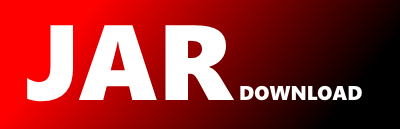
com.pi4j.util.Console Maven / Gradle / Ivy
package com.pi4j.util;
/*
* #%L
* **********************************************************************
* ORGANIZATION : Pi4J
* PROJECT : Pi4J :: LIBRARY :: Java Library (CORE)
* FILENAME : Console.java
*
* This file is part of the Pi4J project. More information about
* this project can be found here: https://pi4j.com/
* **********************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Console class.
*
* @author Robert Savage (http://www.savagehomeautomation.com)
* @version $Id: $Id
*/
public class Console {
private static final Logger logger = LoggerFactory.getLogger(Console.class);
private static final int LINE_WIDTH = 60;
/** Constant CLEAR_SCREEN_ESCAPE_SEQUENCE="\033[2J\033[1;1H"
*/
public static final String CLEAR_SCREEN_ESCAPE_SEQUENCE = "\033[2J\033[1;1H";
/** Constant ERASE_LINE_ESCAPE_SEQUENCE="\033[K"
*/
public static final String ERASE_LINE_ESCAPE_SEQUENCE = "\033[K";
/** Constant LINE_SEPARATOR_CHAR='*'
*/
public static final char LINE_SEPARATOR_CHAR = '*';
/** Constant LINE_SEPARATOR="StringUtil.repeat(LINE_SEPARATOR_CHAR, "{trunked}
*/
public static final String LINE_SEPARATOR = StringUtil.repeat(LINE_SEPARATOR_CHAR, LINE_WIDTH);
protected boolean exiting = false;
/**
* println.
*
* @param format a {@link java.lang.String} object.
* @param args a {@link java.lang.Object} object.
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console println(String format, Object ... args){
return println(String.format(format, args));
}
/**
* print.
*
* @param format a {@link java.lang.String} object.
* @param args a {@link java.lang.Object} object.
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console print(String format, Object ... args){
return print(String.format(format, args));
}
/**
* println.
*
* @param line a {@link java.lang.String} object.
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console println(String line){
logger.info(line);
return this;
}
/**
* println.
*
* @param line a {@link java.lang.Object} object.
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console println(Object line){
logger.info(line.toString());
return this;
}
/**
* println.
*
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console println(){
return println("");
}
/**
* print.
*
* @param data a {@link java.lang.Object} object.
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console print(Object data){
logger.info(data.toString());
return this;
}
/**
* print.
*
* @param data a {@link java.lang.String} object.
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console print(String data){
logger.info(data);
return this;
}
/**
* println.
*
* @param character a char.
* @param repeat a int.
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console println(char character, int repeat){
return println(StringUtil.repeat(character, repeat));
}
/**
* emptyLine.
*
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console emptyLine(){
return emptyLine(1);
}
/**
* emptyLine.
*
* @param number a int.
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console emptyLine(int number){
for(var index = 0; index < number; index++){
println();
}
return this;
}
/**
* separatorLine.
*
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console separatorLine(){
return println(LINE_SEPARATOR);
}
/**
* separatorLine.
*
* @param character a char.
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console separatorLine(char character){
return separatorLine(character, LINE_WIDTH);
}
/**
* separatorLine.
*
* @param character a char.
* @param length a int.
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console separatorLine(char character, int length){
return println(StringUtil.repeat(character, length));
}
/**
* title.
*
* @param title a {@link java.lang.String} object.
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console title(String ... title){
clearScreen().separatorLine().separatorLine().emptyLine();
for(var s : title) {
println(StringUtil.center(s, LINE_WIDTH));
}
emptyLine().separatorLine().separatorLine().emptyLine();
return this;
}
/**
* box.
*
* @param lines a {@link java.lang.String} object.
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console box(String ... lines) {
return box(2, lines);
}
/**
* box.
*
* @param padding a int.
* @param lines a {@link java.lang.String} object.
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console box(int padding, String ... lines) {
int max_length = 0;
for(var l : lines) {
if (l.length() > max_length) {
max_length = l.length();
}
}
separatorLine('-', max_length + padding * 2 + 2);
var left = StringUtil.padRight("|", padding);
var right = StringUtil.padLeft("|", padding);
for(var l : lines){
println(StringUtil.concat(left, StringUtil.padRight(l, max_length - l.length()), right));
}
separatorLine('-', max_length + padding * 2 + 2);
return this;
}
/**
* goodbye.
*
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console goodbye() {
emptyLine();
separatorLine();
println(StringUtil.center("GOODBYE", LINE_WIDTH));
separatorLine();
emptyLine();
return this;
}
/**
* clearScreen.
*
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console clearScreen(){
return print(CLEAR_SCREEN_ESCAPE_SEQUENCE);
}
/**
* eraseLine.
*
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console eraseLine(){
return print(ERASE_LINE_ESCAPE_SEQUENCE);
}
/**
* promptForExit.
*
* @return a {@link com.pi4j.util.Console} object.
*/
public synchronized Console promptForExit(){
box(4, "PRESS CTRL-C TO EXIT");
emptyLine();
exiting = false;
Runtime.getRuntime().addShutdownHook(new Thread() {
@Override
public void run() {
exiting = true;
goodbye();
}
});
return this;
}
/**
* waitForExit.
*
* @throws java.lang.InterruptedException if any.
*/
public void waitForExit() throws InterruptedException {
while(!exiting){
Thread.sleep(50);
}
}
/**
* exiting.
*
* @return a boolean.
*/
public synchronized boolean exiting(){
return exiting;
}
/**
* isRunning.
*
* @return a boolean.
*/
public synchronized boolean isRunning(){
return !exiting;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy