);
_errHandler.sync(this);
switch (_input.LA(1)) {
case FILE:
case FIELD:
case PROPERTY:
case GET:
case SET:
case RECEIVER:
case PARAM:
case SETPARAM:
case DELEGATE:
case IMPORT:
case CONSTRUCTOR:
case BY:
case COMPANION:
case INIT:
case WHERE:
case CATCH:
case FINALLY:
case OUT:
case DYNAMIC:
case PUBLIC:
case PRIVATE:
case PROTECTED:
case INTERNAL:
case ENUM:
case SEALED:
case ANNOTATION:
case DATA:
case INNER:
case VALUE:
case TAILREC:
case OPERATOR:
case INLINE:
case INFIX:
case EXTERNAL:
case SUSPEND:
case OVERRIDE:
case ABSTRACT:
case FINAL:
case OPEN:
case CONST:
case LATEINIT:
case VARARG:
case NOINLINE:
case CROSSINLINE:
case REIFIED:
case EXPECT:
case ACTUAL:
case Identifier:
{
setState(1873);
userType();
}
break;
case LPAREN:
{
setState(1874);
parenthesizedUserType();
}
break;
default:
throw new NoViableAltException(this);
}
setState(1880);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1877);
match(NL);
}
}
setState(1882);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1883);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StatementsContext extends ParserRuleContext {
public List statement() {
return getRuleContexts(StatementContext.class);
}
public StatementContext statement(int i) {
return getRuleContext(StatementContext.class,i);
}
public SemisContext semis() {
return getRuleContext(SemisContext.class,0);
}
public List SEMICOLON() { return getTokens(KotlinParser.SEMICOLON); }
public TerminalNode SEMICOLON(int i) {
return getToken(KotlinParser.SEMICOLON, i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public StatementsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_statements; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterStatements(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitStatements(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitStatements(this);
else return visitor.visitChildren(this);
}
}
public final StatementsContext statements() throws RecognitionException {
StatementsContext _localctx = new StatementsContext(_ctx, getState());
enterRule(_localctx, 126, RULE_statements);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1900);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -72051958986561024L) != 0) || ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -2130322653249L) != 0) || ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & 6814975L) != 0)) {
{
setState(1885);
statement();
setState(1894);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,279,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1887);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(1886);
_la = _input.LA(1);
if ( !(_la==NL || _la==SEMICOLON) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(1889);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==NL || _la==SEMICOLON );
setState(1891);
statement();
}
}
}
setState(1896);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,279,_ctx);
}
setState(1898);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,280,_ctx) ) {
case 1:
{
setState(1897);
semis();
}
break;
}
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StatementContext extends ParserRuleContext {
public DeclarationContext declaration() {
return getRuleContext(DeclarationContext.class,0);
}
public AssignmentContext assignment() {
return getRuleContext(AssignmentContext.class,0);
}
public LoopStatementContext loopStatement() {
return getRuleContext(LoopStatementContext.class,0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public List label() {
return getRuleContexts(LabelContext.class);
}
public LabelContext label(int i) {
return getRuleContext(LabelContext.class,i);
}
public List annotation() {
return getRuleContexts(AnnotationContext.class);
}
public AnnotationContext annotation(int i) {
return getRuleContext(AnnotationContext.class,i);
}
public StatementContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_statement; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterStatement(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitStatement(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitStatement(this);
else return visitor.visitChildren(this);
}
}
public final StatementContext statement() throws RecognitionException {
StatementContext _localctx = new StatementContext(_ctx, getState());
enterRule(_localctx, 128, RULE_statement);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1906);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,283,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
setState(1904);
_errHandler.sync(this);
switch (_input.LA(1)) {
case FILE:
case FIELD:
case PROPERTY:
case GET:
case SET:
case RECEIVER:
case PARAM:
case SETPARAM:
case DELEGATE:
case IMPORT:
case CONSTRUCTOR:
case BY:
case COMPANION:
case INIT:
case WHERE:
case CATCH:
case FINALLY:
case OUT:
case DYNAMIC:
case PUBLIC:
case PRIVATE:
case PROTECTED:
case INTERNAL:
case ENUM:
case SEALED:
case ANNOTATION:
case DATA:
case INNER:
case VALUE:
case TAILREC:
case OPERATOR:
case INLINE:
case INFIX:
case EXTERNAL:
case SUSPEND:
case OVERRIDE:
case ABSTRACT:
case FINAL:
case OPEN:
case CONST:
case LATEINIT:
case VARARG:
case NOINLINE:
case CROSSINLINE:
case REIFIED:
case EXPECT:
case ACTUAL:
case Identifier:
{
setState(1902);
label();
}
break;
case AT_NO_WS:
case AT_PRE_WS:
{
setState(1903);
annotation();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
setState(1908);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,283,_ctx);
}
setState(1913);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,284,_ctx) ) {
case 1:
{
setState(1909);
declaration();
}
break;
case 2:
{
setState(1910);
assignment();
}
break;
case 3:
{
setState(1911);
loopStatement();
}
break;
case 4:
{
setState(1912);
expression();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelContext extends ParserRuleContext {
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public TerminalNode AT_NO_WS() { return getToken(KotlinParser.AT_NO_WS, 0); }
public TerminalNode AT_POST_WS() { return getToken(KotlinParser.AT_POST_WS, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public LabelContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_label; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterLabel(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitLabel(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitLabel(this);
else return visitor.visitChildren(this);
}
}
public final LabelContext label() throws RecognitionException {
LabelContext _localctx = new LabelContext(_ctx, getState());
enterRule(_localctx, 130, RULE_label);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1915);
simpleIdentifier();
setState(1916);
_la = _input.LA(1);
if ( !(_la==AT_NO_WS || _la==AT_POST_WS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1920);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,285,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1917);
match(NL);
}
}
}
setState(1922);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,285,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ControlStructureBodyContext extends ParserRuleContext {
public BlockContext block() {
return getRuleContext(BlockContext.class,0);
}
public StatementContext statement() {
return getRuleContext(StatementContext.class,0);
}
public ControlStructureBodyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_controlStructureBody; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterControlStructureBody(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitControlStructureBody(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitControlStructureBody(this);
else return visitor.visitChildren(this);
}
}
public final ControlStructureBodyContext controlStructureBody() throws RecognitionException {
ControlStructureBodyContext _localctx = new ControlStructureBodyContext(_ctx, getState());
enterRule(_localctx, 132, RULE_controlStructureBody);
try {
setState(1925);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,286,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(1923);
block();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(1924);
statement();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class BlockContext extends ParserRuleContext {
public TerminalNode LCURL() { return getToken(KotlinParser.LCURL, 0); }
public StatementsContext statements() {
return getRuleContext(StatementsContext.class,0);
}
public TerminalNode RCURL() { return getToken(KotlinParser.RCURL, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public BlockContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_block; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterBlock(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitBlock(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitBlock(this);
else return visitor.visitChildren(this);
}
}
public final BlockContext block() throws RecognitionException {
BlockContext _localctx = new BlockContext(_ctx, getState());
enterRule(_localctx, 134, RULE_block);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1927);
match(LCURL);
setState(1931);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,287,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1928);
match(NL);
}
}
}
setState(1933);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,287,_ctx);
}
setState(1934);
statements();
setState(1938);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1935);
match(NL);
}
}
setState(1940);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1941);
match(RCURL);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LoopStatementContext extends ParserRuleContext {
public ForStatementContext forStatement() {
return getRuleContext(ForStatementContext.class,0);
}
public WhileStatementContext whileStatement() {
return getRuleContext(WhileStatementContext.class,0);
}
public DoWhileStatementContext doWhileStatement() {
return getRuleContext(DoWhileStatementContext.class,0);
}
public LoopStatementContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_loopStatement; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterLoopStatement(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitLoopStatement(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitLoopStatement(this);
else return visitor.visitChildren(this);
}
}
public final LoopStatementContext loopStatement() throws RecognitionException {
LoopStatementContext _localctx = new LoopStatementContext(_ctx, getState());
enterRule(_localctx, 136, RULE_loopStatement);
try {
setState(1946);
_errHandler.sync(this);
switch (_input.LA(1)) {
case FOR:
enterOuterAlt(_localctx, 1);
{
setState(1943);
forStatement();
}
break;
case WHILE:
enterOuterAlt(_localctx, 2);
{
setState(1944);
whileStatement();
}
break;
case DO:
enterOuterAlt(_localctx, 3);
{
setState(1945);
doWhileStatement();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ForStatementContext extends ParserRuleContext {
public TerminalNode FOR() { return getToken(KotlinParser.FOR, 0); }
public TerminalNode LPAREN() { return getToken(KotlinParser.LPAREN, 0); }
public TerminalNode IN() { return getToken(KotlinParser.IN, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode RPAREN() { return getToken(KotlinParser.RPAREN, 0); }
public VariableDeclarationContext variableDeclaration() {
return getRuleContext(VariableDeclarationContext.class,0);
}
public MultiVariableDeclarationContext multiVariableDeclaration() {
return getRuleContext(MultiVariableDeclarationContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public List annotation() {
return getRuleContexts(AnnotationContext.class);
}
public AnnotationContext annotation(int i) {
return getRuleContext(AnnotationContext.class,i);
}
public ControlStructureBodyContext controlStructureBody() {
return getRuleContext(ControlStructureBodyContext.class,0);
}
public ForStatementContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_forStatement; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterForStatement(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitForStatement(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitForStatement(this);
else return visitor.visitChildren(this);
}
}
public final ForStatementContext forStatement() throws RecognitionException {
ForStatementContext _localctx = new ForStatementContext(_ctx, getState());
enterRule(_localctx, 138, RULE_forStatement);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1948);
match(FOR);
setState(1952);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1949);
match(NL);
}
}
setState(1954);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1955);
match(LPAREN);
setState(1959);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,291,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1956);
annotation();
}
}
}
setState(1961);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,291,_ctx);
}
setState(1964);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NL:
case AT_NO_WS:
case AT_PRE_WS:
case FILE:
case FIELD:
case PROPERTY:
case GET:
case SET:
case RECEIVER:
case PARAM:
case SETPARAM:
case DELEGATE:
case IMPORT:
case CONSTRUCTOR:
case BY:
case COMPANION:
case INIT:
case WHERE:
case CATCH:
case FINALLY:
case OUT:
case DYNAMIC:
case PUBLIC:
case PRIVATE:
case PROTECTED:
case INTERNAL:
case ENUM:
case SEALED:
case ANNOTATION:
case DATA:
case INNER:
case VALUE:
case TAILREC:
case OPERATOR:
case INLINE:
case INFIX:
case EXTERNAL:
case SUSPEND:
case OVERRIDE:
case ABSTRACT:
case FINAL:
case OPEN:
case CONST:
case LATEINIT:
case VARARG:
case NOINLINE:
case CROSSINLINE:
case REIFIED:
case EXPECT:
case ACTUAL:
case Identifier:
{
setState(1962);
variableDeclaration();
}
break;
case LPAREN:
{
setState(1963);
multiVariableDeclaration();
}
break;
default:
throw new NoViableAltException(this);
}
setState(1966);
match(IN);
setState(1967);
expression();
setState(1968);
match(RPAREN);
setState(1972);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,293,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1969);
match(NL);
}
}
}
setState(1974);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,293,_ctx);
}
setState(1976);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,294,_ctx) ) {
case 1:
{
setState(1975);
controlStructureBody();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class WhileStatementContext extends ParserRuleContext {
public TerminalNode WHILE() { return getToken(KotlinParser.WHILE, 0); }
public TerminalNode LPAREN() { return getToken(KotlinParser.LPAREN, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode RPAREN() { return getToken(KotlinParser.RPAREN, 0); }
public ControlStructureBodyContext controlStructureBody() {
return getRuleContext(ControlStructureBodyContext.class,0);
}
public TerminalNode SEMICOLON() { return getToken(KotlinParser.SEMICOLON, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public WhileStatementContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_whileStatement; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterWhileStatement(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitWhileStatement(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitWhileStatement(this);
else return visitor.visitChildren(this);
}
}
public final WhileStatementContext whileStatement() throws RecognitionException {
WhileStatementContext _localctx = new WhileStatementContext(_ctx, getState());
enterRule(_localctx, 140, RULE_whileStatement);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1978);
match(WHILE);
setState(1982);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1979);
match(NL);
}
}
setState(1984);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1985);
match(LPAREN);
setState(1986);
expression();
setState(1987);
match(RPAREN);
setState(1991);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(1988);
match(NL);
}
}
setState(1993);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1996);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LPAREN:
case LSQUARE:
case LCURL:
case ADD:
case SUB:
case INCR:
case DECR:
case EXCL_WS:
case EXCL_NO_WS:
case COLONCOLON:
case AT_NO_WS:
case AT_PRE_WS:
case RETURN_AT:
case CONTINUE_AT:
case BREAK_AT:
case THIS_AT:
case SUPER_AT:
case FILE:
case FIELD:
case PROPERTY:
case GET:
case SET:
case RECEIVER:
case PARAM:
case SETPARAM:
case DELEGATE:
case IMPORT:
case CLASS:
case INTERFACE:
case FUN:
case OBJECT:
case VAL:
case VAR:
case TYPE_ALIAS:
case CONSTRUCTOR:
case BY:
case COMPANION:
case INIT:
case THIS:
case SUPER:
case WHERE:
case IF:
case WHEN:
case TRY:
case CATCH:
case FINALLY:
case FOR:
case DO:
case WHILE:
case THROW:
case RETURN:
case CONTINUE:
case BREAK:
case OUT:
case DYNAMIC:
case PUBLIC:
case PRIVATE:
case PROTECTED:
case INTERNAL:
case ENUM:
case SEALED:
case ANNOTATION:
case DATA:
case INNER:
case VALUE:
case TAILREC:
case OPERATOR:
case INLINE:
case INFIX:
case EXTERNAL:
case SUSPEND:
case OVERRIDE:
case ABSTRACT:
case FINAL:
case OPEN:
case CONST:
case LATEINIT:
case VARARG:
case NOINLINE:
case CROSSINLINE:
case REIFIED:
case EXPECT:
case ACTUAL:
case RealLiteral:
case IntegerLiteral:
case HexLiteral:
case BinLiteral:
case UnsignedLiteral:
case LongLiteral:
case BooleanLiteral:
case NullLiteral:
case CharacterLiteral:
case Identifier:
case QUOTE_OPEN:
case TRIPLE_QUOTE_OPEN:
{
setState(1994);
controlStructureBody();
}
break;
case SEMICOLON:
{
setState(1995);
match(SEMICOLON);
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DoWhileStatementContext extends ParserRuleContext {
public TerminalNode DO() { return getToken(KotlinParser.DO, 0); }
public TerminalNode WHILE() { return getToken(KotlinParser.WHILE, 0); }
public TerminalNode LPAREN() { return getToken(KotlinParser.LPAREN, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode RPAREN() { return getToken(KotlinParser.RPAREN, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public ControlStructureBodyContext controlStructureBody() {
return getRuleContext(ControlStructureBodyContext.class,0);
}
public DoWhileStatementContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_doWhileStatement; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterDoWhileStatement(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitDoWhileStatement(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitDoWhileStatement(this);
else return visitor.visitChildren(this);
}
}
public final DoWhileStatementContext doWhileStatement() throws RecognitionException {
DoWhileStatementContext _localctx = new DoWhileStatementContext(_ctx, getState());
enterRule(_localctx, 142, RULE_doWhileStatement);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1998);
match(DO);
setState(2002);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,298,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1999);
match(NL);
}
}
}
setState(2004);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,298,_ctx);
}
setState(2006);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,299,_ctx) ) {
case 1:
{
setState(2005);
controlStructureBody();
}
break;
}
setState(2011);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2008);
match(NL);
}
}
setState(2013);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2014);
match(WHILE);
setState(2018);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2015);
match(NL);
}
}
setState(2020);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2021);
match(LPAREN);
setState(2022);
expression();
setState(2023);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AssignmentContext extends ParserRuleContext {
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public DirectlyAssignableExpressionContext directlyAssignableExpression() {
return getRuleContext(DirectlyAssignableExpressionContext.class,0);
}
public TerminalNode ASSIGNMENT() { return getToken(KotlinParser.ASSIGNMENT, 0); }
public AssignableExpressionContext assignableExpression() {
return getRuleContext(AssignableExpressionContext.class,0);
}
public AssignmentAndOperatorContext assignmentAndOperator() {
return getRuleContext(AssignmentAndOperatorContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public AssignmentContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_assignment; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterAssignment(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitAssignment(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitAssignment(this);
else return visitor.visitChildren(this);
}
}
public final AssignmentContext assignment() throws RecognitionException {
AssignmentContext _localctx = new AssignmentContext(_ctx, getState());
enterRule(_localctx, 144, RULE_assignment);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2031);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,302,_ctx) ) {
case 1:
{
setState(2025);
directlyAssignableExpression();
setState(2026);
match(ASSIGNMENT);
}
break;
case 2:
{
setState(2028);
assignableExpression();
setState(2029);
assignmentAndOperator();
}
break;
}
setState(2036);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2033);
match(NL);
}
}
setState(2038);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2039);
expression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SemiContext extends ParserRuleContext {
public TerminalNode SEMICOLON() { return getToken(KotlinParser.SEMICOLON, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public TerminalNode EOF() { return getToken(KotlinParser.EOF, 0); }
public SemiContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_semi; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterSemi(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitSemi(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitSemi(this);
else return visitor.visitChildren(this);
}
}
public final SemiContext semi() throws RecognitionException {
SemiContext _localctx = new SemiContext(_ctx, getState());
enterRule(_localctx, 146, RULE_semi);
int _la;
try {
int _alt;
setState(2049);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NL:
case SEMICOLON:
enterOuterAlt(_localctx, 1);
{
setState(2041);
_la = _input.LA(1);
if ( !(_la==NL || _la==SEMICOLON) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2045);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,304,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(2042);
match(NL);
}
}
}
setState(2047);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,304,_ctx);
}
}
break;
case EOF:
enterOuterAlt(_localctx, 2);
{
setState(2048);
match(EOF);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SemisContext extends ParserRuleContext {
public List SEMICOLON() { return getTokens(KotlinParser.SEMICOLON); }
public TerminalNode SEMICOLON(int i) {
return getToken(KotlinParser.SEMICOLON, i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public TerminalNode EOF() { return getToken(KotlinParser.EOF, 0); }
public SemisContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_semis; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterSemis(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitSemis(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitSemis(this);
else return visitor.visitChildren(this);
}
}
public final SemisContext semis() throws RecognitionException {
SemisContext _localctx = new SemisContext(_ctx, getState());
enterRule(_localctx, 148, RULE_semis);
int _la;
try {
int _alt;
setState(2057);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NL:
case SEMICOLON:
enterOuterAlt(_localctx, 1);
{
setState(2052);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(2051);
_la = _input.LA(1);
if ( !(_la==NL || _la==SEMICOLON) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(2054);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,306,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
}
break;
case EOF:
enterOuterAlt(_localctx, 2);
{
setState(2056);
match(EOF);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExpressionContext extends ParserRuleContext {
public DisjunctionContext disjunction() {
return getRuleContext(DisjunctionContext.class,0);
}
public ExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitExpression(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitExpression(this);
else return visitor.visitChildren(this);
}
}
public final ExpressionContext expression() throws RecognitionException {
ExpressionContext _localctx = new ExpressionContext(_ctx, getState());
enterRule(_localctx, 150, RULE_expression);
try {
enterOuterAlt(_localctx, 1);
{
setState(2059);
disjunction();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DisjunctionContext extends ParserRuleContext {
public List conjunction() {
return getRuleContexts(ConjunctionContext.class);
}
public ConjunctionContext conjunction(int i) {
return getRuleContext(ConjunctionContext.class,i);
}
public List DISJ() { return getTokens(KotlinParser.DISJ); }
public TerminalNode DISJ(int i) {
return getToken(KotlinParser.DISJ, i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public DisjunctionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_disjunction; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterDisjunction(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitDisjunction(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitDisjunction(this);
else return visitor.visitChildren(this);
}
}
public final DisjunctionContext disjunction() throws RecognitionException {
DisjunctionContext _localctx = new DisjunctionContext(_ctx, getState());
enterRule(_localctx, 152, RULE_disjunction);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2061);
conjunction();
setState(2078);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,310,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(2065);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2062);
match(NL);
}
}
setState(2067);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2068);
match(DISJ);
setState(2072);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2069);
match(NL);
}
}
setState(2074);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2075);
conjunction();
}
}
}
setState(2080);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,310,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ConjunctionContext extends ParserRuleContext {
public List equality() {
return getRuleContexts(EqualityContext.class);
}
public EqualityContext equality(int i) {
return getRuleContext(EqualityContext.class,i);
}
public List CONJ() { return getTokens(KotlinParser.CONJ); }
public TerminalNode CONJ(int i) {
return getToken(KotlinParser.CONJ, i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public ConjunctionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_conjunction; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterConjunction(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitConjunction(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitConjunction(this);
else return visitor.visitChildren(this);
}
}
public final ConjunctionContext conjunction() throws RecognitionException {
ConjunctionContext _localctx = new ConjunctionContext(_ctx, getState());
enterRule(_localctx, 154, RULE_conjunction);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2081);
equality();
setState(2098);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,313,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(2085);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2082);
match(NL);
}
}
setState(2087);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2088);
match(CONJ);
setState(2092);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2089);
match(NL);
}
}
setState(2094);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2095);
equality();
}
}
}
setState(2100);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,313,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class EqualityContext extends ParserRuleContext {
public List comparison() {
return getRuleContexts(ComparisonContext.class);
}
public ComparisonContext comparison(int i) {
return getRuleContext(ComparisonContext.class,i);
}
public List equalityOperator() {
return getRuleContexts(EqualityOperatorContext.class);
}
public EqualityOperatorContext equalityOperator(int i) {
return getRuleContext(EqualityOperatorContext.class,i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public EqualityContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_equality; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterEquality(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitEquality(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitEquality(this);
else return visitor.visitChildren(this);
}
}
public final EqualityContext equality() throws RecognitionException {
EqualityContext _localctx = new EqualityContext(_ctx, getState());
enterRule(_localctx, 156, RULE_equality);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2101);
comparison();
setState(2113);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,315,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(2102);
equalityOperator();
setState(2106);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2103);
match(NL);
}
}
setState(2108);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2109);
comparison();
}
}
}
setState(2115);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,315,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ComparisonContext extends ParserRuleContext {
public List genericCallLikeComparison() {
return getRuleContexts(GenericCallLikeComparisonContext.class);
}
public GenericCallLikeComparisonContext genericCallLikeComparison(int i) {
return getRuleContext(GenericCallLikeComparisonContext.class,i);
}
public List comparisonOperator() {
return getRuleContexts(ComparisonOperatorContext.class);
}
public ComparisonOperatorContext comparisonOperator(int i) {
return getRuleContext(ComparisonOperatorContext.class,i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public ComparisonContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_comparison; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterComparison(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitComparison(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitComparison(this);
else return visitor.visitChildren(this);
}
}
public final ComparisonContext comparison() throws RecognitionException {
ComparisonContext _localctx = new ComparisonContext(_ctx, getState());
enterRule(_localctx, 158, RULE_comparison);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2116);
genericCallLikeComparison();
setState(2128);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,317,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(2117);
comparisonOperator();
setState(2121);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2118);
match(NL);
}
}
setState(2123);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2124);
genericCallLikeComparison();
}
}
}
setState(2130);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,317,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GenericCallLikeComparisonContext extends ParserRuleContext {
public InfixOperationContext infixOperation() {
return getRuleContext(InfixOperationContext.class,0);
}
public List callSuffix() {
return getRuleContexts(CallSuffixContext.class);
}
public CallSuffixContext callSuffix(int i) {
return getRuleContext(CallSuffixContext.class,i);
}
public GenericCallLikeComparisonContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_genericCallLikeComparison; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterGenericCallLikeComparison(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitGenericCallLikeComparison(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitGenericCallLikeComparison(this);
else return visitor.visitChildren(this);
}
}
public final GenericCallLikeComparisonContext genericCallLikeComparison() throws RecognitionException {
GenericCallLikeComparisonContext _localctx = new GenericCallLikeComparisonContext(_ctx, getState());
enterRule(_localctx, 160, RULE_genericCallLikeComparison);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2131);
infixOperation();
setState(2135);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,318,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(2132);
callSuffix();
}
}
}
setState(2137);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,318,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InfixOperationContext extends ParserRuleContext {
public List elvisExpression() {
return getRuleContexts(ElvisExpressionContext.class);
}
public ElvisExpressionContext elvisExpression(int i) {
return getRuleContext(ElvisExpressionContext.class,i);
}
public List inOperator() {
return getRuleContexts(InOperatorContext.class);
}
public InOperatorContext inOperator(int i) {
return getRuleContext(InOperatorContext.class,i);
}
public List isOperator() {
return getRuleContexts(IsOperatorContext.class);
}
public IsOperatorContext isOperator(int i) {
return getRuleContext(IsOperatorContext.class,i);
}
public List type() {
return getRuleContexts(TypeContext.class);
}
public TypeContext type(int i) {
return getRuleContext(TypeContext.class,i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public InfixOperationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_infixOperation; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterInfixOperation(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitInfixOperation(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitInfixOperation(this);
else return visitor.visitChildren(this);
}
}
public final InfixOperationContext infixOperation() throws RecognitionException {
InfixOperationContext _localctx = new InfixOperationContext(_ctx, getState());
enterRule(_localctx, 162, RULE_infixOperation);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2138);
elvisExpression();
setState(2159);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,322,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
setState(2157);
_errHandler.sync(this);
switch (_input.LA(1)) {
case IN:
case NOT_IN:
{
setState(2139);
inOperator();
setState(2143);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2140);
match(NL);
}
}
setState(2145);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2146);
elvisExpression();
}
break;
case IS:
case NOT_IS:
{
setState(2148);
isOperator();
setState(2152);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2149);
match(NL);
}
}
setState(2154);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2155);
type();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
setState(2161);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,322,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ElvisExpressionContext extends ParserRuleContext {
public List infixFunctionCall() {
return getRuleContexts(InfixFunctionCallContext.class);
}
public InfixFunctionCallContext infixFunctionCall(int i) {
return getRuleContext(InfixFunctionCallContext.class,i);
}
public List elvis() {
return getRuleContexts(ElvisContext.class);
}
public ElvisContext elvis(int i) {
return getRuleContext(ElvisContext.class,i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public ElvisExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_elvisExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterElvisExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitElvisExpression(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitElvisExpression(this);
else return visitor.visitChildren(this);
}
}
public final ElvisExpressionContext elvisExpression() throws RecognitionException {
ElvisExpressionContext _localctx = new ElvisExpressionContext(_ctx, getState());
enterRule(_localctx, 164, RULE_elvisExpression);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2162);
infixFunctionCall();
setState(2180);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,325,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(2166);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2163);
match(NL);
}
}
setState(2168);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2169);
elvis();
setState(2173);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2170);
match(NL);
}
}
setState(2175);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2176);
infixFunctionCall();
}
}
}
setState(2182);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,325,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ElvisContext extends ParserRuleContext {
public TerminalNode QUEST_NO_WS() { return getToken(KotlinParser.QUEST_NO_WS, 0); }
public TerminalNode COLON() { return getToken(KotlinParser.COLON, 0); }
public ElvisContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_elvis; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterElvis(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitElvis(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitElvis(this);
else return visitor.visitChildren(this);
}
}
public final ElvisContext elvis() throws RecognitionException {
ElvisContext _localctx = new ElvisContext(_ctx, getState());
enterRule(_localctx, 166, RULE_elvis);
try {
enterOuterAlt(_localctx, 1);
{
setState(2183);
match(QUEST_NO_WS);
setState(2184);
match(COLON);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InfixFunctionCallContext extends ParserRuleContext {
public List rangeExpression() {
return getRuleContexts(RangeExpressionContext.class);
}
public RangeExpressionContext rangeExpression(int i) {
return getRuleContext(RangeExpressionContext.class,i);
}
public List simpleIdentifier() {
return getRuleContexts(SimpleIdentifierContext.class);
}
public SimpleIdentifierContext simpleIdentifier(int i) {
return getRuleContext(SimpleIdentifierContext.class,i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public InfixFunctionCallContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_infixFunctionCall; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterInfixFunctionCall(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitInfixFunctionCall(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitInfixFunctionCall(this);
else return visitor.visitChildren(this);
}
}
public final InfixFunctionCallContext infixFunctionCall() throws RecognitionException {
InfixFunctionCallContext _localctx = new InfixFunctionCallContext(_ctx, getState());
enterRule(_localctx, 168, RULE_infixFunctionCall);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2186);
rangeExpression();
setState(2198);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,327,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(2187);
simpleIdentifier();
setState(2191);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2188);
match(NL);
}
}
setState(2193);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2194);
rangeExpression();
}
}
}
setState(2200);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,327,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RangeExpressionContext extends ParserRuleContext {
public List additiveExpression() {
return getRuleContexts(AdditiveExpressionContext.class);
}
public AdditiveExpressionContext additiveExpression(int i) {
return getRuleContext(AdditiveExpressionContext.class,i);
}
public List RANGE() { return getTokens(KotlinParser.RANGE); }
public TerminalNode RANGE(int i) {
return getToken(KotlinParser.RANGE, i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public RangeExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_rangeExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterRangeExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitRangeExpression(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitRangeExpression(this);
else return visitor.visitChildren(this);
}
}
public final RangeExpressionContext rangeExpression() throws RecognitionException {
RangeExpressionContext _localctx = new RangeExpressionContext(_ctx, getState());
enterRule(_localctx, 170, RULE_rangeExpression);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2201);
additiveExpression();
setState(2212);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,329,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(2202);
match(RANGE);
setState(2206);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2203);
match(NL);
}
}
setState(2208);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2209);
additiveExpression();
}
}
}
setState(2214);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,329,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AdditiveExpressionContext extends ParserRuleContext {
public List multiplicativeExpression() {
return getRuleContexts(MultiplicativeExpressionContext.class);
}
public MultiplicativeExpressionContext multiplicativeExpression(int i) {
return getRuleContext(MultiplicativeExpressionContext.class,i);
}
public List additiveOperator() {
return getRuleContexts(AdditiveOperatorContext.class);
}
public AdditiveOperatorContext additiveOperator(int i) {
return getRuleContext(AdditiveOperatorContext.class,i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public AdditiveExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_additiveExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterAdditiveExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitAdditiveExpression(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitAdditiveExpression(this);
else return visitor.visitChildren(this);
}
}
public final AdditiveExpressionContext additiveExpression() throws RecognitionException {
AdditiveExpressionContext _localctx = new AdditiveExpressionContext(_ctx, getState());
enterRule(_localctx, 172, RULE_additiveExpression);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2215);
multiplicativeExpression();
setState(2227);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,331,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(2216);
additiveOperator();
setState(2220);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2217);
match(NL);
}
}
setState(2222);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2223);
multiplicativeExpression();
}
}
}
setState(2229);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,331,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MultiplicativeExpressionContext extends ParserRuleContext {
public List asExpression() {
return getRuleContexts(AsExpressionContext.class);
}
public AsExpressionContext asExpression(int i) {
return getRuleContext(AsExpressionContext.class,i);
}
public List multiplicativeOperator() {
return getRuleContexts(MultiplicativeOperatorContext.class);
}
public MultiplicativeOperatorContext multiplicativeOperator(int i) {
return getRuleContext(MultiplicativeOperatorContext.class,i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public MultiplicativeExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_multiplicativeExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterMultiplicativeExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitMultiplicativeExpression(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitMultiplicativeExpression(this);
else return visitor.visitChildren(this);
}
}
public final MultiplicativeExpressionContext multiplicativeExpression() throws RecognitionException {
MultiplicativeExpressionContext _localctx = new MultiplicativeExpressionContext(_ctx, getState());
enterRule(_localctx, 174, RULE_multiplicativeExpression);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2230);
asExpression();
setState(2242);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,333,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(2231);
multiplicativeOperator();
setState(2235);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2232);
match(NL);
}
}
setState(2237);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2238);
asExpression();
}
}
}
setState(2244);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,333,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AsExpressionContext extends ParserRuleContext {
public PrefixUnaryExpressionContext prefixUnaryExpression() {
return getRuleContext(PrefixUnaryExpressionContext.class,0);
}
public List asOperator() {
return getRuleContexts(AsOperatorContext.class);
}
public AsOperatorContext asOperator(int i) {
return getRuleContext(AsOperatorContext.class,i);
}
public List type() {
return getRuleContexts(TypeContext.class);
}
public TypeContext type(int i) {
return getRuleContext(TypeContext.class,i);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public AsExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_asExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterAsExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitAsExpression(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitAsExpression(this);
else return visitor.visitChildren(this);
}
}
public final AsExpressionContext asExpression() throws RecognitionException {
AsExpressionContext _localctx = new AsExpressionContext(_ctx, getState());
enterRule(_localctx, 176, RULE_asExpression);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2245);
prefixUnaryExpression();
setState(2263);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,336,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(2249);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2246);
match(NL);
}
}
setState(2251);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2252);
asOperator();
setState(2256);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2253);
match(NL);
}
}
setState(2258);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2259);
type();
}
}
}
setState(2265);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,336,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PrefixUnaryExpressionContext extends ParserRuleContext {
public PostfixUnaryExpressionContext postfixUnaryExpression() {
return getRuleContext(PostfixUnaryExpressionContext.class,0);
}
public List unaryPrefix() {
return getRuleContexts(UnaryPrefixContext.class);
}
public UnaryPrefixContext unaryPrefix(int i) {
return getRuleContext(UnaryPrefixContext.class,i);
}
public PrefixUnaryExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_prefixUnaryExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterPrefixUnaryExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitPrefixUnaryExpression(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitPrefixUnaryExpression(this);
else return visitor.visitChildren(this);
}
}
public final PrefixUnaryExpressionContext prefixUnaryExpression() throws RecognitionException {
PrefixUnaryExpressionContext _localctx = new PrefixUnaryExpressionContext(_ctx, getState());
enterRule(_localctx, 178, RULE_prefixUnaryExpression);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2269);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,337,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(2266);
unaryPrefix();
}
}
}
setState(2271);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,337,_ctx);
}
setState(2272);
postfixUnaryExpression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UnaryPrefixContext extends ParserRuleContext {
public AnnotationContext annotation() {
return getRuleContext(AnnotationContext.class,0);
}
public LabelContext label() {
return getRuleContext(LabelContext.class,0);
}
public PrefixUnaryOperatorContext prefixUnaryOperator() {
return getRuleContext(PrefixUnaryOperatorContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public UnaryPrefixContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_unaryPrefix; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterUnaryPrefix(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitUnaryPrefix(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitUnaryPrefix(this);
else return visitor.visitChildren(this);
}
}
public final UnaryPrefixContext unaryPrefix() throws RecognitionException {
UnaryPrefixContext _localctx = new UnaryPrefixContext(_ctx, getState());
enterRule(_localctx, 180, RULE_unaryPrefix);
int _la;
try {
setState(2283);
_errHandler.sync(this);
switch (_input.LA(1)) {
case AT_NO_WS:
case AT_PRE_WS:
enterOuterAlt(_localctx, 1);
{
setState(2274);
annotation();
}
break;
case FILE:
case FIELD:
case PROPERTY:
case GET:
case SET:
case RECEIVER:
case PARAM:
case SETPARAM:
case DELEGATE:
case IMPORT:
case CONSTRUCTOR:
case BY:
case COMPANION:
case INIT:
case WHERE:
case CATCH:
case FINALLY:
case OUT:
case DYNAMIC:
case PUBLIC:
case PRIVATE:
case PROTECTED:
case INTERNAL:
case ENUM:
case SEALED:
case ANNOTATION:
case DATA:
case INNER:
case VALUE:
case TAILREC:
case OPERATOR:
case INLINE:
case INFIX:
case EXTERNAL:
case SUSPEND:
case OVERRIDE:
case ABSTRACT:
case FINAL:
case OPEN:
case CONST:
case LATEINIT:
case VARARG:
case NOINLINE:
case CROSSINLINE:
case REIFIED:
case EXPECT:
case ACTUAL:
case Identifier:
enterOuterAlt(_localctx, 2);
{
setState(2275);
label();
}
break;
case ADD:
case SUB:
case INCR:
case DECR:
case EXCL_WS:
case EXCL_NO_WS:
enterOuterAlt(_localctx, 3);
{
setState(2276);
prefixUnaryOperator();
setState(2280);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2277);
match(NL);
}
}
setState(2282);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PostfixUnaryExpressionContext extends ParserRuleContext {
public PrimaryExpressionContext primaryExpression() {
return getRuleContext(PrimaryExpressionContext.class,0);
}
public List postfixUnarySuffix() {
return getRuleContexts(PostfixUnarySuffixContext.class);
}
public PostfixUnarySuffixContext postfixUnarySuffix(int i) {
return getRuleContext(PostfixUnarySuffixContext.class,i);
}
public PostfixUnaryExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_postfixUnaryExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterPostfixUnaryExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitPostfixUnaryExpression(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitPostfixUnaryExpression(this);
else return visitor.visitChildren(this);
}
}
public final PostfixUnaryExpressionContext postfixUnaryExpression() throws RecognitionException {
PostfixUnaryExpressionContext _localctx = new PostfixUnaryExpressionContext(_ctx, getState());
enterRule(_localctx, 182, RULE_postfixUnaryExpression);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2285);
primaryExpression();
setState(2289);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,340,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(2286);
postfixUnarySuffix();
}
}
}
setState(2291);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,340,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PostfixUnarySuffixContext extends ParserRuleContext {
public PostfixUnaryOperatorContext postfixUnaryOperator() {
return getRuleContext(PostfixUnaryOperatorContext.class,0);
}
public TypeArgumentsContext typeArguments() {
return getRuleContext(TypeArgumentsContext.class,0);
}
public CallSuffixContext callSuffix() {
return getRuleContext(CallSuffixContext.class,0);
}
public IndexingSuffixContext indexingSuffix() {
return getRuleContext(IndexingSuffixContext.class,0);
}
public NavigationSuffixContext navigationSuffix() {
return getRuleContext(NavigationSuffixContext.class,0);
}
public PostfixUnarySuffixContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_postfixUnarySuffix; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterPostfixUnarySuffix(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitPostfixUnarySuffix(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitPostfixUnarySuffix(this);
else return visitor.visitChildren(this);
}
}
public final PostfixUnarySuffixContext postfixUnarySuffix() throws RecognitionException {
PostfixUnarySuffixContext _localctx = new PostfixUnarySuffixContext(_ctx, getState());
enterRule(_localctx, 184, RULE_postfixUnarySuffix);
try {
setState(2297);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,341,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(2292);
postfixUnaryOperator();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(2293);
typeArguments();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(2294);
callSuffix();
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(2295);
indexingSuffix();
}
break;
case 5:
enterOuterAlt(_localctx, 5);
{
setState(2296);
navigationSuffix();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DirectlyAssignableExpressionContext extends ParserRuleContext {
public PostfixUnaryExpressionContext postfixUnaryExpression() {
return getRuleContext(PostfixUnaryExpressionContext.class,0);
}
public AssignableSuffixContext assignableSuffix() {
return getRuleContext(AssignableSuffixContext.class,0);
}
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public ParenthesizedDirectlyAssignableExpressionContext parenthesizedDirectlyAssignableExpression() {
return getRuleContext(ParenthesizedDirectlyAssignableExpressionContext.class,0);
}
public DirectlyAssignableExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_directlyAssignableExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterDirectlyAssignableExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitDirectlyAssignableExpression(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitDirectlyAssignableExpression(this);
else return visitor.visitChildren(this);
}
}
public final DirectlyAssignableExpressionContext directlyAssignableExpression() throws RecognitionException {
DirectlyAssignableExpressionContext _localctx = new DirectlyAssignableExpressionContext(_ctx, getState());
enterRule(_localctx, 186, RULE_directlyAssignableExpression);
try {
setState(2304);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,342,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(2299);
postfixUnaryExpression();
setState(2300);
assignableSuffix();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(2302);
simpleIdentifier();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(2303);
parenthesizedDirectlyAssignableExpression();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParenthesizedDirectlyAssignableExpressionContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(KotlinParser.LPAREN, 0); }
public DirectlyAssignableExpressionContext directlyAssignableExpression() {
return getRuleContext(DirectlyAssignableExpressionContext.class,0);
}
public TerminalNode RPAREN() { return getToken(KotlinParser.RPAREN, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public ParenthesizedDirectlyAssignableExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parenthesizedDirectlyAssignableExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterParenthesizedDirectlyAssignableExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitParenthesizedDirectlyAssignableExpression(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitParenthesizedDirectlyAssignableExpression(this);
else return visitor.visitChildren(this);
}
}
public final ParenthesizedDirectlyAssignableExpressionContext parenthesizedDirectlyAssignableExpression() throws RecognitionException {
ParenthesizedDirectlyAssignableExpressionContext _localctx = new ParenthesizedDirectlyAssignableExpressionContext(_ctx, getState());
enterRule(_localctx, 188, RULE_parenthesizedDirectlyAssignableExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2306);
match(LPAREN);
setState(2310);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2307);
match(NL);
}
}
setState(2312);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2313);
directlyAssignableExpression();
setState(2317);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2314);
match(NL);
}
}
setState(2319);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2320);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AssignableExpressionContext extends ParserRuleContext {
public PrefixUnaryExpressionContext prefixUnaryExpression() {
return getRuleContext(PrefixUnaryExpressionContext.class,0);
}
public ParenthesizedAssignableExpressionContext parenthesizedAssignableExpression() {
return getRuleContext(ParenthesizedAssignableExpressionContext.class,0);
}
public AssignableExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_assignableExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterAssignableExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitAssignableExpression(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitAssignableExpression(this);
else return visitor.visitChildren(this);
}
}
public final AssignableExpressionContext assignableExpression() throws RecognitionException {
AssignableExpressionContext _localctx = new AssignableExpressionContext(_ctx, getState());
enterRule(_localctx, 190, RULE_assignableExpression);
try {
setState(2324);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,345,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(2322);
prefixUnaryExpression();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(2323);
parenthesizedAssignableExpression();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParenthesizedAssignableExpressionContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(KotlinParser.LPAREN, 0); }
public AssignableExpressionContext assignableExpression() {
return getRuleContext(AssignableExpressionContext.class,0);
}
public TerminalNode RPAREN() { return getToken(KotlinParser.RPAREN, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public ParenthesizedAssignableExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parenthesizedAssignableExpression; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterParenthesizedAssignableExpression(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitParenthesizedAssignableExpression(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitParenthesizedAssignableExpression(this);
else return visitor.visitChildren(this);
}
}
public final ParenthesizedAssignableExpressionContext parenthesizedAssignableExpression() throws RecognitionException {
ParenthesizedAssignableExpressionContext _localctx = new ParenthesizedAssignableExpressionContext(_ctx, getState());
enterRule(_localctx, 192, RULE_parenthesizedAssignableExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2326);
match(LPAREN);
setState(2330);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2327);
match(NL);
}
}
setState(2332);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2333);
assignableExpression();
setState(2337);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2334);
match(NL);
}
}
setState(2339);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2340);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AssignableSuffixContext extends ParserRuleContext {
public TypeArgumentsContext typeArguments() {
return getRuleContext(TypeArgumentsContext.class,0);
}
public IndexingSuffixContext indexingSuffix() {
return getRuleContext(IndexingSuffixContext.class,0);
}
public NavigationSuffixContext navigationSuffix() {
return getRuleContext(NavigationSuffixContext.class,0);
}
public AssignableSuffixContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_assignableSuffix; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterAssignableSuffix(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitAssignableSuffix(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitAssignableSuffix(this);
else return visitor.visitChildren(this);
}
}
public final AssignableSuffixContext assignableSuffix() throws RecognitionException {
AssignableSuffixContext _localctx = new AssignableSuffixContext(_ctx, getState());
enterRule(_localctx, 194, RULE_assignableSuffix);
try {
setState(2345);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LANGLE:
enterOuterAlt(_localctx, 1);
{
setState(2342);
typeArguments();
}
break;
case LSQUARE:
enterOuterAlt(_localctx, 2);
{
setState(2343);
indexingSuffix();
}
break;
case NL:
case DOT:
case COLONCOLON:
case QUEST_NO_WS:
enterOuterAlt(_localctx, 3);
{
setState(2344);
navigationSuffix();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class IndexingSuffixContext extends ParserRuleContext {
public TerminalNode LSQUARE() { return getToken(KotlinParser.LSQUARE, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public TerminalNode RSQUARE() { return getToken(KotlinParser.RSQUARE, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public List COMMA() { return getTokens(KotlinParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(KotlinParser.COMMA, i);
}
public IndexingSuffixContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_indexingSuffix; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterIndexingSuffix(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitIndexingSuffix(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitIndexingSuffix(this);
else return visitor.visitChildren(this);
}
}
public final IndexingSuffixContext indexingSuffix() throws RecognitionException {
IndexingSuffixContext _localctx = new IndexingSuffixContext(_ctx, getState());
enterRule(_localctx, 196, RULE_indexingSuffix);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2347);
match(LSQUARE);
setState(2351);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2348);
match(NL);
}
}
setState(2353);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2354);
expression();
setState(2371);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,352,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(2358);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2355);
match(NL);
}
}
setState(2360);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2361);
match(COMMA);
setState(2365);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2362);
match(NL);
}
}
setState(2367);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2368);
expression();
}
}
}
setState(2373);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,352,_ctx);
}
setState(2381);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,354,_ctx) ) {
case 1:
{
setState(2377);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2374);
match(NL);
}
}
setState(2379);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2380);
match(COMMA);
}
break;
}
setState(2386);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2383);
match(NL);
}
}
setState(2388);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2389);
match(RSQUARE);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NavigationSuffixContext extends ParserRuleContext {
public MemberAccessOperatorContext memberAccessOperator() {
return getRuleContext(MemberAccessOperatorContext.class,0);
}
public SimpleIdentifierContext simpleIdentifier() {
return getRuleContext(SimpleIdentifierContext.class,0);
}
public ParenthesizedExpressionContext parenthesizedExpression() {
return getRuleContext(ParenthesizedExpressionContext.class,0);
}
public TerminalNode CLASS() { return getToken(KotlinParser.CLASS, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public NavigationSuffixContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_navigationSuffix; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterNavigationSuffix(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitNavigationSuffix(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitNavigationSuffix(this);
else return visitor.visitChildren(this);
}
}
public final NavigationSuffixContext navigationSuffix() throws RecognitionException {
NavigationSuffixContext _localctx = new NavigationSuffixContext(_ctx, getState());
enterRule(_localctx, 198, RULE_navigationSuffix);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2391);
memberAccessOperator();
setState(2395);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2392);
match(NL);
}
}
setState(2397);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2401);
_errHandler.sync(this);
switch (_input.LA(1)) {
case FILE:
case FIELD:
case PROPERTY:
case GET:
case SET:
case RECEIVER:
case PARAM:
case SETPARAM:
case DELEGATE:
case IMPORT:
case CONSTRUCTOR:
case BY:
case COMPANION:
case INIT:
case WHERE:
case CATCH:
case FINALLY:
case OUT:
case DYNAMIC:
case PUBLIC:
case PRIVATE:
case PROTECTED:
case INTERNAL:
case ENUM:
case SEALED:
case ANNOTATION:
case DATA:
case INNER:
case VALUE:
case TAILREC:
case OPERATOR:
case INLINE:
case INFIX:
case EXTERNAL:
case SUSPEND:
case OVERRIDE:
case ABSTRACT:
case FINAL:
case OPEN:
case CONST:
case LATEINIT:
case VARARG:
case NOINLINE:
case CROSSINLINE:
case REIFIED:
case EXPECT:
case ACTUAL:
case Identifier:
{
setState(2398);
simpleIdentifier();
}
break;
case LPAREN:
{
setState(2399);
parenthesizedExpression();
}
break;
case CLASS:
{
setState(2400);
match(CLASS);
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CallSuffixContext extends ParserRuleContext {
public AnnotatedLambdaContext annotatedLambda() {
return getRuleContext(AnnotatedLambdaContext.class,0);
}
public ValueArgumentsContext valueArguments() {
return getRuleContext(ValueArgumentsContext.class,0);
}
public TypeArgumentsContext typeArguments() {
return getRuleContext(TypeArgumentsContext.class,0);
}
public CallSuffixContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_callSuffix; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterCallSuffix(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitCallSuffix(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitCallSuffix(this);
else return visitor.visitChildren(this);
}
}
public final CallSuffixContext callSuffix() throws RecognitionException {
CallSuffixContext _localctx = new CallSuffixContext(_ctx, getState());
enterRule(_localctx, 200, RULE_callSuffix);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2404);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LANGLE) {
{
setState(2403);
typeArguments();
}
}
setState(2411);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,360,_ctx) ) {
case 1:
{
setState(2407);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LPAREN) {
{
setState(2406);
valueArguments();
}
}
setState(2409);
annotatedLambda();
}
break;
case 2:
{
setState(2410);
valueArguments();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AnnotatedLambdaContext extends ParserRuleContext {
public LambdaLiteralContext lambdaLiteral() {
return getRuleContext(LambdaLiteralContext.class,0);
}
public List annotation() {
return getRuleContexts(AnnotationContext.class);
}
public AnnotationContext annotation(int i) {
return getRuleContext(AnnotationContext.class,i);
}
public LabelContext label() {
return getRuleContext(LabelContext.class,0);
}
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public AnnotatedLambdaContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_annotatedLambda; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterAnnotatedLambda(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitAnnotatedLambda(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof KotlinParserVisitor ) return ((KotlinParserVisitor extends T>)visitor).visitAnnotatedLambda(this);
else return visitor.visitChildren(this);
}
}
public final AnnotatedLambdaContext annotatedLambda() throws RecognitionException {
AnnotatedLambdaContext _localctx = new AnnotatedLambdaContext(_ctx, getState());
enterRule(_localctx, 202, RULE_annotatedLambda);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2416);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==AT_NO_WS || _la==AT_PRE_WS) {
{
{
setState(2413);
annotation();
}
}
setState(2418);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2420);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 61)) & ~0x3f) == 0 && ((1L << (_la - 61)) & -17588927330817L) != 0) || ((((_la - 125)) & ~0x3f) == 0 && ((1L << (_la - 125)) & 2098175L) != 0)) {
{
setState(2419);
label();
}
}
setState(2425);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NL) {
{
{
setState(2422);
match(NL);
}
}
setState(2427);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2428);
lambdaLiteral();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeArgumentsContext extends ParserRuleContext {
public TerminalNode LANGLE() { return getToken(KotlinParser.LANGLE, 0); }
public List typeProjection() {
return getRuleContexts(TypeProjectionContext.class);
}
public TypeProjectionContext typeProjection(int i) {
return getRuleContext(TypeProjectionContext.class,i);
}
public TerminalNode RANGLE() { return getToken(KotlinParser.RANGLE, 0); }
public List NL() { return getTokens(KotlinParser.NL); }
public TerminalNode NL(int i) {
return getToken(KotlinParser.NL, i);
}
public List COMMA() { return getTokens(KotlinParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(KotlinParser.COMMA, i);
}
public TypeArgumentsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_typeArguments; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).enterTypeArguments(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof KotlinParserListener ) ((KotlinParserListener)listener).exitTypeArguments(this);
}
@Override
public