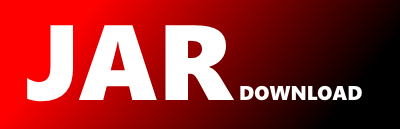
com.pi4j.platform.Platforms Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pi4j-core Show documentation
Show all versions of pi4j-core Show documentation
Pi4J Java API & Runtime Library
The newest version!
package com.pi4j.platform;
/*
* #%L
* **********************************************************************
* ORGANIZATION : Pi4J
* PROJECT : Pi4J :: LIBRARY :: Java Library (CORE)
* FILENAME : Platforms.java
*
* This file is part of the Pi4J project. More information about
* this project can be found here: https://pi4j.com/
* **********************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import com.pi4j.common.Describable;
import com.pi4j.common.Descriptor;
import com.pi4j.platform.exception.PlatformException;
import com.pi4j.platform.exception.PlatformNotFoundException;
import com.pi4j.platform.exception.PlatformTypeException;
import java.util.Collections;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* Platforms
*
* @see http://www.pi4j.com/
* @author Robert Savage (http://www.savagehomeautomation.com)
* @version $Id: $Id
*/
public interface Platforms extends Describable {
/**
* all.
*
* @return a {@link java.util.Map} object.
*/
Map all();
/**
* exists.
*
* @param platformId a {@link java.lang.String} object.
* @return a boolean.
*/
boolean exists(String platformId);
/**
* get.
*
* @param platformId a {@link java.lang.String} object.
* @return a {@link com.pi4j.platform.Platform} object.
* @throws com.pi4j.platform.exception.PlatformNotFoundException if any.
*/
Platform get(String platformId) throws PlatformNotFoundException;
/**
* defaultPlatform.
*
* @param a T object.
* @return a T object.
*/
T defaultPlatform();
// DEFAULT METHODS
/**
* exists.
*
* @param platformId a {@link java.lang.String} object.
* @param platformClass a {@link java.lang.Class} object.
* @param a T object.
* @return a boolean.
* @throws com.pi4j.platform.exception.PlatformNotFoundException if any.
*/
default boolean exists(String platformId, Class platformClass) throws PlatformNotFoundException {
// determine if the requested platform exists by ID and CLASS TYPE
try {
return get(platformId, platformClass) != null;
} catch (PlatformException e) {
return false;
}
}
/**
* hasDefault.
*
* @return a boolean.
*/
default boolean hasDefault(){
return defaultPlatform() != null;
}
/**
* getDefault.
*
* @param a T object.
* @return a T object.
*/
default T getDefault(){
return defaultPlatform();
}
/**
* get.
*
* @param platformId a {@link java.lang.String} object.
* @param platformClass a {@link java.lang.Class} object.
* @param a T object.
* @return a T object.
* @throws com.pi4j.platform.exception.PlatformNotFoundException if any.
* @throws com.pi4j.platform.exception.PlatformTypeException if any.
*/
default T get(String platformId, Class platformClass) throws PlatformNotFoundException, PlatformTypeException {
// return the platform instance from the managed platform map that contains the given platform-id and platform-class
var platform = get(platformId);
if(platformClass == null) {
return (T) platform;
}
if (platformClass.isAssignableFrom(platform.getClass())) {
return (T) platform;
}
throw new PlatformTypeException(platformId, platformClass);
}
default boolean exists(Class extends Platform> platformClass) throws PlatformNotFoundException {
// determine if the requested platform exists by ID and CLASS TYPE
try {
return get(platformClass) != null;
} catch (PlatformException e) {
return false;
}
}
/**
* get.
*
* @param platformClass a {@link java.lang.Class} object.
* @param a T object.
* @return a T object.
* @throws com.pi4j.platform.exception.PlatformNotFoundException if any.
*/
default T get(Class platformClass) throws PlatformNotFoundException{
// return the platform instance from the managed platform map that contains the given platform-id and platform-class
var subset = all(platformClass);
if(subset.isEmpty()){
throw new PlatformNotFoundException(platformClass);
}
// return first instance found
return (T)subset.values().iterator().next();
}
/**
* Get all platforms of a specified io class/interface.
*
* @param platformClass a {@link java.lang.Class} object.
* @param platforms objects extending the {@link com.pi4j.platform.Platform} interface
* @return a {@link java.util.Map} object.
* @throws com.pi4j.platform.exception.PlatformNotFoundException if any.
*/
default Map all(Class platformClass) throws PlatformNotFoundException {
// create a map of platforms that extend of the given platform class/interface
var result = new ConcurrentHashMap();
all().values().stream().filter(platformClass::isInstance).forEach(p -> {
result.put(p.id(), platformClass.cast(p));
});
if(result.size() <= 0) throw new PlatformNotFoundException(platformClass);
return Collections.unmodifiableMap(result);
}
/**
* getAll.
*
* @return a {@link java.util.Map} object.
*/
default Map getAll() { return all(); }
/**
* getAll.
*
* @param platformClass a {@link java.lang.Class} object.
* @param a T object.
* @return a {@link java.util.Map} object.
* @throws com.pi4j.platform.exception.PlatformNotFoundException if any.
*/
default Map getAll(Class platformClass) throws PlatformNotFoundException {
return all(platformClass);
}
/**
* describe.
*
* @return a {@link com.pi4j.common.Descriptor} object.
*/
default Descriptor describe() {
var platforms = all();
Descriptor descriptor = Descriptor.create()
.category("PLATFORMS")
.name("Pi4J Runtime Platforms")
.quantity((platforms == null) ? 0 : platforms.size())
.type(this.getClass());
if(platforms != null && !platforms.isEmpty()) {
platforms.forEach((id, platform) -> {
descriptor.add(platform.describe());
});
}
return descriptor;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy