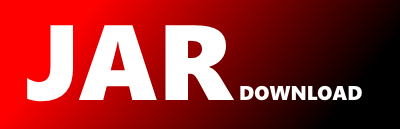
com.pi4j.util.ExecUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pi4j-core Show documentation
Show all versions of pi4j-core Show documentation
Pi4J Java API & Runtime Library
package com.pi4j.util;
/*
* #%L
* **********************************************************************
* ORGANIZATION : Pi4J
* PROJECT : Pi4J :: Java Library (Core)
* FILENAME : ExecUtil.java
*
* This file is part of the Pi4J project. More information about
* this project can be found here: http://www.pi4j.com/
* **********************************************************************
* %%
* Copyright (C) 2012 - 2015 Pi4J
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.List;
public class ExecUtil
{
public static String[] execute(String command) throws IOException, InterruptedException {
return execute(command, null);
}
public static String[] execute(String command, String split) throws IOException, InterruptedException {
List result = new ArrayList<>();
Process p = Runtime.getRuntime().exec(command);
p.waitFor();
if(p.exitValue() != 0)
return null;
InputStreamReader isr = new InputStreamReader(p.getInputStream());
BufferedReader reader = new BufferedReader(isr);
String line = reader.readLine();
while (line != null) {
if (!line.isEmpty()) {
if (split == null || split.isEmpty()) {
result.add(line.trim());
} else {
String[] parts = line.trim().split(split);
for(String part : parts) {
if (part != null && !part.isEmpty()) {
result.add(part.trim());
}
}
}
}
line = reader.readLine();
}
// close readers and stream
reader.close();
isr.close();
p.getInputStream().close();
if (result.size() > 0) {
return result.toArray(new String[result.size()]);
} else {
return new String[0];
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy